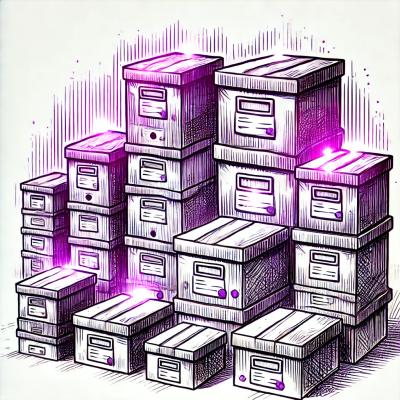
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
ember-intl
Advanced tools
This library provides Ember Handlebar helpers and a localization service. The service, and helpers, provide a way to format dates, numbers, strings messages, including pluralization.
Ember Intl is part of FormatJS, the docs can be found on the website:
ember install ember-intl@2.0.0-beta.10
(or ember install:addon ember-intl@2.0.0-beta.10
for ember-cli < v0.2.3)<script src="assets/intl/intl.min.js"></script>
<script src="assets/intl/locales/en-us.js"></script>
<script src="assets/intl/locales/fr-fr.js"></script>
<script src="assets/intl/locales/es-es.js"></script>
<!--
You can view the full list of CLDR locales which can be accessed from the `/assets/intl` folder
of your application. The CLDRs are automatically placed there at build time. Typically this folder
on your filesystem is ``<project>/dist/assets/intl`
Full list: https://github.com/yahoo/formatjs-extract-cldr-data/tree/master/data/main
-->
Translations are defined in /translations
, outside of app
in either JSON or YAML format. Example of /translations/en-us.yaml
:
# en-us
product:
info: '{product} will cost {price, number, USD} if ordered by {deadline, date, time}'
title: 'Hello world!'
html:
info: '<strong>{product}</strong> will cost <em>{price, number, USD}</em> if ordered by {deadline, date, time}'
Open, or create, app/routes/application.js
and in the beforeModel
hook set intl.locale
. Example:
// app/routes/application.js
export default Ember.Route.extend({
intl: Ember.inject.service(),
beforeModel() {
// define the app's runtime locale
// For example, here you would maybe do an API lookup to resolver
// which locale the user should be targeted and perhaps lazily
// load translations using XHR and calling intl's `addMessage`/`addMessages`
// method with the results of the XHR request
this.set('intl.locale', 'en-us');
}
});
config/environment.js
.// config/environment.js
return {
intl: {
defaultLocale: 'en-us' // default value
}
};
Formats numbers using Intl.NumberFormat
, and returns the formatted string value.
{{format-number num}}
{{format-number num format='EUR'}}
{{format-number num style='currency' currency='USD'}}
Or programmatically convert a number within any Ember Object.
// example
export default Ember.Component.extend({
intl: Ember.inject.service(),
computedNumber: Ember.computed('cost', function() {
return this.get('intl').formatNumber(this.get('cost')/*, optional options hash */);
})
});
List of supported format number options
Formats dates using Intl.DateTimeFormat
, and returns the formatted string value.
{{format-date now weekday='long' timeZone='UTC'}}
{{format-date now hour='numeric' minute='numeric' hour12=false}}
Or programmatically convert a date within any Ember Object.
// example
export default Ember.Component.extend({
intl: Ember.inject.service(),
computedNow: Ember.computed(function() {
return this.get('intl').formatDate(new Date()/*, optional options hash */);
})
});
List of supported format date options
This is just like the {{format-date}}
helper, except it will reference any string-named format
from formats.time
.
{{format-time now format='hhmmss'}}
{{format-time now hour='numeric' minute='numeric' hour12=false}}
Or programmatically convert a time within any Ember Object.
// example
export default Ember.Component.extend({
intl: Ember.inject.service(),
computedNow: Ember.computed(function() {
return this.get('intl').formatTime(new Date()/*, optional options hash */);
})
});
List of supported format date options
Formats dates relative to "now" using IntlRelativeFormat
, and returns the formatted string value.
export default Ember.Component.extend({
timestamp: Ember.computed(function() {
var date = new Date();
date.setDate(date.getDate() - 3);
return date;
})
});
{{format-relative timestamp}} -> 3 days ago
Or programmatically convert a relative time within any Ember Object.
// example
export default Ember.Component.extend({
intl: Ember.inject.service(),
yesterday: Ember.computed(function() {
var date = new Date();
return this.get('intl').formatRelative(date.setDate(date.getDate() - 1)/*, optional options hash */);
})
});
List of supported format date options
Formats ICU Message strings with the given values supplied as the hash arguments.
You have {numPhotos, plural,
=0 {no photos.}
=1 {one photo.}
other {# photos.}}
{{format-message (intl-get 'product.info')
product='Apple watch'
price=200
deadline=yesterday}}
{{format-message boundProperty
name='Jason'
numPhotos=num
takenDate=yesterday}}
Or programmatically convert a message within any Ember Object.
export default Ember.Component.extend({
intl: Ember.inject.service(),
yesterday: Ember.computed(function() {
var now = new Date();
return this.get('intl').formatMessage('Hello {name}', { name: 'Jason' });
})
});
This delegates to the {{format-message}}
helper, but will first HTML-escape all of the hash argument values. This allows the message
string to contain HTML and it will be considered safe since it's part of the template and not user-supplied data.
{{format-html-message (intl-get 'product.html.info')
product='Apple watch'
price=200
deadline=yesterday}}
{{format-html-message '<strong>{numPhotos}</strong>'
numPhotos=(format-number num)}}
Utility helper for returning the value, or eventual value, based on a translation key. Should only ever be used as a subexpression, never as a standalone helper.
{{format-message (intl-get 'product.info')
product='Apple watch'
price=200
deadline=yesterday}}
Will return the message from the current locale, or locale explicitly passed as an argument, message object.
product:
info: '{product} will cost {price, number, EUR} if ordered by {deadline, date, time}'
// config/environment.js
module.exports = function() {
return {
intl: {
disablePolyfill: true
}
};
};
// Brocfile.js
var app = new EmberApp({
outputPaths: {
intl: '/assets/intl' // default
}
});
date value is not finite in DateTimeFormat.format()
Browser vendors implement date/time parsing differently. For example, the following will parse correctly in Chrome but fail in Firefox: new Intl.DateTimeFormat().format('2015-04-21 20:47:31 GMT');
The solution is the ensure that the value you are passing in is in a format which is valid for the Date
constructor. This library currently does not try and normalize date strings outside of what the browser already implements.
locale
argument to explicitly pass/override the application localeformat
argument which you pass in a key corresponding to a format configuration in app/formats.js
If using the intl helpers within a components or views that is unit tested, needs
the service, helper, and formatter into the unit test.
In the setup hook of moduleFor
/moduleForComponent
you'll want to also invoke registerIntl(container);
-- which is a utility function to setup the injection logic on the unit test container.
NOTE: Add the following above all script tags in tests/index.html
<script src="assets/intl/intl.complete.js"></script>
This is to shim your test runner if running within phantomjs, or any browser which does not natively support the Intl API.
/**
* unit test for testing index view which contains the helpers: `format-message` and `intl-get`
*
* unit/views/index-test.js
*/
import Ember from 'ember';
import { registerIntl } from '../../../initializers/ember-intl';
import {
moduleFor,
test
} from 'ember-qunit';
moduleFor('view:index', 'IndexView', {
needs: [
'template:index',
'ember-intl@adapter:-intl-adapter',
'service:intl',
'helper:intl-get',
'ember-intl@formatter:format-message',
'translation:en-us',
'translation:es-es'
],
setup: function () {
// depending on your test library, container will be hanging off `this`
// or otherwise passed in as the first argument
var container = this.container || arguments[0];
// injects the service on to all logical factory types
registerIntl(container);
// set the initial intl service locale to `en-us`
var intl = container.lookup('service:intl');
intl.set('locale', 'en-us');
}
});
test('index renders', function () {
expect(2);
var view = this.subject({
context: Ember.Object.create({
firstName: 'Tom'
})
});
var intl = view.get('intl');
Ember.run(view, 'appendTo', '#qunit-fixture');
equal(view.$().text().trim(), "hello Tom");
Ember.run(function () { intl.set('locale', 'es-es'); });
equal(view.$().text().trim(), "hola Tom");
Ember.run(view, 'destroy');
});
ember server
ember test
ember test --server
FAQs
Internationalization for Ember projects
The npm package ember-intl receives a total of 32,188 weekly downloads. As such, ember-intl popularity was classified as popular.
We found that ember-intl demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.