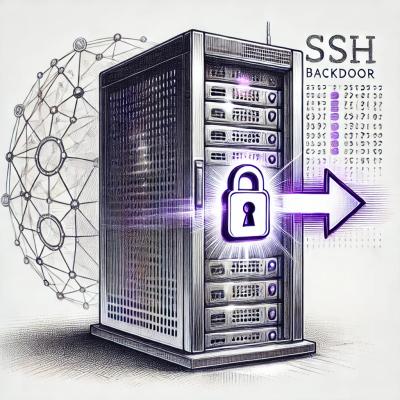
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
A lightweight event emitter for clients and servers.
E$ can be used as a standalone constructor, or to extend prototypical objects.
var emoneyStandalone = E$({
handleE$: function( e ) { ... }
});
var emoneyExtended = (function() {
function E$ishConstructor() {
E$.construct( this );
}
E$ishConstructor.prototype = E$.create({
handleE$: function( e ) { ... }
});
return new E$ishConstructor();
}());
E$ provides a clean way to interface with object instances.
emoneyExtended
.$when( 'loading' , function( e , pct ) {
console.log( 'loading... (' + pct + '%)' );
})
.$when( 'ready' , function( e ) {
console.log( 'ready!' );
})
.$when( 'error' , function( e , err ) {
console.error( err.stack );
});
E$ instances can communicate via the
handleE$
method.
emoneyExtended.$watch( emoneyStandalone );
// when emoneyStandalone emits an event
emoneyStandalone.$emit( 'gnarly' , [ 'something' , { rad: true }]);
// emoneyExtended.handleE$ is executed with arguments:
// [ event , something , { rad: true }]
creates a new object that extends the E$ prototype.
E$ishConstructor.prototype = E$.create({
method1: function() { ... },
method2: function() { ... },
handleE$: function( e ) { ... }
});
defines required properties for an E$ instance.
function E$ishConstructor() {
E$.construct( this );
// ...
}
determines whether subject is E$-ish.
var emoney = E$({ gnarly: true });
var emoneyIsh = new E$ishConstructor();
var somethingElse = new SomethingElse();
emoney instanceof E$; // true
E$.is( emoney ); // true
emoneyIsh instanceof E$; // false
E$.is( emoneyIsh ); // true
E$.is( somethingElse ); // false
All public methods can be chained.
adds an event listener.
Parameter | Type | Description | Required |
---|---|---|---|
events | String Array | The event(s) to be handled. | yes |
args | Variant Array | The argument(s) to be bound to the event handler. | no |
handler | Function E$ | The event handler. If E$.is( handler ) == true , the event will be bound to instance.handleE$ .If handler is falsy, the event will be bound to emoney.handleE$ . | no |
// basic use
emoney.$when( 'gnarly' , function( e ) { ... });
// bind an argument to multiple events
emoney.$when([ 'gnarly' , 'rad' ] , 'arg' , function( e , arg ) { ... });
// bind multiple arguments to the wildcard event
emoney.$when( '*' , [ 'arg1' , 'arg2' ] , function( e , arg1 , arg2 ) { ... });
adds an event listener that is removed after the first time it is invoked.
Parameter | Type | Description | Required |
---|---|---|---|
events | String Array | The event(s) to be handled. | yes |
args | Variant Array | The argument(s) to be bound to the event handler. | no |
handler | Function E$ | the event handler | no |
// basic use
emoney.$once( 'gnarly' , function( e ) { ... });
// bind an argument to multiple events
emoney.$once([ 'gnarly' , 'rad' ] , 'arg' , function( e , arg ) { ... });
// bind multiple arguments to the wildcard event
emoney.$once( '*' , [ 'arg1' , 'arg2' ] , function( e , arg1 , arg2 ) { ... });
emits an event.
Parameter | Type | Description | Required |
---|---|---|---|
events | String Array | the event(s) to be emitted | yes |
args | Variant Array | the argument(s) to be passed to the event handler | no |
callback | Function | a function to be executed at the end of the event chain (see event behavior) | no |
// basic use
emoney.$emit( 'gnarly' , function( e ) { ... });
// pass an argument to multiple event handlers
emoney.$emit([ 'gnarly' , 'rad' ] , 'arg' , function( e ) { ... });
// pass multiple arguments to an event handler
emoney.$emit( 'gnarly' , [ 'arg1' , 'arg2' ] , function( e ) { ... });
removes an event listener.
Parameter | Type | Description | Required |
---|---|---|---|
events | String Array null | The event(s) to be removed. | yes |
wildcard | Boolean | A boolean value denoting whether handlers bound to the wildcard event should be removed. | no |
handler | Function E$ | the event handler | no |
// remove any gnarly listeners bound to handlerFunc
emoney.$dispel( 'gnarly' , handlerFunc );
// remove all gnarly or rad listeners bound to any handler
emoney.$dispel([ 'gnarly' , 'rad' ]);
// remove all listeners bound to handlerFunc except wildcard listeners
emoney.$dispel( null , handlerFunc );
// remove all listeners bound to handlerFunc
emoney.$dispel( null , true , handlerFunc );
// remove all listeners
emoney.$dispel( null , true );
starts listening to an E$ instance.
emitter
events will be handled bylistener.handleE$
.
Parameter | Type | Description | Required |
---|---|---|---|
emitters | E$ Array | The target E$ instance(s). | yes |
// watch a single emitter
listener.$watch( emitter1 );
// watch multiple emitters
listener.$watch([ emitter1 , emitter2 ]);
stops listening to an E$ instance.
Parameter | Type | Description | Required |
---|---|---|---|
emitters | E$ Array | The target E$ instance(s). | yes |
// ignore a single emitter
listener.$ignore( emitter1 );
// ignore multiple emitters
listener.$ignore([ emitter1 , emitter2 ]);
Property | Type | Default | Description |
---|---|---|---|
target | Object | n/a | The event target. |
type | String | n/a | The event type. |
defaultPrevented | Boolean | false | A flag denoting whether default was prevented. |
cancelBubble | Boolean | false | A flag denoting whether propagation was stopped. |
timeStamp | Float | n/a | The time at which the event was first triggered. |
prevents the $emit callback from being executed.
emoney
.$when( 'gnarly' , function( e ) {
e.preventDefault();
console.log( 'handler1' );
})
.$when( 'gnarly' , function( e ) {
console.log( 'handler2' );
})
.$emit( 'gnarly' , function( e ) {
console.log( 'callback' );
});
/*
** > 'handler1'
** > 'handler2'
*/
stops execution of the event chain and executes the emit callback.
emoney
.$when( 'gnarly' , function( e ) {
e.stopPropagation();
console.log( 'handler1' );
})
.$when( 'gnarly' , function( e ) {
console.log( 'handler2' );
})
.$emit( 'gnarly' , function( e ) {
console.log( 'callback' );
});
/*
** > 'handler1'
** > 'callback'
*/
Build configs can be found in Gruntfile.js
Builds dev and prod releases, then runs tests.
grunt
Builds dev release and then runs tests.
grunt test
Builds dev release, runs tests, then watches source files for changes.
grunt debug
FAQs
A lightweight, isomorphic event emitter
The npm package emoney receives a total of 0 weekly downloads. As such, emoney popularity was classified as not popular.
We found that emoney demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.