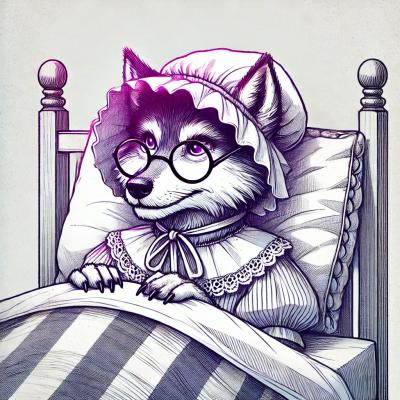
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The encodeurl npm package is used to encode a URL to a percent-encoded form, excluding already-encoded sequences. This is particularly useful when you need to encode a URL in a way that is safe to include in HTTP headers and HTML links without double-encoding existing percent-encoded characters.
Percent-encoding URL
This feature allows you to encode a URL into a format that can be safely transmitted over the internet. The code sample demonstrates how to encode a URL with query parameters, ensuring that spaces and other special characters are properly percent-encoded.
const encodeUrl = require('encodeurl');
const encodedUrl = encodeUrl('https://example.com/foo?user=bar+baz');
console.log(encodedUrl);
The querystring package provides utilities for parsing and formatting URL query strings. It can be used to percent-encode a query string, but unlike encodeurl, it is specifically designed for handling the query string part of a URL and not the entire URL.
Similar to querystring, the qs package allows for parsing and stringifying query strings with more advanced features like nested objects. It also handles percent-encoding but is focused on the query string component rather than full URLs.
The urlencode package is another alternative for percent-encoding URLs and query strings. It offers similar functionality to encodeurl but with a slightly different API and additional options for encoding.
Encode a URL to a percent-encoded form, excluding already-encoded sequences
$ npm install encodeurl
var encodeUrl = require('encodeurl')
Encode a URL to a percent-encoded form, excluding already-encoded sequences.
This function will take an already-encoded URL and encode all the non-URL
code points (as UTF-8 byte sequences). This function will not encode the
"%" character unless it is not part of a valid sequence (%20
will be
left as-is, but %foo
will be encoded as %25foo
).
This encode is meant to be "safe" and does not throw errors. It will try as hard as it can to properly encode the given URL, including replacing any raw, unpaired surrogate pairs with the Unicode replacement character prior to encoding.
This function is similar to the intrinsic function encodeURI
, except it
will not encode the %
character if that is part of a valid sequence, will
not encode [
and ]
(for IPv6 hostnames) and will replace raw, unpaired
surrogate pairs with the Unicode replacement character (instead of throwing).
var encodeUrl = require('encodeurl')
var escapeHtml = require('escape-html')
http.createServer(function onRequest (req, res) {
// get encoded form of inbound url
var url = encodeUrl(req.url)
// create html message
var body = '<p>Location ' + escapeHtml(url) + ' not found</p>'
// send a 404
res.statusCode = 404
res.setHeader('Content-Type', 'text/html; charset=UTF-8')
res.setHeader('Content-Length', String(Buffer.byteLength(body, 'utf-8')))
res.end(body, 'utf-8')
})
var encodeUrl = require('encodeurl')
var escapeHtml = require('escape-html')
var url = require('url')
http.createServer(function onRequest (req, res) {
// parse inbound url
var href = url.parse(req)
// set new host for redirect
href.host = 'localhost'
href.protocol = 'https:'
href.slashes = true
// create location header
var location = encodeUrl(url.format(href))
// create html message
var body = '<p>Redirecting to new site: ' + escapeHtml(location) + '</p>'
// send a 301
res.statusCode = 301
res.setHeader('Content-Type', 'text/html; charset=UTF-8')
res.setHeader('Content-Length', String(Buffer.byteLength(body, 'utf-8')))
res.setHeader('Location', location)
res.end(body, 'utf-8')
})
$ npm test
$ npm run lint
FAQs
Encode a URL to a percent-encoded form, excluding already-encoded sequences
The npm package encodeurl receives a total of 32,720,677 weekly downloads. As such, encodeurl popularity was classified as popular.
We found that encodeurl demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.