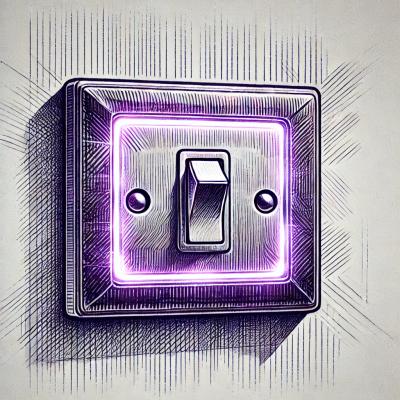
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
esbuild-plugin-pipe
Advanced tools
Pipe esbuild plugins output.
A pipe is a form of redirection that is used to send the output of one program to another program for further processing.
⚠️ The plugins have to support piping.
npm install esbuild-plugin-pipe --save-dev
esbuild.config.js
import esbuild from 'esbuild';
import pipe from 'esbuild-plugin-pipe';
esbuild
.build({
entryPoints: ['index.js'],
bundle: true,
outfile: 'main.js',
plugins: [
pipe({
plugins: [...]
})
]
})
.catch(() => process.exit(1));
package.json
{
"type": "module",
"scripts": {
"start": "node esbuild.config.js"
}
}
esbuild.config.js
pipe({
filter: /.*/,
namespace: '',
plugins: []
});
If you are a plugin maker, it's really easy to support piping. Here’s a commented plugin example.
const pluginExample = () => ({
name: 'example',
setup(build, { transform } = {}) {
// The `setup` function receives a new `transform` argument if it’s in a pipe.
// Create a function and move all the content of your `onLoad` function in it, except the `readfile`.
const transformContents = ({ args, contents }) => {
// It receives an object as an argument containing both the standard arguments of the `onLoad` function
// and the `contents` of the previous plugin or file.
// Move your code here. It should return `contents`.
return { contents };
};
// If the `transform` argument exists, pass it to your new function.
if (transform) return transformContents(transform);
// Else call your `onLoad` function.
build.onLoad({ filter: /.*/ }, async args => {
// Read the files from disk.
const contents = await fs.promises.readFile(args.path, 'utf8');
// Call your function, but this time pass an object with the `onLoad` arguments and the file `contents`.
return transformContents({ args, contents });
});
}
});
export default pluginExample;
esbuild-plugin-babel → Babel plugin for esbuild.
esbuild-plugin-postcss-literal → PostCSS tagged template literals plugin for esbuild.
FAQs
Pipe esbuild plugins output.
We found that esbuild-plugin-pipe demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.