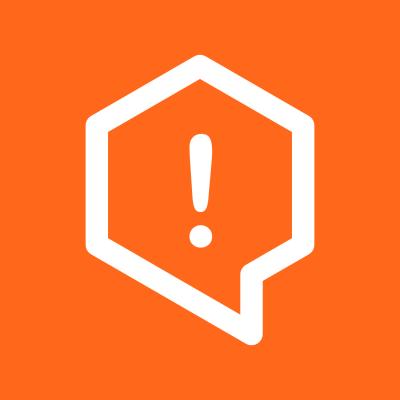
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
error-handler-module
Advanced tools
This module provides handling error methods for different systems.
This module provides a way to handle error in an express app with a few methods that creates error and an express error middleware handleHttpError
.
npm install --save error-handler-module
We have setup some errors that we use often use in our projects and we store them in an object which is this:
const CustomErrorTypes = {
BAD_REQUEST: 'bad_request',
FORBIDDEN: 'forbidden',
NOT_FOUND: 'not_found',
OAS_VALIDATOR: 'OpenAPIUtilsError:response',
SWAGGER_VALIDATOR: 'swagger_validator', // Deprecated
UNAUTHORIZED: 'unauthorized',
WRONG_INPUT: 'wrong_input',
};
NOTE If you need another kind of error keep reading on tagError method.
This function creates a custom Error with a type and a message you decide.
Example
const { errorFactory, CustomErrorTypes } = require('error-handler-module');
// With Basic Errors
const wrongInputError = errorFactory(CustomErrorTypes.WRONG_INPUT);
wrongInputError('Error message');
/* returns
CustomError {
name: 'CustomError',
type: 'wrong_input',
message: 'Error message'
}
*/
// with your custom types
// With Basic Errors
const customTypeError = errorFactory('custom-type');
customTypeError('Error message');
/* returns
CustomError {
name: 'CustomError',
type: 'custom-type',
message: 'Error message'
}
*/
This is a function which will work as a middleware for your express app so that your errors will response with an HTTP response.
You have to pass logger and metrics objects as parameters (Metrics is not required).
Example
const express = require('express');
const { handleHttpError } = require('error-handler-module');
const app = express();
app.use(handleHttpError(logger, metrics));
This function is going to tag your errors from CustomError
to HTTPErrors
so that handleHttpError middleware will understand them.
It receives error and newTypes (not required)
NewTypes object
In order to add new error types, this object must match this structure:
const newTypes = {
<Error_type_string>: <status_code_number>,
<Error_type_string>: <status_code_number>,
...
};
Example
const { errorFactory } = require('error-handler-module');
// With Custom Errors
const customTypeError = errorFactory('tea-pot-error');
const error = customTypeError('Error message');
// const new Type
const newTypes = {
'tea-pot-error': 418,
};
tagError(error, newTypes);
/* This returns
CustomHTTPError {
name: 'CustomHTTPError',
statusCode: 418,
message: 'Error message',
extra: undefined
}
*/
// This tagError will be added to the catch of the enpoints like this
return next(tagError(error, newTypes))
// With Basic Errors
// With Custom Errors
const customTypeError = errorFactory(CustomErrorTypes.WRONG_INPUT);
const error = customTypeError('Error message');
tagError(error);
/* This returns
CustomHTTPError {
name: 'CustomHTTPError',
statusCode: 400,
message: 'Error message',
extra: undefined
}
*/
const express = require('express');
const {
CustomErrorTypes,
errorFactory,
handleHttpError,
tagError,
} = require('error-handler-module');
const app = express();
const loggerMock = {
error: () => '',
};
app.get('/test-error-basic', (req, res, next) => {
// creating tagged error
const wrongInputError = errorFactory(CustomErrorTypes.NOT_FOUND);
try {
throw wrongInputError('Wrong Input message');
} catch (error) {
return next(tagError(error));
}
});
app.get('/test-error-extended', (req, res, next) => {
// creating a custom tag error
// for example custom db-error
const dbError = errorFactory('db-access-not-allowed');
/*
* New types must be objects with error and a valid status code
*/
const newErrors = {
'db-access-not-allowed': 401,
};
try {
throw dbError('db Error');
} catch (error) {
return next(tagError(error, newErrors));
}
});
app.use(handleHttpError(loggerMock));
module.exports = app;
This project uses Debug if you want more info of your error please run DEBUG=error-handler-module <Your_npm_script>
.
FAQs
This module provides handling error methods for different systems.
The npm package error-handler-module receives a total of 652 weekly downloads. As such, error-handler-module popularity was classified as not popular.
We found that error-handler-module demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.