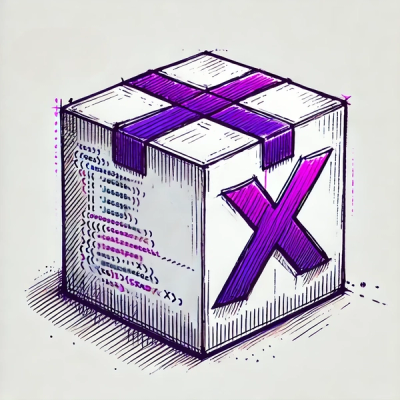
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
ember-composable-helpers
Advanced tools
Composable helpers for Ember that enables more declarative templating. These helpers can be composed together to form powerful ideas:
{{#each (map-by "fullName" users) as |fullName|}}
<input type="text" value={{fullName}} onchange={{action (mut newName)}}>
<button {{action (pipe updateFullName saveUser) newName}}>
Update and save {{fullName}} to {{newName}}
</button>
{{/each}}
To install:
Ember 3.13+:
ember install ember-composable-helpers
Ember 3.12 and below:
ember install ember-composable-helpers@^2.4.0
Watch a free video overview presented by EmberMap:
If you don't need all the helpers, you can specify which to include or remove from your build using only
or except
within your ember-cli-build.js
:
module.exports = function(defaults) {
var app = new EmberApp(defaults, {
'ember-composable-helpers': {
only: ['inc', 'dec', 'pipe'],
except: ['filter-by']
}
});
Both only
and except
can be safely used together (the addon computes the diff), although it's best if you only use one for your own sanity.
except: ['pipe'] // imports all helpers except `pipe`
only: ['pipe'] // imports only `pipe`
This addon is built with composability in mind, and in order to faciliate that, the ordering of arguments is somewhat different then you might be used to.
For all non-unary helpers, the subject of the helper function will always be the last argument. This way the arguments are better readable if you compose together multiple helpers:
{{take 5 (sort-by "lastName" "firstName" (filter-by "active" array))}}
For action helpers, this will mean better currying semantics:
<button {{action (pipe (action "closePopover") (toggle "isExpanded")) this}}>
{{if isExpanded "I am expanded" "I am not"}}
</button>
For help upgrading between major versions, check out the upgrading documentation.
pipe
Pipes the return values of actions in a sequence of actions. This is useful to compose a pipeline of actions, so each action can do only one thing.
<button {{action (pipe (action 'addToCart') (action 'purchase') (action 'redirectToThankYouPage')) item}}>
1-Click Buy
</button>
The pipe
helper is Promise-aware, meaning that if any action in the pipeline returns a Promise, its return value will be piped into the next action. If the Promise rejects, the rest of the pipeline will be aborted.
The pipe
helper can also be used directly as a closure action (using pipe-action
) when being passed into a Component, which provides an elegant syntax for composing actions:
{{foo-bar
addAndSquare=(pipe-action (action "add") (action "square"))
multiplyAndSquare=(pipe-action (action "multiply") (action "square"))
}}
{{! foo-bar/template.hbs }}
<button {{action addAndSquare 2 4}}>Add and Square</button>
<button {{action multiplyAndSquare 2 4}}>Multiply and Square</button>
call
Calls the given function with arguments
{{#each (call (fn this.callMeWith @daysInMonth) as |week|}}
{{#each week as |day|}}
{{day}}
{{/each}}
{{/each}}
compute
Calls an action as a template helper.
The square of 4 is {{compute (action "square") 4}}
toggle
Toggles a boolean value.
<button {{action (toggle "isExpanded" this)}}>
{{if isExpanded "I am expanded" "I am not"}}
</button>
toggle
can also be used directly as a closure action using toggle-action
:
{{foo-bar
toggleIsExpanded=(toggle-action "isExpanded" this)
toggleIsSelected=(toggle-action "isSelected" this)
}}
{{! foo-bar/template.hbs }}
<button {{action toggleIsExpanded}}>Open / Close</button>
<button {{action toggleIsSelected}}>Select / Deselect</button>
toggle
also accepts optional values to rotate through:
<button {{action (toggle "currentName" this "foo" "bar" "baz")}}>
{{currentName}}
</button>
noop
Returns an empty function.
<div {{on "mouseenter" (if @isLoading (noop) @sendTrackingEvent))}}>Some content</div>
optional
Allows for the passed in action to not exist.
<button {{action (optional handleClick)}}>Click Me</button>
queue
Like pipe
, this helper runs actions in a sequence (from left-to-right). The
difference is that this helper passes the original arguments to each action, not
the result of the previous action in the sequence.
If one of the actions in the sequence returns a promise, then it will wait for that promise to resolve before calling the next action in the sequence. If a promise is rejected it will stop the sequence and no further actions will be called.
<button {{action (queue (action "backupData") (action "unsafeOperation") (action "restoreBackup"))}} />
map
Maps a callback on an array.
{{#each (map (action "getName") users) as |fullName|}}
{{fullName}}
{{/each}}
map-by
Maps an array on a property.
{{#each (map-by "fullName" users) as |fullName|}}
{{fullName}}
{{/each}}
sort-by
Sort an array by given properties.
{{#each (sort-by "lastName" "firstName" users) as |user|}}
{{user.lastName}}, {{user.firstName}}
{{/each}}
You can append :desc
to properties to sort in reverse order.
{{#each (sort-by "age:desc" users) as |user|}}
{{user.firstName}} {{user.lastName}} ({{user.age}})
{{/each}}
You can also pass a method as the first argument:
{{#each (sort-by (action "mySortAction") users) as |user|}}
{{user.firstName}} {{user.lastName}} ({{user.age}})
{{/each}}
filter
Filters an array by a callback.
{{#each (filter (action "isActive") users) as |user|}}
{{user.name}} is active!
{{/each}}
filter-by
Filters an array by a property.
{{#each (filter-by "isActive" true users) as |user|}}
{{user.name}} is active!
{{/each}}
If you omit the second argument it will test if the property is truthy.
{{#each (filter-by "address" users) as |user|}}
{{user.name}} has an address specified!
{{/each}}
You can also pass an action as second argument:
{{#each (filter-by "age" (action "olderThan" 18) users) as |user|}}
{{user.name}} is older than eighteen!
{{/each}}
reject-by
The inverse of filter by.
{{#each (reject-by "isActive" true users) as |user|}}
{{user.name}} is not active!
{{/each}}
If you omit the third argument it will test if the property is falsey.
{{#each (reject-by "address" users) as |user|}}
{{user.name}} does not have an address specified!
{{/each}}
You can also pass an action as third argument:
{{#each (reject-by "age" (action "youngerThan" 18) users) as |user|}}
{{user.name}} is older than eighteen!
{{/each}}
find-by
Returns the first entry matching the given value.
{{#with (find-by 'name' lookupName people) as |person|}}
{{#if person}}
{{#link-to 'person' person}}
Click here to see {{person.name}}'s details
{{/link-to}}
{{/if}}
{{/with}}
intersect
Creates an array of unique values that are included in all given arrays.
<h1>Matching skills</h1>
{{#each (intersect desiredSkills currentSkills) as |skill|}}
{{skill.name}}
{{/each}}
invoke
Invokes a method on an object, or on each object of an array.
<div id="popup">
{{#each people as |person|}}
<button {{action (invoke "rollbackAttributes" person)}}>
Undo
</button>
{{/each}}
<a {{action (invoke "save" people)}}>Save</a>
</div>
union
Joins arrays to create an array of unique values. When applied to a single array, has the same behavior as uniq
.
{{#each (union cartA cartB cartC) as |cartItem|}}
{{cartItem.price}} x {{cartItem.quantity}} for {{cartItem.name}}
{{/each}}
take
Returns the first n
entries of a given array.
<h3>Top 3:</h3>
{{#each (take 3 contestants) as |contestant|}}
{{contestant.rank}}. {{contestant.name}}
{{/each}}
drop
Returns an array with the first n
entries omitted.
<h3>Other contestants:</h3>
{{#each (drop 3 contestants) as |contestant|}}
{{contestant.rank}}. {{contestant.name}}
{{/each}}
reduce
Reduce an array to a value.
{{reduce (action "sum") 0 (array 1 2 3)}}
The last argument is initial value. If you omit it, undefined will be used.
repeat
Repeats n
times. This can be useful for making an n-length arbitrary list for iterating upon (you can think of this form as a times helper, a la Ruby's 5.times { ... }
):
{{#each (repeat 3) as |empty|}}
I will be rendered 3 times
{{/each}}
You can also give it a value to repeat:
{{#each (repeat 3 "Adam") as |name|}}
{{name}}
{{/each}}
reverse
Reverses the order of the array.
{{#each (reverse friends) as |friend|}}
If {{friend}} was first, they are now last.
{{/each}}
range
Generates a range of numbers between a min
and max
value.
{{#each (range 10 20) as |number|}}
{{! `number` will go from 10 to 19}}
{{/each}}
It can also be set to inclusive
:
{{#each (range 10 20 true) as |number|}}
{{! `number` will go from 10 to 20}}
{{/each}}
And works with a negative range:
{{#each (range 20 10) as |number|}}
{{! `number` will go from 20 to 11}}
{{/each}}
join
Joins the given array with an optional separator into a string.
{{join ', ' categories}}
compact
Removes blank items from an array.
{{#each (compact arrayWithBlanks) as |notBlank|}}
{{notBlank}} is most definitely not blank!
{{/each}}
contains
:warning: contains
is deprecated. Use includes
instead.
Checks if a given value or sub-array is contained within an array.
{{contains selectedItem items}}
{{contains 1234 items}}
{{contains "First" (w "First Second Third") }}
{{contains (w "First Second") (w "First Second Third")}}
includes
Checks if a given value or sub-array is included within an array.
{{includes selectedItem items}}
{{includes 1234 items}}
{{includes "First" (w "First Second Third") }}
{{includes (w "First Second") (w "First Second Third")}}
append
Appends the given arrays and/or values into a single flat array.
{{#each (append catNames dogName) as |petName|}}
{{petName}}
{{/each}}
chunk
Returns the given array split into sub-arrays the length of the given value.
{{#each (chunk 7 daysInMonth) as |week|}}
{{#each week as |day|}}
{{day}}
{{/each}}
{{/each}}
without
Returns the given array without the given item(s).
{{#each (without selectedItem items) as |remainingItem|}}
{{remainingItem.name}}
{{/each}}
shuffle
Shuffles an array with a randomizer function, or with Math.random
as a default. Your randomizer function should return a number between 0 and 1.
{{#each (shuffle array) as |value|}}
{{value}}
{{/each}}
{{#each (shuffle (action "myRandomizer") array) as |value|}}
{{value}}
{{/each}}
flatten
Flattens an array to a single dimension.
{{#each (flatten anArrayOfNamesWithMultipleDimensions) as |name|}}
Name: {{name}}
{{/each}}
object-at
Returns the object at the given index of an array.
{{object-at index array}}
slice
Slices an array
{{#each (slice 1 3 array) as |value|}}
{{value}}
{{/each}}
next
Returns the next element in the array given the current element. Note: Accepts an optional boolean
parameter, useDeepEqual
, to flag whether a deep equal comparison should be performed.
<button onclick={{action (mut selectedItem) (next selectedItem useDeepEqual items)}}>Next</button>
has-next
Checks if the array has an element after the given element. Note: Accepts an optional boolean
parameter, useDeepEqual
, to flag whether a deep equal comparison should be performed.
{{#if (has-next page useDeepEqual pages)}}
<button>Next</button>
{{/if}}
previous
Returns the previous element in the array given the current element. Note: Accepts an optional boolean
parameter, useDeepEqual
, to flag whether a deep equal comparison should be performed.
<button onclick={{action (mut selectedItem) (previous selectedItem useDeepEqual items)}}>Previous</button>
has-previous
Checks if the array has an element before the given element. Note: Accepts an optional boolean
parameter, useDeepEqual
, to flag whether a deep equal comparison should be performed
{{#if (has-previous page useDeepEqual pages)}}
<button>Previous</button>
{{/if}}
entries
Returns an array of a given object's own enumerable string-keyed property [key, value]
pairs
{{#each (entries object) as |entry|}}
{{get entry 0}}:{{get entry 1}}
{{/each}}
You can pair it with other array helpers too. For example
{{#each (sort-by myOwnSortByFunction (entries myObject)) as |entry|}}
{{get entry 0}}
{{/each}}`);
from-entries
Converts a two-dimensional array of [key, value]
pairs into an Object
{{#each-in (from-entries entries) as |key value|}}
{{key}}:{{value}}
{{/each}}
You can pair it with other array helpers too. For example, to copy only properties with non-falsey values:
{{#each-in (from-entries (filter-by "1" (entries myObject))) as |k v|}}
{{k}}: {{v}}
{{/each-in}}`);
group-by
Returns an object where the keys are the unique values of the given property, and the values are an array with all items of the array that have the same value of that property.
{{#each-in (group-by "category" artists) as |category artists|}}
<h3>{{category}}</h3>
<ul>
{{#each artists as |artist|}}
<li>{{artist.name}}</li>
{{/each}}
</ul>
{{/each-in}}
keys
Returns an array of keys of given object.
{{#with (keys fields) as |labels|}}
<h3>This article contain {{labels.length}} fields</h3>
<ul>
{{#each labels as |label|}}
<li>{{label}}</li>
{{/each}}
</ul>
{{/with}}
pick
Receives an object and picks a specified path off of it to pass on. Intended for use with {{on}}
modifiers placed on form elements.
<input
...
{{on 'input' (pipe (pick 'target.value') this.onInput)}}
/>
It also supports an optional second argument to make common usage more ergonomic.
<input
...
{{on 'input' (pick 'target.value' this.onInput)}}
/>
values
Returns an array of values from the given object.
{{#with (values fields) as |data|}}
<h3>This article contain {{data.length}} fields</h3>
<ul>
{{#each data as |datum|}}
<li>{{datum}}</li>
{{/each}}
</ul>
{{/with}}
inc
Increments by 1
or step
.
{{inc numberOfPeople}}
{{inc 2 numberOfPeople}}
dec
Decrements by 1
or step
.
{{dec numberOfPeople}}
{{dec 2 numberOfPeople}}
String helpers were extracted to the ember-cli-string-helpers addon.
DockYard, Inc © 2016
Licensed under the MIT license
We're grateful to these wonderful contributors who've contributed to ember-composable-helpers
:
FAQs
Composable helpers for Ember
The npm package ember-composable-helpers receives a total of 17,998 weekly downloads. As such, ember-composable-helpers popularity was classified as popular.
We found that ember-composable-helpers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.