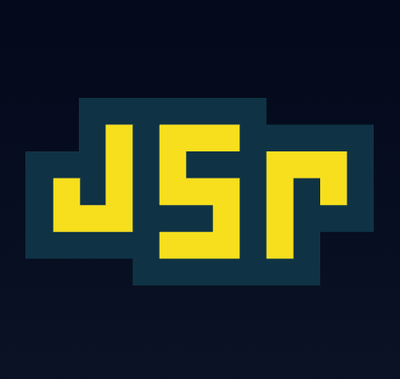
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
ember-cli-babel
Advanced tools
ember-cli-babel is an Ember CLI addon that provides Babel transpilation for Ember.js applications. It allows developers to use the latest JavaScript features by transpiling ES6+ code to ES5, ensuring compatibility with older browsers.
Transpile ES6+ to ES5
This feature allows you to transpile modern JavaScript (ES6+) to ES5, making your code compatible with older browsers. The code sample shows how to configure ember-cli-babel in an Ember CLI project to include the Babel polyfill.
module.exports = function(defaults) {
let app = new EmberApp(defaults, {
'ember-cli-babel': {
includePolyfill: true
}
});
return app.toTree();
};
Custom Babel Plugins
You can add custom Babel plugins to your Ember CLI project. The code sample demonstrates how to include the '@babel/plugin-proposal-class-properties' plugin.
module.exports = function(defaults) {
let app = new EmberApp(defaults, {
babel: {
plugins: ['@babel/plugin-proposal-class-properties']
}
});
return app.toTree();
};
Custom Babel Presets
This feature allows you to use custom Babel presets in your Ember CLI project. The code sample shows how to include the '@babel/preset-env' preset.
module.exports = function(defaults) {
let app = new EmberApp(defaults, {
babel: {
presets: ['@babel/preset-env']
}
});
return app.toTree();
};
babel-loader is a webpack loader that allows you to transpile JavaScript files using Babel and webpack. It is similar to ember-cli-babel in that it enables the use of modern JavaScript features, but it is used in the context of a webpack build process rather than an Ember CLI project.
gulp-babel is a Gulp plugin that allows you to use Babel to transpile your JavaScript files. Like ember-cli-babel, it helps you write modern JavaScript while ensuring compatibility with older browsers. However, it is used within a Gulp build system instead of Ember CLI.
rollup-plugin-babel is a Rollup plugin that integrates Babel into the Rollup bundler. It provides similar functionality to ember-cli-babel by allowing you to transpile modern JavaScript code, but it is used within a Rollup build process.
This Ember-CLI plugin uses Babel and babel-preset-env to allow you to use ES6 syntax with your Ember CLI project.
ember install ember-cli-babel
This plugin should work without any configuration after installing. By default
it will take every .js
file in your project and run it through the Babel
transpiler to convert your ES6 code to code supported by your target browsers
(as specified in config/targets.js
in ember-cli >= 2.13). Running non-ES6
code through the transpiler shouldn't change the code at all (likely just a
format change if it does).
If you need to customize the way that babel-preset-env
configures the plugins
that transform your code, you can do it by passing in any of the options found
here. Note:
.babelrc
files are ignored by default.
Example (configuring babel directly):
// ember-cli-build.js
let app = new EmberApp({
babel: {
// enable "loose" mode
loose: true,
// don't transpile generator functions
exclude: [
'transform-regenerator',
],
plugins: [
'transform-object-rest-spread'
]
}
});
Example (configuring ember-cli-babel itself):
// ember-cli-build.js
let app = new EmberApp({
'ember-cli-babel': {
compileModules: false
}
});
There are a few different options that may be provided to ember-cli-babel.
These options are typically set in an apps ember-cli-build.js
file, or in an
addon or engine's index.js
.
type BabelPlugin = string | [string, any] | [any, any];
interface EmberCLIBabelConfig {
/**
Configuration options for babel-preset-env.
See https://github.com/babel/babel-preset-env/tree/v1.6.1#options for details on these options.
*/
babel?: {
spec?: boolean;
loose?: boolean;
debug?: boolean;
include?: string[];
exclude?: string[];
useBuiltIns?: boolean;
sourceMaps?: boolean | "inline" | "both";
plugins?: BabelPlugin[];
};
/**
Configuration options for ember-cli-babel itself.
*/
'ember-cli-babel'?: {
includePolyfill?: boolean;
compileModules?: boolean;
disableDebugTooling?: boolean;
disablePresetEnv?: boolean;
disableEmberModulesAPIPolyfill?: boolean;
extensions?: string[];
};
}
The exact location you specify these options varies depending on the type of
project you're working on. As a concrete example, to add
babel-plugin-transform-object-rest-spread
so that your project can use object
rest/spread syntax, you would do something like this in an app:
// ember-cli-build.js
let app = new EmberApp(defaults, {
babel: {
plugins: ['transform-object-rest-spread']
}
});
In an engine:
// index.js
module.exports = EngineAddon.extend({
babel: {
plugins: ['transform-object-rest-spread']
}
});
In an addon:
// index.js
module.exports = {
options: {
babel: {
plugins: ['transform-object-rest-spread']
}
}
};
Babel comes with a polyfill that includes a custom regenerator runtime and core-js. Many transformations will work without it, but for full support you may need to include the polyfill in your app.
To include it in your app, pass includePolyfill: true
in your ember-cli-babel
options.
// ember-cli-build.js
let app = new EmberApp(defaults, {
'ember-cli-babel': {
includePolyfill: true
}
});
Babel generated source maps will enable you to debug your original ES6 source code. This is disabled by default because it will slow down compilation times.
To enable it, pass sourceMaps: 'inline'
in your babel
options.
// ember-cli-build.js
let app = new EmberApp(defaults, {
babel: {
sourceMaps: 'inline'
}
});
Older versions of Ember CLI (< 2.12
) use its own ES6 module transpiler.
Because of that, this plugin disables Babel module compilation by blacklisting
that transform when running under affected ember-cli versions. If you find that
you want to use the Babel module transform instead of the Ember CLI one, you'll
have to explicitly set compileModules
to true
in your configuration. If
compileModules
is anything other than true
, this plugin will leave the
module syntax compilation up to Ember CLI.
If for some reason you need to disable this debug tooling, you can opt-out via configuration.
In an app that would look like:
// ember-cli-build.js
module.exports = function(defaults) {
let app = new EmberApp(defaults, {
'ember-cli-babel': {
disableDebugTooling: true
}
});
return app.toTree();
}
You can add custom plugins to be used during transpilation of the addon/
or
addon-test-support/
trees by ensuring that your addon's options.babel
is
properly populated (as mentioned above in the Options
section).
For addons which want additional customizations, they are able to interact with this addon directly.
interface EmberCLIBabel {
/**
Used to generate the options that will ultimately be passed to babel itself.
*/
buildBabelOptions(config?: EmberCLIBabelConfig): Opaque;
/**
Supports easier transpilation of non-standard input paths (e.g. to transpile
a non-addon NPM dependency) while still leveraging the logic within
ember-cli-babel for transpiling (e.g. targets, preset-env config, etc).
*/
transpileTree(inputTree: BroccoliTree, config?: EmberCLIBabelConfig): BroccoliTree;
/**
Used to determine if a given plugin is required by the current target configuration.
Does not take `includes` / `excludes` into account.
See https://github.com/babel/babel-preset-env/blob/master/data/plugins.json for the list
of known plugins.
*/
isPluginRequired(pluginName: string): boolean;
}
buildBabelOptions
usage// find your babel addon (can use `this.findAddonByName('ember-cli-babel')` in ember-cli@2.14 and newer)
let babelAddon = this.addons.find(addon => addon.name === 'ember-cli-babel');
// create the babel options to use elsewhere based on the config above
let options = babelAddon.buildBabelOptions(config)
// now you can pass these options off to babel or broccoli-babel-transpiler
require('babel-core').transform('something', options);
transpileTree
usage// find your babel addon (can use `this.findAddonByName('ember-cli-babel')` in ember-cli@2.14 and newer)
let babelAddon = this.addons.find(addon => addon.name === 'ember-cli-babel');
// invoke .transpileTree passing in the custom input tree
let transpiledCustomTree = babelAddon.transpileTree(someCustomTree);
In order to allow apps and addons to easily provide good development mode ergonomics (assertions, deprecations, etc) but still perform well in production mode ember-cli-babel automatically manages stripping / removing certain debug statements. This concept was originally proposed in ember-cli/rfcs#50, but has been slightly modified during implementation (after researching what works well and what does not).
To add convienient deprecations and assertions, consumers (in either an app or an addon) can do the following:
import { deprecate, assert } from '@ember/debug';
export default Ember.Component.extend({
init() {
this._super(...arguments);
deprecate(
'Passing a string value or the `sauce` parameter is deprecated, please pass an instance of Sauce instead',
false,
{ until: '1.0.0', id: 'some-addon-sauce' }
);
assert('You must provide sauce for x-awesome.', this.sauce);
}
})
In testing and development environments those statements will be executed (and assert or deprecate as appropriate), but in production builds they will be inert (and stripped during minification).
The following are named exports that are available from @ember/debug
:
function deprecate(message: string, predicate: boolean, options: any): void
- Results in calling Ember.deprecate
.function assert(message: string, predicate: boolean): void
- Results in calling Ember.assert
.function warn(message: string, predicate: boolean): void
- Results in calling Ember.warn
.In some cases you may have the need to do things in debug builds that isn't
related to asserts/deprecations/etc. For example, you may expose certain API's
for debugging only. You can do that via the DEBUG
environment flag:
import { DEBUG } from '@glimmer/env';
const Component = Ember.Component.extend();
if (DEBUG) {
Component.reopen({
specialMethodForDebugging() {
// do things ;)
}
});
}
In testing and development environments DEBUG
will be replaced by the boolean
literal true
, and in production builds it will be replaced by false
. When
ran through a minifier (with dead code elimination) the entire section will be
stripped.
Please note, that these general purpose environment related flags (e.g. DEBUG
as a boolean flag) are imported from @glimmer/env
not from an @ember
namespace.
By default, [broccoli-babel-transpiler] will attempt to spin up several sub-processes (~1 per available core), to achieve parallelization. (Once node has built-in worker support, we plan to utilize). This yields significant babel build time improvements.
Unfortunately, some babel-plugins may break this functionality More Details. When this occurs, we gracefully fallback to the old serial strategy.
To have the build fail when failing to do parallel builds, opt-in is via:
let app = new EmberAddon(defaults, {
'ember-cli-babel': {
throwUnlessParallelizable: true
}
});
note: future versions will enable this flag by default
FAQs
Ember CLI addon for Babel
The npm package ember-cli-babel receives a total of 540,276 weekly downloads. As such, ember-cli-babel popularity was classified as popular.
We found that ember-cli-babel demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.