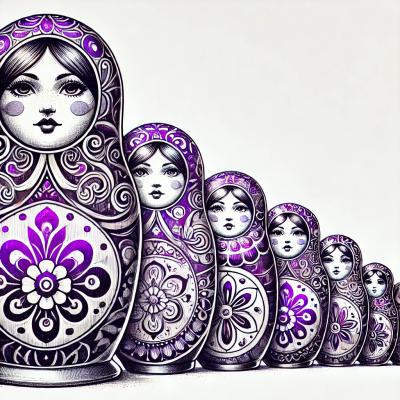
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
The envalid npm package is a library for validating and accessing environment variables in Node.js applications. It helps ensure that your environment variables are present and correctly formatted, reducing the risk of runtime errors due to misconfiguration.
Environment Variable Validation
This feature allows you to define and validate environment variables with specific types and constraints. The `cleanEnv` function ensures that the environment variables are present and correctly formatted, providing default values if necessary.
const envalid = require('envalid');
const { str, num, bool } = envalid;
const env = envalid.cleanEnv(process.env, {
NODE_ENV: str({ choices: ['development', 'production', 'test'] }),
PORT: num({ default: 3000 }),
DEBUG: bool({ default: false })
});
console.log(env.NODE_ENV); // 'development', 'production', or 'test'
console.log(env.PORT); // 3000 if not specified
console.log(env.DEBUG); // false if not specified
Custom Validators
Envalid allows you to create custom validators for environment variables using the `makeValidator` function. This is useful for more complex validation logic that isn't covered by the built-in validators.
const envalid = require('envalid');
const { makeValidator } = envalid;
const myCustomValidator = makeValidator(x => {
if (x !== 'foo' && x !== 'bar') throw new Error('Must be either foo or bar');
return x;
});
const env = envalid.cleanEnv(process.env, {
MY_VAR: myCustomValidator()
});
console.log(env.MY_VAR); // 'foo' or 'bar'
Type Coercion
Envalid automatically coerces environment variables to the specified types. This ensures that your application receives the correct data types, reducing the need for manual type conversion.
const envalid = require('envalid');
const { str, num, bool } = envalid;
const env = envalid.cleanEnv(process.env, {
MY_STRING: str(),
MY_NUMBER: num(),
MY_BOOLEAN: bool()
});
console.log(typeof env.MY_STRING); // 'string'
console.log(typeof env.MY_NUMBER); // 'number'
console.log(typeof env.MY_BOOLEAN); // 'boolean'
Dotenv is a zero-dependency module that loads environment variables from a .env file into process.env. While it doesn't provide validation or type coercion like envalid, it is widely used for managing environment variables in Node.js applications.
Joi is a powerful schema description language and data validator for JavaScript. It can be used to validate environment variables, but it requires more setup compared to envalid. Joi is more flexible and can be used for validating other types of data as well.
Convict is a configuration management tool for Node.js that allows you to define a schema for your configuration, including environment variables. It provides validation and default values, similar to envalid, but also supports nested configurations and more complex use cases.
Envalid is a small library for validating and accessing environment variables in Node.js (v8.12 or later) programs, aiming to:
envalid.cleanEnv(environment, validators, options)
cleanEnv()
returns a sanitized, immutable environment object, and accepts three
positional arguments:
environment
- An object containing your env vars (eg. process.env
)validators
- An object that specifies the format of required vars.options
- An (optional) object, which supports the following keys:
strict
- (default: false
) Enable more rigorous behavior. See "Strict Mode" belowreporter
- Pass in a function to override the default error handling and
console output. See src/reporter.js
for the default implementation.transformer
- A function used to transform the cleaned environment object
before it is returned from cleanEnv
dotEnvPath
- (default: '.env'
) Path to the file that is parsed by
dotenv to
optionally load more env vars at runtime. Pass null
if you want
to skip dotenv
processing entirely and only load from process.env
.By default, cleanEnv()
will log an error message and exit if any required
env vars are missing or invalid.
const envalid = require('envalid')
const { str, email, json } = envalid
const env = envalid.cleanEnv(process.env, {
API_KEY: str(),
ADMIN_EMAIL: email({ default: 'admin@example.com' }),
EMAIL_CONFIG_JSON: json({ desc: 'Additional email parameters' })
})
// Read an environment variable, which is validated and cleaned during
// and/or filtering that you specified with cleanEnv().
env.ADMIN_EMAIL // -> 'admin@example.com'
// Envalid parses NODE_ENV automatically, and provides the following
// shortcut (boolean) properties for checking its value:
env.isProduction // true if NODE_ENV === 'production'
env.isTest // true if NODE_ENV === 'test'
env.isDev // true if NODE_ENV === 'development'
For an example you can play with, clone this repo and see the example/
directory.
Node's process.env
only stores strings, but sometimes you want to retrieve other types
(booleans, numbers), or validate that an env var is in a specific format (JSON,
url, email address). To these ends, the following validation functions are available:
str()
- Passes string values through, will ensure an value is present unless a
default
value is given. Note that an empty string is considered a valid value -
if this is undesirable you can easily create your own validator (see below)bool()
- Parses env var strings "0", "1", "true", "false", "t", "f"
into booleansnum()
- Parses an env var (eg. "42", "0.23", "1e5"
) into a Numberemail()
- Ensures an env var is an email addresshost()
- Ensures an env var is either a domain name or an ip address (v4 or v6)port()
- Ensures an env var is a TCP port (1-65535)url()
- Ensures an env var is a url with a protocol and hostnamejson()
- Parses an env var with JSON.parse
Each validation function accepts an (optional) object with the following attributes:
choices
- An Array that lists the admissable parsed values for the env var.default
- A fallback value, which will be used if the env var wasn't specified.
Providing a default effectively makes the env var optional.devDefault
- A fallback value to use only when NODE_ENV
is not 'production'
. This is handy
for env vars that are required for production environments, but optional
for development and testing.desc
- A string that describes the env var.example
- An example value for the env var.docs
- A url that leads to more detailed documentation about the env var.You can easily create your own validator functions with envalid.makeValidator()
. It takes
a function as its only parameter, and should either return a cleaned value, or throw if the
input is unacceptable:
const { makeValidator, cleanEnv } = require('envalid')
const twochars = makeValidator(x => {
if (/^[A-Za-z]{2}$/.test(x)) return x.toUpperCase()
else throw new Error('Expected two letters')
})
const env = cleanEnv(process.env, {
INITIALS: twochars()
});
You can, and should, also provide a type
with your validator. This can be exposed by tools
to help other developers better understand you configuration options.
To add it, pass a string with the name as the second argument to makeValidator
.
const { makeValidator } = require('envalid')
const twochars = makeValidator(x => {
if (/^[A-Za-z]{2}$/.test(x)) return x.toUpperCase()
else throw new Error('Expected two letters')
}, 'twochars')
By default, if any required environment variables are missing or have invalid
values, envalid will log a message and call process.exit(1)
. You can override
this behavior by passing in your own function as options.reporter
. For example:
const env = cleanEnv(process.env, myValidators, {
reporter: ({ errors, env }) => {
emailSiteAdmins('Invalid env vars: ' + Object.keys(errors))
}
})
Additionally, envalid exposes EnvError
and EnvMissingError
, which can be checked in case specific error handling is desired:
const env = cleanEnv(process.env, myValidators, {
reporter: ({ errors, env }) => {
errors.forEach(err => {
if (err instanceof envalid.EnvError) {
...
} else if (err instanceof envalid.EnvMissingError) {
...
} else {
...
}
});
}
})
By passing the { strict: true }
option, envalid gives you extra tight guarantees
about the cleaned env object:
validators
..env
File SupportEnvalid wraps the very handy dotenv package,
so if you have a .env
file in your project, envalid will read and validate the
env vars from that file as well.
When using Envalid within React Native the dotenv
integration will not work, and the dotEnvPath
option will be ignored.
Envalid can be used within React Native with a custom reporter.
Instead of dotenv
react-native-config can be used to read the configuration.
Example:
const reactNativeConfig = require('react-native-config')
const rawConfig = reactNativeConfig.default
const validatedConfig = envalid.cleanEnv(
rawConfig,
{
// validators
},
{
dotEnvPath: null,
reporter: ({ errors = {}, env = {} }) => {
// handle errors
},
},
)
When using Envalid within browsers the dotenv
integration will not work, and the dotEnvPath
option will be ignored.
A helper function called testOnly
is available, in case you need an default env var only when
NODE_ENV=test
. It should be used along with devDefault
, for example:
const env = cleanEnv(process.env, {
SOME_VAR: envalid.str({devDefault: testOnly('myTestValue')})
})
For more context see this issue.
FAQs
Validation for your environment variables
The npm package envalid receives a total of 218,592 weekly downloads. As such, envalid popularity was classified as popular.
We found that envalid demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.