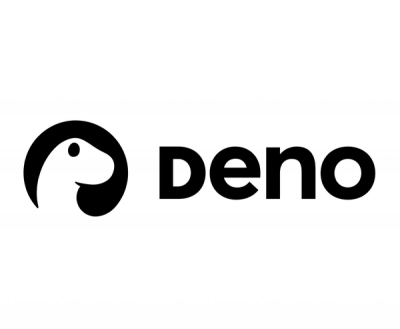
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
ember-backoff
Advanced tools
Simple exponential backoff strategy for Ember.js promises
import retryWithBackoff from 'ember-backoff/retry-with-backoff';
export default Em.Route.extend({
model: function(params) {
retryWithBackoff(function() {
return this.store.find('user', 142857); //return any promise here
}, 5, 100); //retry 5 times: 100ms, 200ms, 400ms, 800ms, 1600ms between tries
}
});
Or using one of the supplied strategies
import retryWithBackoff from 'ember-backoff/retry-with-backoff';
import constant from 'ember-backoff/strategy/constant';
export default Em.Route.extend({
model: function(params) {
retryWithBackoff(function() {
// poll until record exists
return this.store.find('completedJob', 22);
}, 10, 1000, constant); //retry 10 times: always 1s between tries
}
});
Or you own custom strategy
import retryWithBackoff from 'ember-backoff/retry-with-backoff';
import exponential from 'ember-backoff/strategy/exponential';
function exponentialWithDither(initialWait, retryCount) {
var ditherFrac = Math.random() * 10;
return (1 + ditherFrac) * exponential(initialWait, retryCount);
}
export default Em.Route.extend({
model: function(params) {
retryWithBackoff(function() {
return this.store.query('thunderindHerd');
}, 10, 1000, exponentialWithDither);
}
});
Questions? Ping me @gavinjoyce
npm install ember-backoff --save
Pull requests are very welcome, thanks.
git clone
this repositorynpm install
bower install
ember server
ember test
ember test --server
FAQs
Exponential backoff and retry for rsvp.js promises
We found that ember-backoff demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.