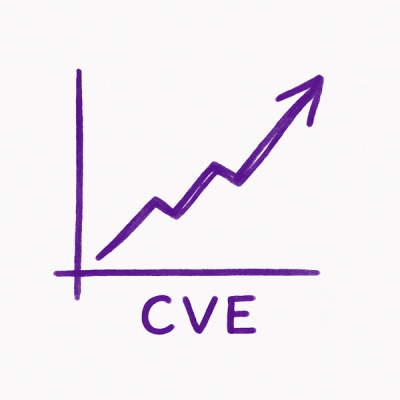
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
es6-javascript-validators
Advanced tools
An ES6 module with various methods and exports for validating variables in JavaScript.
Stop for a second. Do you see this a lot in your code?:
if (typeof var1 === 'string' && var1.length > 0) {
// other code here...
}
I see it all the time -- and in tons of places too. Or, my favorite:
if (typeof var2 === 'object' && var2 !== null) {
// but what if it's an empty object?
}
Well, lets collectively stop that. Lets use simple functions to do that for us! es6-javascript-validators is a collection of functions which will do all of this for you and keep your code consistent and clean.
import { isStr, isObj } from 'your/path/to/module.js';
console.log(isStr('')); // true
console.log(isStr([])); // false
console.log(isStr(null)); // false
console.log(isStr(0)); // false
console.log(isObj('')); // false
console.log(isObj([])); // false
console.log(isObj(null)); // false
console.log(isObj({})); // true
import Util from 'your/path/to/module.js';
console.log(Util.isObj({})); // true
console.log(Util.validObj({})); // false
console.log(Util.validObj({ a:1, b:2 })); // true
console.log(Util.isStr('')); // true
console.log(Util.validStr('')); // false
console.log(Util.isArr([])); // true
console.log(Util.validArr([1, 2, 3])); // true
//.. and so on
FAQs
An ES6 module with various methods and exports for validating variables in JavaScript.
The npm package es6-javascript-validators receives a total of 0 weekly downloads. As such, es6-javascript-validators popularity was classified as not popular.
We found that es6-javascript-validators demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.