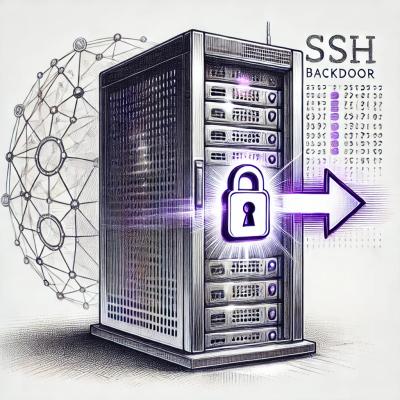
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
ember-decorators
Advanced tools
This addon adds decorator support to Ember, allowing you to DRY-up your code and write modern ES6 classes.
More details:
To use ember-decorators you must update Babel's configuration to allow usage of the decorator proposal.
Babel 6 has split out the various transforms to plugins, so we need to install the plugins for decorators:
yarn add --dev babel-plugin-transform-decorators-legacy
yarn add --dev babel-plugin-transform-class-properties # needed for ES6 class syntax
Or if you're using NPM:
npm install --save-dev babel-plugin-transform-decorators-legacy
npm install --save-dev babel-plugin-transform-class-properties
Now simply tell Babel to use these plugins:
// ember-cli-build.js
var app = new EmberApp({
babel: {
plugins: [
'transform-decorators-legacy',
'transform-class-properties'
]
}
});
As of Babel 5.1.0 the following should be all you need in your ember-cli application:
// Brocfile.js
var app = new EmberApp({
babel: {
optional: ['es7.decorators']
}
});
// ember-cli-build.js
var app = new EmberApp({
babel: {
optional: ['es7.decorators']
}
});
Add the following init
method to index.js
of your addon.
This should enable the decorators to work on the parent app/addon. Use version below that corresponds with your Babel 5.x addon or your Babel 6.x addon.
// Babel 5.x version
init: function(app) {
this._super.init && this._super.init.apply(this, arguments);
this.options = this.options || {};
this.options.babel = this.options.babel || {};
this.options.babel.optional = this.options.babel.optional || [];
if (this.options.babel.optional.indexOf('es7.decorators') === -1) {
this.options.babel.optional.push('es7.decorators');
}
}
// ~~ OR ~~
// Babel 6.x version
init: function(app) {
this._super.init && this._super.init.apply(this, arguments);
this.options = this.options || {};
this.options.babel = this.options.babel || {};
this.options.babel.plugins = this.options.babel.plugins || [];
if (this.options.babel.plugins.indexOf('transform-decorators-legacy') === -1) {
this.options.babel.plugins.push('transform-decorators-legacy');
}
if (this.options.babel.plugins.indexOf('transform-class-properties') === -1) {
this.options.babel.plugins.push('transform-class-properties');
}
}
In your application where you would normally have:
import Ember from 'ember';
export default Ember.Component.extend({
foo: Ember.inject.service(),
bar: Ember.computed('someKey', 'otherKey', function() {
var someKey = this.get('someKey');
var otherKey = this.get('otherKey');
// do stuff
}),
actions: {
handleClick() {
// do stuff
}
}
})
You replace it with this:
import Ember from 'ember'
import { action, computed } from 'ember-decorators/object';
import { service } from 'ember-decorators/service';
export default class MyComponent extends Ember.Component {
@service foo
@computed('someKey', 'otherKey')
bar(someKey, otherKey) {
// do stuff
}
@action
handleClick() {
// do stuff
}
}
The packages in ember-decorators
are setup to mirror Ember's javascript module
API. Decorators can be imported from the packages that they belong to:
import {
attr,
hasMany,
belongsTo
} from 'ember-decorators/data';
import {
controller
} from 'ember-decorators/controller';
import {
action,
computed,
observes
} from 'ember-decorators/object';
import {
alias,
or,
reads
} from 'ember-decorators/object/computed';
import {
on
} from 'ember-decorators/object/evented';
import {
service
} from 'ember-decorators/service';
See the API Documentation for detailed examples and documentation of the individual decorators.
Note: The @computed
decorator wraps ember-macro-helpers
which provides a lot of helpful features on top of standard computeds. It is
highly recommended that you read the documentation for that addon as well.
git clone <repository-url>
this repositorycd ember-decorators
npm install
bower install
ember serve
npm test
(Runs ember try:each
to test your addon against multiple Ember versions)ember test
ember test --server
ember build
For more information on using ember-cli, visit https://ember-cli.com/.
FAQs
Useful decorators for Ember applications.
The npm package ember-decorators receives a total of 54,023 weekly downloads. As such, ember-decorators popularity was classified as popular.
We found that ember-decorators demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.