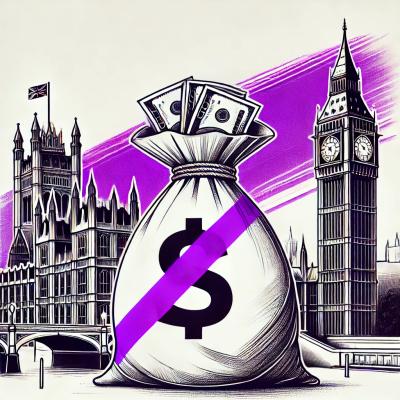
Security News
UK Officials Consider Banning Ransomware Payments from Public Entities
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.
ErrorMail is a Node.js module for sending error emails using the Nodemailer library, and it supports being used as middleware for easy integration into your existing Node.js application.
npm i errormail
const ErrorMail = require('errormail');
const errorMail = new ErrorMail({
transporter: {
service: 'gmail',
auth: {
user: 'youremail@gmail.com',
pass: 'yourpassword',
}
},
receiver: {
to: 'recipient@example.com',
},
showLogs: true,
});
//Usage Example1
const error = new Error('Something went wrong');
errorMail.sendError(error, 'optional-recipient@example.com', 'Optional Subject')
.then(info => {
console.log(`Error email sent: ${info.response}`);
})
.catch(err => {
console.error('Error sending email:', err);
});
//Usage Example2
fs.readFile('path/file/errors.txt', (err, chunk) => {
if (err) {
errorMail.sendError(
err,
'optional-recipient@example.com',
'Optional Subject'
).then(() => console.log('Error email sent.')).catch(console.error);
}
// your code...
});
//Usage Example3
(async () => {
try {
await fs.promises.readFile('path/file/errors.txt');
} catch (err) {
const sendedError = await errorMail.sendError(err, 'optional-recipient@example.com');
console.log(sendedError);
//handle err...
}
})();
The ErrorMail constructor takes an options object with the following properties:
const errorMail = new ErrorMail({
transporter: {
service: 'gmail', // String specifying the email service. Default is 'gmail'.
auth: {
user: 'sender@example.com', // String specifying the email address of the sender.
pass: 'password' // String specifying the password for the email address of the sender.
}
},
receiver: {
to: 'recipient@example.com' // String specifying the email address of the recipient.
},
showLogs: false // Boolean specifying whether to show logs in the console. Default is false.
});
When creating an instance of the ErrorMail class, you can provide the following options:
The ErrorMail constructor takes an options object with the following properties:
Required properties:
Required properties:
const options = {
transporter: {
service: 'gmail',
auth: {
user: 'youremail@gmail.com',
pass: 'yourpassword'
}
},
receiver: {
to: 'recipient1@example.com, recipient2@example.com'
},
showLogs: true
};
const errorMail = new ErrorMail(options);
thenable
and takes three optional parameters:
errorMail.sendError(error, to, subject);
NOTE: When using ErrorMail as a middleware, you can pass the errorMail.sendError method as a parameter to the app.use function. The errorHandler middleware will catch any errors that occur in subsequent middleware or routes, and pass them to errorMail.sendError to be sent as an email.
ErrorMail can be used as a middleware to send error emails automatically when an error occurs in your Node.js application. To use ErrorMail as a middleware, you can pass the errorMail.sendError method as a parameter to the next function in your error handling middleware.
Here's an example of how you can use ErrorMail with middleware:
const express = require('express');
const ErrorMail = require('errormail');
const app = express();
const { errorHandler } = new ErrorMail({
transporter: {
service: 'gmail',
auth: {
user: 'youremail@gmail.com',
pass: 'yourpassword',
}
},
receiver: {
to: 'recipient@example.com',
},
showLogs: true,
});
app.use(errorHandler);
app.get('/', (req, res) => {
throw new Error('Something went wrong');
});
app.listen(3000, () => console.log('Server started on port 3000'));
In the example above, we create an instance of the ErrorMail class and pass it to our error handling middleware. When an error occurs in our application, the middleware will call the errorMail.sendError method and pass the error object as a parameter. The sendError method will then send an error email to the specified recipient.
After the email is sent, the middleware will pass the error to the next middleware or error handler using the next function.
License This project is licensed under the MIT License - see the LICENSE file for details.
This README.md
file includes information on how to install the module, as well as an example usage snippet that shows how to create an instance of the ErrorMail
class and send an error email with optional recipient and subject parameters.
It also includes an Options
section that describes the properties of the options object that can be passed to the ErrorMail
constructor, as well as the optional parameters for the sendError
method.
Finally, it includes a License
section that describes the license for the module.
FAQs
Error mail sender
The npm package errormail receives a total of 0 weekly downloads. As such, errormail popularity was classified as not popular.
We found that errormail demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.