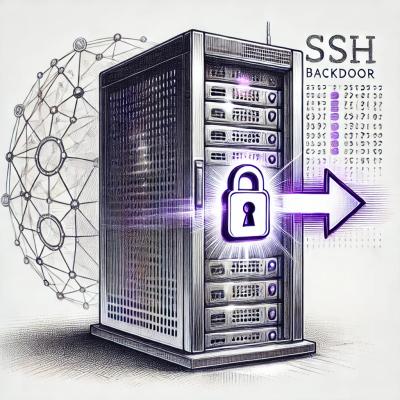
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Functional programming helpers for Enonic XP. This library provides fp-ts wrappers around the Enonic-interfaces provided by enonic-types, which again wraps the official standard libraries (in jars).
We recommend using this library together with the
xp-codegen-plugin Gradle plugin. xp-codegen-plugin will create TypeScript
interfaces
for your content-types. Those interfaces will be very useful together with this library.
Most functions in this library wraps the result in an IOEither<EnonicError, A>.
This gives us two things:
fold
pipe
the results from one operation into the next using chain
(or map
). Chain expects another
IOEither<EnonicError, A>
to be returned. When the first left<EnonicError>
is returned, the pipe will short
circuit to the error case in fold
.This style of programming encourages us to write re-usable functions that we can compose together using pipe
.
In this example we have a service that returns Article content – that has a key
as id – as json. Or if something goes
wrong, we return an Internal Server Error instead.
import {fold} from "fp-ts/IOEither";
import {pipe} from "fp-ts/pipeable";
import {get as getContent} from "enonic-fp/content";
import {Article} from "../../site/content-types/article/article"; // 1
import {internalServerError, ok} from "enonic-fp/controller";
export function get(req: XP.Request): XP.Response { // 2
const program = pipe( // 3
getContent<Article>(req.params.key!), // 4
fold( // 5
internalServerError,
ok
)
);
return program(); // 6
}
interface Article { ... }
generated by
xp-codegen-plugin.Request
and Response
to control the shape of our controller.pipe
function from fp-ts to pipe the result of one function into the next one.get
function from content
– here renamed getContent
so it won't collide with the get
function in
the controller – to return some content where the type is IOEither<EnonicError, Content<Article>>
.IOEither
. This is done with
fold(handleError, handleSuccess)
. enonic-fp comes with a set of functions that creates an IO<Response>
with
the data. There are pre-configured functions that can be used in fold
for some of the most common http status
numbers. Like ok()
and internalServerError()
.program
of type IO<Response>
, but we have not yet performed a single
side effect. It's time to perform those side effects, so we run the IO
by calling it, and return the Response
we
get back.In this example we delete come content by key
. We are first doing this on the draft
branch. And then we publish
it
to the master
branch.
We will return a http error based on the type of error that happened (trough a lookup in the errorsKeyToStatus
map).
Or we return a http status 204
, indicating success.
import {chain, fold} from "fp-ts/IOEither";
import {pipe} from "fp-ts/pipeable";
import {publish, remove} from "enonic-fp/content";
import {run} from "enonic-fp/context";
import {errorResponse, noContent} from "enonic-fp/controller";
function del(req: XP.Request): XP.Response {
const program = pipe(
runOnBranchDraft(
remove(req.params.key!) // 1
),
chain(() => publish(req.params.key!)), // 2
fold( // 3
errorResponse({ req, i18nPrefix: "articleErrors" }), // 4
noContent // 5
)
);
return program();
}
export {del as delete}; // 6
const runOnBranchDraft = run({ branch: 'draft' }); // 7
remove
function with the key
to delete some content. We want to do this on the draft branch, so we
wrap the call in the runInDraftContext
function that is defined below.
Remove returns IOEither<EnonicError, void>
. If the content didn't exist, it will return an EnonicError
with of
type "https://problem.item.no/xp/not-found", that can be handled in the
fold()
.publish()
function in
enonic-fp has an overload that only takes the key
as a string
and defaults to publish from draft to
master.Response
we call fold
, where we handle the error and success cases, and return IO<Response>
.errorResponse()
function use the HttpError.status
field to know which http status number to use on the
Response
. It can optionally take the Request
and a i18nPrefix
as parameters.
Request
adds the HttpError.instance
on the return object, and it will check if req.mode !== 'live'
,
and if yes, return more details about the error (this is to prevent exploits based on the error messages).i18nPrefix
is detailed the i18n for error messages chapter.delete
function.ContextLib.run
. It returns a new function – here assigned to the constant
runOnBranchDraft
– that takes an IO
as parameter (which all the wrapped functions already return as IOEither
).In this example we do three queries. First we look up an article by key
, then we search for comments related to that
article based on the articles key. And then we get a list of open positions in the company, that we want to display on
the web page.
import {sequenceT} from "fp-ts/Apply";
import {Json} from "fp-ts/Either";
import {chain, fold, ioEither, IOEither, map} from "fp-ts/IOEither";
import {pipe} from "fp-ts/pipeable";
import {Content, QueryResponse} from "/lib/xp/content";
import {getRenderer} from "enonic-fp/thymeleaf";
import {EnonicError} from "enonic-fp/errors";
import {get as getContent, query} from "enonic-fp/content";
import {bodyAsJson, request} from "enonic-fp/http";
import {Article} from "../../site/content-types/article/article";
import {Comment} from "../../site/content-types/comment/comment";
import {ok, unsafeRenderErrorPage} from "enonic-fp/controller";
import {tupled} from "fp-ts/function";
const view = resolve('./article.html');
const errorView = resolve('../../templates/error.html');
const renderer = getRenderer<ThymeleafParams>(view); // 1
export function get(req: XP.Request): XP.Response {
const articleId = req.params.key!;
return pipe(
sequenceT(ioEither)( // 2
getContent<Article>(articleId),
getCommentsByArticleKey(articleId),
getOpenPositionsOverHttp()
),
map(tupled(createThymeleafParams)), // 3
chain(renderer), // 4
fold(
unsafeRenderErrorPage(errorView), // 5
ok
)
)();
}
function getCommentsByArticleKey(articleId: string)
: IOEither<EnonicError, QueryResponse<Comment>> {
return query<Comment>({
contentTypes: ["com.example:comment"],
count: 100,
query: `data.articleId = '${articleId}'`
});
}
function getOpenPositionsOverHttp(): IOEither<EnonicError, Json> {
return pipe(
request("https://example.com/api/open-positions"), // 6
chain(bodyAsJson)
);
}
function createThymeleafParams( // 7
article: Content<Article>,
comments: QueryResponse<Comment>,
openPositions: Json
): ThymeleafParams {
return {
id: article._id,
data: article.data,
comments: comments.hits,
openPositions
};
}
interface ThymeleafParams {
readonly id: string;
readonly data: Article;
readonly comments: ReadonlyArray<Comment>;
readonly openPositions: Json
}
getRenderer()
is a curried version of ThymeleafLib.render()
. It takes ThymeleafParams
(defined below) as a
type parameter and the view
as a parameter, and returns a function with this signature:(params: ThymeleafParams) => IOEither<EnonicError, string>
, where the string is the finished rendered page.sequenceT
taking the three IOEither<EnonicError, A>
as input, and getting an IOEither
with the results
in a tuple (IOEither<EnonicError, [Content<Article>, QueryResponse<Comment>, Json]>
). The first two are queries in
Enonic, and the last one is over http.map
over the tuple, using createThymeleafParams()
. But first we use the tupled
function on
createThymeleafParams()
to give us a new version of createThymeleafParams
that takes the parameters as a
tuple, instead of as individual arguments. A good rule of thumb is to always use tupled
together with sequenceT
!render()
function in a chain()
, since it returns an IOEither<EnonicError, string>
.pipe
has returned a Left<EnonicError>
, we need to handle the EnonicError
. In
this case we want to render an error page. The unsafeRenderErrorPage()
takes the errorView
(html page) as
parameter, which should be a template for EnonicError
. If the templating succeeds, an IO<Response>
is created
with the page as the body
, and with the http status from the EnonicError
. But if it fails, we just need to let
it fail completely and handled by Enonic XP, because we don't want an infinite loop of failing templating.HttpLib.request
, which only takes the url as parameter. We then pipe
it into the
bodyAsJson
function that parses the json in the Request.body
and returns an EnonicError
if it fails.createThymeleafParams
function gathers all the data and creates one new object that the Thymeleaf-renderer
will take as input.There is support for adding internationalization for error-messages. This is done, when you generate the Response
using the errorResponse({ req: Request, i18nPrefix: string})
method.
The i18n-key to use to look up the message has the following shape: ${i18nPrefix}.title.${typeString}
where
typeString
is the last section of EnonicError.type
. To support every error in enonic-fp, typeString
can only be
one of these:
If your i18nPrefix
is e.g "getArticleError"
, then you can add the following to your phrases.properties to get
customized error messages for different endpoints.
getArticleError.title.bad-request-error=Problems with client parameters
getArticleError.title.not-found=No Article Found
getArticleError.title.internal-server-error=Can not retreive article.
getArticleError.title.missing-id-provider=Missing ID Provider.
getArticleError.title.publish-error=Unable to publish the article.
getArticleError.title.unpublish-error=Unable to unpublish the article
getArticleError.title.bad-gateway=Unable to retreive open positions.
We recommend adding the following (but translated) keys to your phrases.properties file, as they will provide backup error messages for all instances where custom error messages have not been specified.
errors.title.bad-request-error=Bad request error
errors.title.not-found=Not found
errors.title.internal-server-error=Internal Server Error
errors.title.missing-id-provider=Missing ID Provider.
errors.title.publish-error=Unable to publish data
errors.title.unpublish-error=Unable to unpublish data
errors.title.bad-gateway=Bad gateway
Alternatively you could use the status number as the typeString
-part of the key. But this will not be able to separate
different errors with the same status
(e.g both internal-server-error, missing-id-provider and publish-error
has status = 500).
errors.title.400=Bad request error
errors.title.404=Not found
errors.title.500=Internal Server Error
errors.title.502=Bad gateway
npm run build
FAQs
Functional programming helpers for Enonic XP
We found that enonic-fp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.