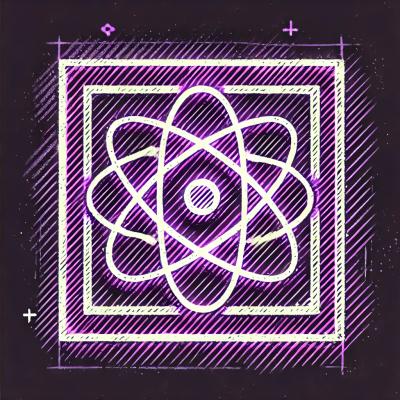
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
ember-truth-helpers
Advanced tools
The ember-truth-helpers package provides a set of template helpers for common logical operations in Ember.js applications. These helpers allow you to perform logical comparisons and operations directly within your templates, making your code more readable and reducing the need for complex computed properties or controller logic.
and
The `and` helper allows you to check if multiple conditions are true. If all conditions are true, the block inside the `if` statement will be executed.
{{#if (and condition1 condition2)}}
Both conditions are true
{{/if}}
or
The `or` helper allows you to check if at least one of the conditions is true. If any condition is true, the block inside the `if` statement will be executed.
{{#if (or condition1 condition2)}}
At least one condition is true
{{/if}}
not
The `not` helper allows you to check if a condition is false. If the condition is false, the block inside the `if` statement will be executed.
{{#if (not condition)}}
Condition is false
{{/if}}
eq
The `eq` helper allows you to check if two values are equal. If the values are equal, the block inside the `if` statement will be executed.
{{#if (eq value1 value2)}}
Values are equal
{{/if}}
gt
The `gt` helper allows you to check if one value is greater than another. If `value1` is greater than `value2`, the block inside the `if` statement will be executed.
{{#if (gt value1 value2)}}
value1 is greater than value2
{{/if}}
The ember-composable-helpers package provides a collection of composable template helpers for Ember.js. It includes logical helpers similar to ember-truth-helpers, such as `and`, `or`, and `not`, but also offers a wider range of utilities for working with arrays, objects, and functions. This makes it a more comprehensive solution for template logic.
The ember-math-helpers package provides a set of template helpers for performing mathematical operations in Ember.js templates. While it focuses on math operations rather than logical comparisons, it can be used in conjunction with ember-truth-helpers to handle more complex template logic involving both math and logical operations.
HTMLBars template helpers for additional truth logic in if
and unless
statements.
ember install ember-truth-helpers
Helper | JavaScript | HTMLBars | Variable argument count allowed |
---|---|---|---|
eq | if (a === b) | {{if (eq a b)}} | No |
not-eq | if (a !== b) | {{if (not-eq a b)}} | No |
not | if (!a) | {{if (not a)}} | Yes |
and | if (a && b) | {{if (and a b)}} | Yes |
or | if (a || b) | {{if (or a b)}} | Yes |
xor | if (a !== b) | {{if (xor a b)}} | No |
gt | if (a > b) | {{if (gt a b)}} | No |
gte | if (a >= b) | {{if (gte a b)}} | No |
lt | if (a < b) | {{if (lt a b)}} | No |
lte | if (a <= b) | {{if (lte a b)}} | No |
is-array | if (Ember.isArray(a)) | {{if (is-array a)}} | Yes |
is-empty | if (Ember.isEmpty(a)) | {{if (is-empty a)}} | No |
is-equal | if (Ember.isEqual(a, b)) | {{if (is-equal a b)}} | No |
is-equal
uses Ember.isEqual
helper to evaluate equality of two values.
eq
should be sufficient for most applications. is-equal
is necessary when trying to compare a complex object to
a primitive value.
ember-truth-helpers
is a glint enabled addon. Add this to your
types/global.d.ts
file:
import '@glint/environment-ember-loose';
import type EmberTruthRegistry from 'ember-truth-helpers/template-registry';
declare module '@glint/environment-ember-loose/registry' {
export default interface Registry extends EmberTruthRegistry, /* other addon registries */ {
// local entries
}
}
For the entire guide, please refer to Using Addons section on the glint handbook.
Types are made available through package.json exports
field. In order for TS
to recognize this (beginning from TS 4.7), you must set
moduleResolution
to node16
or nodenext
.
For usage in gts
or gjs
files, all helpers are exported from the index:
import { or } from 'ember-truth-helpers';
<template>
{{#if (or @admin @user)}}
Admin Controls are going here
{{/if}}
</template>
See the Contributing guide for details.
This project is licensed under the MIT License.
v4.0.3 (2023-09-11)
FAQs
Ember Truth Helpers
The npm package ember-truth-helpers receives a total of 88,617 weekly downloads. As such, ember-truth-helpers popularity was classified as popular.
We found that ember-truth-helpers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.