es6-tween
ES6 implementation of tween.js
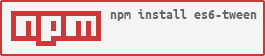
let coords = { x: 0, y: 0 };
let tween = new TWEEN.Tween(coords)
.to({ x: 100, y: 100 }, 1000)
.on('update', ({x, y}) => {
console.log(`The values is x: ${x} and y: ${y}`);
})
.start();
TWEEN.autoPlay(true);
}
Demos
Installation
Download the library and include it in your code:
<script src="js/Tween.js"></script>
CDN-Hosted version
- See cdnjs-hosted version for get which result you want
- NOTE:
@latest
suffix sometimes saves life by loading latest, because sometimes CDN services will not load the latest
<script src="https://cdn.jsdelivr.net/npm/es6-tween"></script>
<script src="https://unpkg.com/es6-tween"></script>
<script src="https://npmcdn.com/es6-tween"></script>
More advanced users might want to...
Using grunt
PR are welcome...
Using gulp
PR are welcome...
Using import
import { Easing, Interpolation, Tween, autoPlay } from 'es6-tween';
<script src="https://unpkg.com/getlibs"></script>
<script type="x-module">
import { Easing, Interpolation, Tween, autoPlay } from 'es6-tween';
</script>
Using npm
, yarn
or bower
$ yarn add es6-tween
$ npm install es6-tween
$ bower install es6-tween
Then include the Tween.js module with the standard node.js require
:
const { Tween, Easing, Interpolation, autoPlay } = require('es6-tween');
And you can use Tween.js as in all other examples--for example:
const t = new Tween( );
t.start();
You can run script commands to build modules into single UMD
compatible file:
Using commands
$ npm run build
$ npm run dev
Then reference the library source:
<script src="dist/Tween.min.js"></script>
Features
- Tweens everything you give them, string (numbers only), number, number of arrays, number of object, etc...
- Can use CSS units (e.g. appending
px
) - Can interpolate colours (partially)
- Easing functions are reusable outside of Tween
- Can also use custom easing functions
- Much of easings
Documentation
Caveats
We removed the shim from core functions for reduce size and improve execution speed, so you need some polyfills to work better
Array.isArray
*Object.assign
requestAnimationFrame
*performance.now()
*cancelAnimationFrame
*Array.from
*
* - optional for best performance, fallback supported in core
Examples
Demos with this version are not yet implemented, sorry.
Tests
You need to install npm
first--this comes with node.js, so install that one first. Then, cd to es6-tween
's directory and run:
npm install
if running the tests for the first time, to install additional dependencies for running tests, and then run
npm test
or you can go here for more information, tests and etc...
every time you want to run the tests.
If you want to add any feature or change existing features, you must run the tests to make sure you didn't break anything else. If you send a PR to add something new and it doesn't have tests, or the tests don't pass, the PR won't be accepted. See contributing for more information.
Every PR, Commits and Update now automacilly updates the version based on commit message with semantic-release
with Travis CI
People
All contributors.
Thanks to:
- @sole (author of this library)
- tween.js contributors
- @michaelvillar for physics easing
- Rollup, Buble, Travis CI, semantic-release and others (make issue, if i'm missed you) with their teams, devs and supporters
Projects using tween.js
If you using our app and happy with this and share your app? Please make PR and we append to there your project