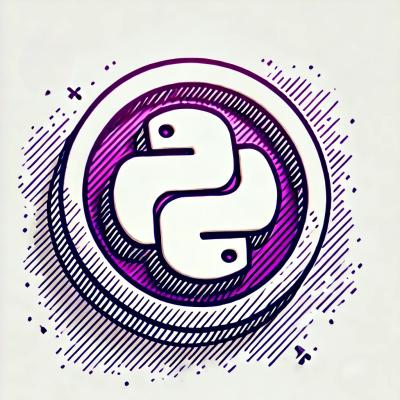
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
elvalidator
Advanced tools
ElValidator is a JSON Object validator and sanitizer. It's a great little tool for validating user input and sanitizing. It's heavily inspired from the familiar Mongoose schema format with a few more features. (Note: ElValidator doesn't attempt to strictly match the Mongoose validator)
$ npm install elvalidator --save
Or in the browser:
<script type="text/javascript" src="https://unpkg.com/elvalidator/elvalidator.min.js"></script>
import ElValidator from 'elvalidator';
// Set up the validator object
//let validator = new ElValidator(schema, options);
let validator = new ElValidator({
name : { type: String, required:true, trim:true, minlength:3 },
age : { type: Number, required:true, integer:true, min:18, max:100 },
agreedTelemetry : { type: Boolean, default:false },
// Array example:
tags : [
{ type: String, minlength:3, lowercase:true, trim:true, match: /^[a-z0-9]+$/ }
],
// Object example:
settings : {
darkMode : { type: Boolean, default:false },
codeEditor : { type: String, required:false, default:'atom', enum:['atom', 'vstudio', 'notepad++'] },
}
}, {
strictMode : true, // When disabled, the validator will go easy on the errors. (i.e. a number is provided instead of a string the number will be converted to a string instead of throwing an error)
throwUnkownFields : false,// Throw error when an unknown field is present
accumulateErrors : false,// Validate everything and then show big error message (vs. throw error as soon as an issue is detected)
});
// Validate/Sanitize a data object
// If there's an error, it will be thrown. You can thus use a try ... catch block to process the errors.
var sanitizedData = await validator.validate({
name : 'Yassine',
age : 27,
tags : ['PROgrammer', 'javascript '],
settings : {
darkMode : false,
},
other : 'unknown field',
});
console.log(sanitizedData);
/*
Outputs:
{
name: 'Yassine',
age: 27,
agreedTelemetry: false,
tags: [ 'programmer', 'javascript' ],
settings: { darkMode: false, codeEditor: 'atom' }
}
*/
{
field : {
type : String,
name : "Field Name", // For cleaner error messages
required : false, // Is this a mandatory field?
default : '', // If provided, the required field is not considered
lowercase : false, // Force string to lower case
uppercase : false, // Force string to UPPER CASE
trim : false, // Trim text (remove whitespace at the start and end of the string)
minlength : 3, // Minimum length of the string
maxlength : 15, // Maximum length of the string
enum : ['hello', 'world'], // Array with valid values for this field
match : /^(hello|world)$/i, // String must match with this regex
validator : async (value) => { // Manually validate/sanitize the value
if(!value)
throw new Error('Invalid value');
return value;
},
}
}
{
field : {
type : Number,
name : "Field Name", // For cleaner error messages
required : false, // Is this a mandatory field?
default : 0, // If provided, the required field is not considered
integer : false, // Force number to be an integer
min : 3, // Minimum value of the number
max : 15, // Maximum value of the number
validator : async (value) => { // Manually validate/sanitize the value
if(!value)
throw new Error('Invalid value');
return value;
},
}
}
{
field : {
type : Boolean,
name : "Field Name", // For cleaner error messages
required : false, // Is this a mandatory field?
default : false, // If provided, the required field is not considered
validator : async (value) => { // Manually validate/sanitize the value
if(!value)
throw new Error('Invalid value');
return value;
},
}
}
{
// With default options
field : [
{ type: String } // Define the schema for the values this array should allow, in this case it accepts strings
],
// OR with options
field : {
type : [
{ type: String } // Define the schema for the values this array should allow, in this case it accepts strings
],
name : "Field Name", // For cleaner error messages
required : false, // Is this a mandatory field?
default : [], // If provided, the required field is not considered
minEntries : 0, // Minimum number of entries this array can hold
maxEntries : 10, // Maximum number of entries this array can hold
uniqueValues: false, // Whether to filter out the values of the array to only return non-repeating values
validator : async (value) => { // Manually validate/sanitize the value
if(!value)
throw new Error('Invalid value');
return value;
},
}
}
This type allows you to accept Objects with arbitrary fields and no particular validation.
{
field : {
type : Object,
name : "Field Name", // For cleaner error messages
required : false, // Is this a mandatory field?
default : {}, // If provided, the required field is not considered
validator : async (value) => { // Manually validate/sanitize the value
if(!value)
throw new Error('Invalid value');
return value;
},
}
}
{
field : {
subField1 : { type: String },
subField2 : { type: Number },
subField3 : {
type : { type: String }, // Here type is a sub-sub-field and accepts strings
subSubField2 : { type: Number },
},
}
}
{
field : {
$or : [ // You can define different possible types for this particular field using the $or operator
{ type: String, }, // field can either be a string,
{ type: Number, min:0, max:10}, // a number between 0 and 10
{ type: Number, min:100, max:1000, integer: true}, // or an integer between 100 and 1000
],
default : '',
required : true,
}
}
A few validators are available to make your life easier.
{
url : ElValidator.Builtins.Url,
domainName : ElValidator.Builtins.DomainName,
email : ElValidator.Builtins.Email,
youtubeVideo : ElValidator.Builtins.YoutubeVideo,
vimeoVideo : ElValidator.Builtins.VimeoVideo,
}
// will validate for:
{
url : 'https://www.example.com/index.html?query=param',
domainName : 'www.example.com',
email : 'user@example.com',
youtubeVideo : 'https://www.youtube.com/watch?v=UNG7vNchvVQ',
vimeoVideo : 'https://vimeo.com/347119375',
}
[1.0.0] - 2022-03-05
FAQs
Validate and Sanitize JSON objects.
The npm package elvalidator receives a total of 4 weekly downloads. As such, elvalidator popularity was classified as not popular.
We found that elvalidator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.