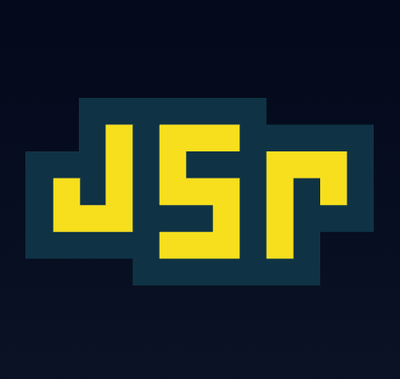
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
electron-builder
Advanced tools
A complete solution to package and build a ready for distribution Electron app for MacOS, Windows and Linux with “auto update” support out of the box
electron-builder is a complete solution to package and build a ready-for-distribution Electron app with “auto update” support out of the box. It supports numerous target formats and platforms, making it a versatile tool for Electron app developers.
Building for Multiple Platforms
This feature allows you to build your Electron app for multiple platforms such as Windows, macOS, and Linux. The code sample demonstrates how to configure and initiate a build process for a Windows target.
const builder = require('electron-builder');
builder.build({
targets: builder.Platform.WINDOWS.createTarget(),
config: {
appId: 'com.example.app',
productName: 'ExampleApp',
directories: {
output: 'dist'
}
}
}).then(() => {
console.log('Build complete!');
}).catch((error) => {
console.error('Error during build:', error);
});
Auto Update
The auto-update feature allows your app to automatically check for updates and install them. The code sample shows how to set up the auto-updater to check for updates when the app is ready and handle update events.
const { autoUpdater } = require('electron-updater');
app.on('ready', () => {
autoUpdater.checkForUpdatesAndNotify();
});
autoUpdater.on('update-available', () => {
console.log('Update available.');
});
autoUpdater.on('update-downloaded', () => {
autoUpdater.quitAndInstall();
});
Custom Configuration
This feature allows you to customize the build configuration for your Electron app. The code sample demonstrates how to specify custom directories, files, and target formats for different platforms.
const builder = require('electron-builder');
builder.build({
config: {
appId: 'com.example.app',
productName: 'ExampleApp',
directories: {
output: 'dist'
},
files: [
'build/**/*'
],
extraResources: [
'assets/'
],
win: {
target: 'nsis'
},
mac: {
target: 'dmg'
}
}
}).then(() => {
console.log('Build complete!');
}).catch((error) => {
console.error('Error during build:', error);
});
electron-packager is a command-line tool and Node.js library that bundles Electron-based application source code with a renamed Electron executable and supporting files into folders ready for distribution. Unlike electron-builder, it does not include auto-update support out of the box and is generally considered less feature-rich.
electron-forge is a complete tool for creating, publishing, and installing modern Electron applications. It provides a more integrated development experience compared to electron-builder, including project scaffolding, packaging, and publishing. However, it may not offer the same level of customization and flexibility in build configurations.
electron-updater is a standalone module that provides auto-update functionality for Electron apps. While electron-builder includes auto-update support as part of its feature set, electron-updater can be used independently or in conjunction with other packaging tools like electron-packager.
A complete solution to package and build a ready for distribution Electron app for macOS, Windows and Linux with “auto update” support out of the box.
7z
, zip
, tar.xz
, tar.lz
, tar.gz
, tar.bz2
, dir
(unpacked directory).dmg
, pkg
, mas
.deb
), rpm
, freebsd
, pacman
, p5p
, apk
.nsis
(Installer), nsis-web
(Web installer), portable
(portable app without installation), AppX (Windows Store), Squirrel.Windows.Question | Answer |
---|---|
“I want to configure electron-builder” | See options |
“I have a question” | Open an issue or join the chat |
“I found a bug” | Open an issue |
“I want to donate” | Donate with Donorbox or Paypal |
Real project example — onshape-desktop-shell.
Yarn is strongly recommended instead of npm.
Note: Platform specific 7zip-bin-*
packages are optionalDependencies
, which may require manual install if you have npm configured to not install optional deps by default.
Specify the standard fields in the application package.json
— name, description
, version
and author.
Specify the build configuration in the package.json
as follows:
"build": {
"appId": "your.id",
"mac": {
"category": "your.app.category.type"
}
}
See all options.
Create a directory build in the root of the project and save a background.png
(macOS DMG background), icon.icns
(macOS app icon) and icon.ico
(Windows app icon) into it.
The Linux icon set will be generated automatically based on the macOS icns
file (or you can put them into the build/icons
directory if you want to specify them yourself. The filename must contain the size (e.g. 32x32.png
) of the icon).
Add the scripts key to the development package.json
:
"scripts": {
"pack": "build --dir",
"dist": "build"
}
Then you can run npm run dist
(to package in a distributable format (e.g. dmg, windows installer, deb package)) or npm run pack
(only generates the package directory without really packaging it. This is useful for testing purposes).
To ensure your native dependencies are always matched electron version, simply add "postinstall": "install-app-deps"
to your package.json
.
If you have native addons of your own that are part of the application (not as a dependency), add "nodeGypRebuild": true
to the build
section of your development package.json
.
:bulb: Don't use npm (neither .npmrc
) for configuring electron headers. Use node-gyp-rebuild bin instead.
Installing the required system packages.
Please note that everything is packaged into an asar archive by default.
For an app that will be shipped to production, you should sign your application. See Where to buy code signing certificates.
electron-builder
produces all required artifacts, for example, for macOS:
.dmg
: macOS installer, required for the initial installation process on macOS.-mac.zip
: required for Squirrel.Mac.See the Auto Update section of the Wiki.
Execute node_modules/.bin/build --help
to get the actual CLI usage guide.
Building:
--mac, -m, -o, --macos Build for macOS, accepts target list (see
https://goo.gl/HAnnq8). [array]
--linux, -l Build for Linux, accepts target list (see
https://goo.gl/O80IL2) [array]
--win, -w, --windows Build for Windows, accepts target list (see
https://goo.gl/dL4i8i) [array]
--x64 Build for x64 [boolean]
--ia32 Build for ia32 [boolean]
--armv7l Build for armv7l [boolean]
--dir Build unpacked dir. Useful to test. [boolean]
--prepackaged, --pd The path to prepackaged app (to pack in a
distributable format)
--projectDir, --project The path to project directory. Defaults to current
working directory.
--config, -c The path to an electron-builder config. Defaults to
`electron-builder.yml` (or `json`, or `json5`), see
https://goo.gl/YFRJOM
Publishing:
--publish, -p Publish artifacts (to GitHub Releases), see
https://goo.gl/WMlr4n
[choices: "onTag", "onTagOrDraft", "always", "never"]
--draft Create a draft (unpublished) release [boolean]
--prerelease Identify the release as a prerelease [boolean]
Deprecated:
--platform The target platform (preferred to use --mac, --win or --linux)
[choices: "mac", "win", "linux", "darwin", "win32", "all"]
--arch The target arch (preferred to use --x64 or --ia32)
[choices: "ia32", "x64", "all"]
Other:
--help Show help [boolean]
--version Show version number [boolean]
Examples:
build -mwl build for macOS, Windows and Linux
build --linux deb tar.xz build deb and tar.xz for Linux
build --win --ia32 build for Windows ia32
build --em.foo=bar set package.json property `foo` to `bar`
build --config.nsis.unicode=false configure unicode options for NSIS
See node_modules/electron-builder/out/electron-builder.d.ts
. Typings is supported.
"use strict"
const builder = require("electron-builder")
const Platform = builder.Platform
// Promise is returned
builder.build({
targets: Platform.MAC.createTarget(),
config: {
"//": "build options, see https://goo.gl/ZhRfla"
}
})
.then(() => {
// handle result
})
.catch((error) => {
// handle error
})
You can use electron-builder only to pack your electron app in a AppImage, Snaps, Debian package, NSIS, macOS installer component package (pkg
)
and other distributable formats.
./node_modules/.bin/build --prepackaged <packed dir>
--projectDir
(the path to project directory) option also can be useful.
electron-builder on Slack (please use threads). Public archive without registration.
See the Wiki for more documentation.
FAQs
A complete solution to package and build a ready for distribution Electron app for MacOS, Windows and Linux with “auto update” support out of the box
The npm package electron-builder receives a total of 249,729 weekly downloads. As such, electron-builder popularity was classified as popular.
We found that electron-builder demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.