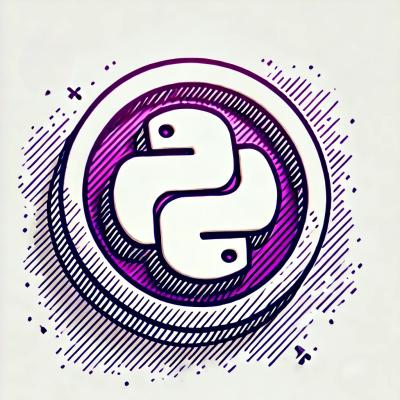
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
electron-builder
Advanced tools
Complete solution to build ready for distribution and 'auto update' Electron App installers
electron-builder is a complete solution to package and build a ready-for-distribution Electron app with “auto update” support out of the box. It supports numerous target formats and platforms, making it a versatile tool for Electron app developers.
Building for Multiple Platforms
This feature allows you to build your Electron app for multiple platforms such as Windows, macOS, and Linux. The code sample demonstrates how to configure and initiate a build process for a Windows target.
const builder = require('electron-builder');
builder.build({
targets: builder.Platform.WINDOWS.createTarget(),
config: {
appId: 'com.example.app',
productName: 'ExampleApp',
directories: {
output: 'dist'
}
}
}).then(() => {
console.log('Build complete!');
}).catch((error) => {
console.error('Error during build:', error);
});
Auto Update
The auto-update feature allows your app to automatically check for updates and install them. The code sample shows how to set up the auto-updater to check for updates when the app is ready and handle update events.
const { autoUpdater } = require('electron-updater');
app.on('ready', () => {
autoUpdater.checkForUpdatesAndNotify();
});
autoUpdater.on('update-available', () => {
console.log('Update available.');
});
autoUpdater.on('update-downloaded', () => {
autoUpdater.quitAndInstall();
});
Custom Configuration
This feature allows you to customize the build configuration for your Electron app. The code sample demonstrates how to specify custom directories, files, and target formats for different platforms.
const builder = require('electron-builder');
builder.build({
config: {
appId: 'com.example.app',
productName: 'ExampleApp',
directories: {
output: 'dist'
},
files: [
'build/**/*'
],
extraResources: [
'assets/'
],
win: {
target: 'nsis'
},
mac: {
target: 'dmg'
}
}
}).then(() => {
console.log('Build complete!');
}).catch((error) => {
console.error('Error during build:', error);
});
electron-packager is a command-line tool and Node.js library that bundles Electron-based application source code with a renamed Electron executable and supporting files into folders ready for distribution. Unlike electron-builder, it does not include auto-update support out of the box and is generally considered less feature-rich.
electron-forge is a complete tool for creating, publishing, and installing modern Electron applications. It provides a more integrated development experience compared to electron-builder, including project scaffolding, packaging, and publishing. However, it may not offer the same level of customization and flexibility in build configurations.
electron-updater is a standalone module that provides auto-update functionality for Electron apps. While electron-builder includes auto-update support as part of its feature set, electron-updater can be used independently or in conjunction with other packaging tools like electron-packager.
Complete solution to build ready for distribution and "auto update" installers of your app for OS X, Windows and Linux.
electron-packager, appdmg and windows-installer are used under the hood.
Real project example — onshape-desktop-shell.
package.json
:Standard name
, description
, version
and author
.
Custom build
field must be specified:
"build": {
"app-bundle-id": "your.id",
"app-category-type": "your.app.category.type",
"iconUrl": "(windows only) A URL to an ICO file to use as the application icon, see details below"
}
This object will be used as a source of electron-packager options. You can specify any other options here.
Create directory build
in the root of the project and put your background.png
(OS X DMG background), icon.icns
(OS X app icon) and icon.ico
(Windows app icon).
Linux icon set will be generated automatically on the fly from the OS X icns
file.
Add scripts to the development package.json
:
"scripts": {
"postinstall": "install-app-deps",
"pack": "build",
"dist": "build"
}
And then you can run npm run pack
or npm run dist
(to package in a distributable format (e.g. DMG, windows installer, NuGet package)).
Install required system packages.
Please note — local icon file url is not accepted, must be https/http.
https://raw.githubusercontent.com/${info.user}/${info.project}/master/build/icon.ico
by default.In the development package.json
custom build
field can be specified to customize distributable format:
"build": {
"osx": {
"title": "computed name from app package.js, you can overwrite",
"icon": "build/icon.icns",
"icon-size": 80,
"background": "build/background.png",
"contents": [
{
"x": 410,
"y": 220,
"type": "link",
"path": "/Applications"
},
{
"x": 130,
"y": 220,
"type": "file",
"path": "computed path to artifact, do not specify it - will be overwritten"
}
]
},
"win": "see https://github.com/electronjs/windows-installer#usage"
}
As you can see, you need to customize OS X options only if you want to provide custom x, y
.
Don't customize paths to background and icon, — just follow conventions (if you don't want to use build
as directory of resources — please create issue to ask ability to customize it).
See OS X options and Windows options.
electron-builder
produces all required artifacts:
.dmg
: OS X installer, required for OS X user to initial install.-mac.zip
: required for Squirrel.Mac..exe
and -x64.exe
: Windows installer, required for Windows user to initial install. Please note — your app must handle Squirrel.Windows events. See real example..full-nupkg
: required for Squirrel.Windows.-amd64.deb
and -i386.deb
: Linux Debian package. Please note — by default the most effective xz compression format used.You need to deploy somewhere releases/downloads server. Consider to use Nuts (GitHub as a backend to store assets) or Electron Release Server.
In general, there is a possibility to setup it as a service for all (it is boring to setup own if cloud service is possible). May be it will be soon (feel free to file an issue to track progress). It is safe since you should sign your app in any case (so, even if server will be compromised, users will not be affected because OS X will just block unsigned/unidentified app).
OS X and Windows code singing is supported.
On a development machine set environment variable CSC_NAME
to your identity (recommended). Or pass --sign
parameter.
export CSC_NAME="Developer ID Application: Your Name (code)"
To sign app on build server:
*.p12
file (e.g. on Google Drive).CSC_LINK
and CSC_KEY_PASSWORD
environment variables:travis encrypt "CSC_LINK='https://drive.google.com/uc?export=download&id=***'" --add
travis encrypt 'CSC_KEY_PASSWORD=beAwareAboutBashEscaping!!!' --add
CFBundleVersion
(OS X) and FileVersion
(Windows) will be set automatically to version
.build_number
on CI server (Travis, AppVeyor and CircleCI supported).
Execute node_modules/.bin/build --help
to get actual CLI usage guide.
In most cases you should not explicitly pass flags, so, we don't want to promote it here (npm lifecycle is supported and script name is taken in account).
Want more — please file issue.
See node_modules/electron-builder/out/electron-builder.d.ts
. Typings is supported.
Old API is deprecated, but not dropped. You can use it as before. Please note — new API by default produces Squirrel.Windows installer, set target
to build NSIS:
build --target=nsis
FAQs
A complete solution to package and build a ready for distribution Electron app for MacOS, Windows and Linux with “auto update” support out of the box
We found that electron-builder demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.