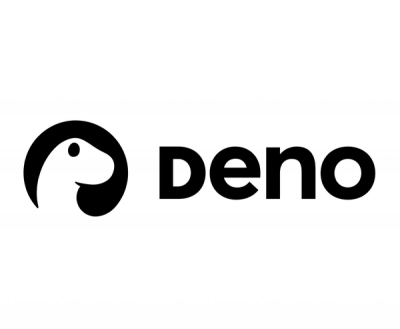
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
ember-cli-barcode
Advanced tools
An ember-cli addon to render barcodes in Ember applications using the JsBarcode library. See the demo
ember-cli-barcode is compatible with Ember 2.4 onward and is passing tests for Ember 3.x. ember-cli-barcode version 2.0 onward no longer requires Bower - thanks @donaldwasserman
After upgrading to 2.x, if jsbarcode was your only Bower dependency you can remove bower.json from your project and delete the bower_components directory. If you have other Bower dependencies, remove the jsbarcode dependency from bower.json
$ ember install ember-cli-barcode
Configure the addon options below in ember-cli-build.js
. See the configuration section for slimming your build
// ember-cli-build.js
const EmberApp = require('ember-cli/lib/broccoli/ember-app');
module.exports = function (defaults) {
let app = new EmberApp(defaults, {
'ember-cli-barcode': {
include: 'all'
}
});
return app.toTree();
};
The simplest form to render a barcode is to pass in a value using the default options which generate a CODE128 barcode:
{{bar-code value="abc123456"}}
Which renders:
By default, barcodes are rendered using the svg
element. The element can be changed to img
or canvas
using the tagName property:
{{bar-code
value="A45689"
tagName="img"}}
{{bar-code
value="A45689"
tagName="canvas"}}
Use the img
tag if you want the ability to right-click copy or save the displayed barcode.
All JsBarcode's options are supported by the addon. See Barcode Specifications for details on each format. A few examples are below. See the demo application for more.
Change the barcode format by passing the format name into the component. To display a UPC barcode:
{{bar-code
value="123456789999"
format="UPC"}}
The color of the barcode or it's background can be changed:
{{bar-code
value="abc123456"
lineColor="red"}}
background color changed:
{{bar-code
value="abc123456"
lineColor="#ffffff"
background="#660033"}}
Any valid html or hexadecimal color can be used for the lineColor
or background
options. The component does not support the Ember component blockform.
If you have many options, pass an object using the options
parameter instead of passing a large number of individual parameters. The options
will override any other parameters set on the component.
// app/controllers/application.js
myOptions: {
format: "UPC",
textPosition: "top",
lineColor: "#ff3399",
}
{{!-- app/templates/application.hbs --}}
{{bar-code
value="11223344"
options=myOptions}}
{{!-- options override other settings --}}
{{!-- line color will be ##ff3399 --}}
{{bar-code
value="11223344"
options=myOptions
lineColor="blue"}}
The flat
option is supported for both EAN13 and UPC barcodes defaulting to false
if not specified Additionally the lastChar
option is supported for EAN13 barcodes with a default value of ''.
If you pass an invalid value based on the format, the barcode will not render. To capture invalid values assign an action to the vaild
property.
// app/templates/application.hbs
// pass invalid code for EAN8 barcodes
{{bar-code format="EAN8" value="9638" valid=(action 'checkValid')}}
{{if validCode "Valid" "Invalid"}}
// app/controllers/application.js
import Controller from '@ember/controller';
export default Controller.extend({
validCode: false,
actions: {
checkValid (status) {
this.set('validCode', status)
},
}
});
IF you have have multiple barcodes in a template and want to check the validity of each individually, you would need a dedicated action and controller property for each barcode.
By default, this addon provides the JsBarcode.all
javascript file. If you are looking to slim your build and only need a specific version provided by the upstream package,
you can pass the type of file you'd like to include via the include
option. If you supply all
or a falsy value, the addon will include the default all package.
// ember-cli-build.js
const EmberApp = require('ember-cli/lib/broccoli/ember-app');
module.exports = function (defaults) {
let app = new EmberApp(defaults, {
'ember-cli-barcode': {
include: 'code128'
}
});
return app.toTree();
};
all
codabar
code128
code39
ean-upc
itf-14
itf
msi
pharmacode
.The dummy application allows you to experiment with many of the barcode options. As you select different barcode formats a predefined valid code is selected for rendering. scandit.com has a nice summary of different barcode formats.
To run the dummy application
npm install
or yarn
ember serve
npm install
or yarn
npm test
(Runs ember try:each
to test your addon against multiple Ember versions)ember test
ember test --server
For more information on using ember-cli, visit https://ember-cli.com/.
MIT
FAQs
Ember barcode generator using the JSBarcode library.
The npm package ember-cli-barcode receives a total of 38 weekly downloads. As such, ember-cli-barcode popularity was classified as not popular.
We found that ember-cli-barcode demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.