What is electron?
Electron is a framework for creating native applications with web technologies like JavaScript, HTML, and CSS. It combines the Chromium rendering engine and the Node.js runtime, allowing you to build cross-platform desktop applications.
What are electron's main functionalities?
Creating a Browser Window
This feature allows you to create a new browser window in your Electron application. The code sample demonstrates how to create a window and load a URL into it.
const { app, BrowserWindow } = require('electron');
app.on('ready', () => {
const mainWindow = new BrowserWindow({ width: 800, height: 600 });
mainWindow.loadURL('https://example.com');
});
Inter-Process Communication (IPC)
Electron provides IPC (Inter-Process Communication) to allow communication between the main process and renderer processes. The code sample shows how to send and receive messages asynchronously.
const { ipcMain, ipcRenderer } = require('electron');
// Main process
ipcMain.on('asynchronous-message', (event, arg) => {
console.log(arg); // prints 'ping'
event.reply('asynchronous-reply', 'pong');
});
// Renderer process
ipcRenderer.send('asynchronous-message', 'ping');
ipcRenderer.on('asynchronous-reply', (event, arg) => {
console.log(arg); // prints 'pong'
});
Using Node.js Modules
Electron allows you to use Node.js modules in your application. The code sample demonstrates how to use the 'fs' module to read a file.
const fs = require('fs');
fs.readFile('/path/to/file', (err, data) => {
if (err) throw err;
console.log(data.toString());
});
Packaging the Application
Electron applications can be packaged for distribution using tools like 'electron-packager'. The code sample shows how to package an Electron app for Windows using a child process.
const { exec } = require('child_process');
exec('electron-packager . myApp --platform=win32 --arch=x64', (err, stdout, stderr) => {
if (err) {
console.error(`exec error: ${err}`);
return;
}
console.log(`stdout: ${stdout}`);
console.error(`stderr: ${stderr}`);
});
Other packages similar to electron
nw
NW.js (previously known as node-webkit) is another framework for building desktop applications using web technologies. It also combines Node.js and Chromium but offers different APIs and a different approach to application architecture compared to Electron.
proton-native
Proton Native is a framework for building native desktop applications using React. Unlike Electron, which uses web technologies and Chromium, Proton Native uses native components for rendering, which can result in better performance and a more native look and feel.
electron-prebuilt

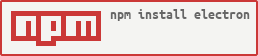
Install Electron prebuilt binaries for
command-line use using npm. This module helps you easily install the electron
command for use on the command line without having to compile anything.
Electron is a JavaScript runtime that bundles Node.js
and Chromium. You use it similar to the node
command on the command line for
executing JavaScript programs. For more info you can read this intro blog post
or dive into the Electron documentation.
Installation
Note As of version 1.3.1, this package is published to npm under two names:
electron
and electron-prebuilt
. You can currently use either name, but
electron
is recommended, as the electron-prebuilt
name is deprecated, and
will only be published until the end of 2016.
Download and install the latest build of Electron for your OS and add it to your
project's package.json
as a devDependency
:
npm install electron --save-dev
This is the preferred way to use Electron, as it doesn't require users to
install Electron globally.
You can also use the -g
flag (global) to symlink it into your PATH:
npm install -g electron
If that command fails with an EACCESS
error you may have to run it again with sudo
:
sudo npm install -g electron
Now you can just run electron
to run electron:
electron
If you need to use an HTTP proxy you can set these environment variables.
If you want to change the architecture that is downloaded (e.g., ia32
on an
x64
machine), you can use the --arch
flag with npm install or set the
npm_config_arch
environment variable:
npm install --arch=ia32 electron
About
Works on Mac, Windows and Linux OSes that Electron supports (e.g. Electron
does not support Windows XP).
The version numbers of this module match the version number of the official
Electron releases, which
do not follow semantic versioning.
This module is automatically released whenever a new version of Electron is
released thanks to electron-prebuilt-updater,
originally written by John Muhl.
Usage
First, you have to write an Electron application.
Then, you can run your app using:
electron your-app/
Related modules
- electron-packager -
Package and distribute your Electron app with OS-specific bundles
(.app, .exe etc)
- electron-builder -
create installers
- menubar - high level way to create
menubar desktop applications with electron
Find more at the awesome-electron list.
Programmatic usage
Most people use this from the command line, but if you require electron
inside
your Node app (not your Electron app) it will return the file path to the
binary. Use this to spawn Electron from Node scripts:
var electron = require('electron')
var proc = require('child_process')
console.log(electron)
var child = proc.spawn(electron)