esbuild-plugins-node-modules-polyfill
Polyfills nodejs builtin modules for the browser.

Description
Polyfills nodejs builtin modules and globals for the browser.
Features
- Written In Typescript
- Offers CJS and ESM builds
- Full TypeScript & JavaScript support
- Supports
node:
protocol - Supports
browser
field in package.json
- Optionally injects globals
- Optionally provides empty fallbacks
Install
npm install --save-dev esbuild-plugins-node-modules-polyfill
Usage
import { nodeModulesPolyfillPlugin } from 'esbuild-plugins-node-modules-polyfill';
import { build } from 'esbuild';
build({
plugins: [nodeModulesPolyfillPlugin()],
});
Inject globals when detected:
import { nodeModulesPolyfillPlugin } from 'esbuild-plugins-node-modules-polyfill';
import { build } from 'esbuild';
build({
plugins: [
nodeModulesPolyfillPlugin({
globals: {
process: true,
Buffer: true,
},
}),
],
});
Note
If you are utilizing the modules
option, ensure that you include polyfills for the global modules you are using.
Configure which modules to polyfill:
import { nodeModulesPolyfillPlugin } from 'esbuild-plugins-node-modules-polyfill';
import { build } from 'esbuild';
build({
plugins: [
nodeModulesPolyfillPlugin({
modules: ['crypto'],
}),
],
});
import { nodeModulesPolyfillPlugin } from 'esbuild-plugins-node-modules-polyfill';
import { build } from 'esbuild';
build({
plugins: [
nodeModulesPolyfillPlugin({
modules: {
crypto: true,
fs: false,
},
}),
],
});
Provide empty polyfills:
Provide empty polyfills for specific modules:
import { nodeModulesPolyfillPlugin } from 'esbuild-plugins-node-modules-polyfill';
import { build } from 'esbuild';
build({
plugins: [
nodeModulesPolyfillPlugin({
modules: {
fs: 'empty',
crypto: true,
},
}),
],
});
Provide empty fallbacks for any unpolyfilled modules:
import { nodeModulesPolyfillPlugin } from 'esbuild-plugins-node-modules-polyfill';
import { build } from 'esbuild';
build({
plugins: [
nodeModulesPolyfillPlugin({
fallback: 'empty',
}),
],
});
Provide empty fallbacks for any unconfigured modules:
import { nodeModulesPolyfillPlugin } from 'esbuild-plugins-node-modules-polyfill';
import { build } from 'esbuild';
build({
plugins: [
nodeModulesPolyfillPlugin({
fallback: 'empty',
modules: {
crypto: true,
},
}),
],
});
Fail the build when certain modules are used:
Warning
The write
option in esbuild
must be false
to support this.
import { nodeModulesPolyfillPlugin } from 'esbuild-plugins-node-modules-polyfill';
import { build } from 'esbuild';
const buildResult = await build({
write: false,
plugins: [
nodeModulesPolyfillPlugin({
modules: {
crypto: 'error',
path: true,
},
}),
],
});
Fail the build when a module is not polyfilled or configured:
Warning
The write
option in esbuild
must be false
to support this.
import { nodeModulesPolyfillPlugin } from 'esbuild-plugins-node-modules-polyfill';
import { build } from 'esbuild';
const buildResult = await build({
write: false,
plugins: [
nodeModulesPolyfillPlugin({
fallback: 'error',
modules: {
path: true,
},
}),
],
});
Provide a custom error formatter when a module is not polyfilled or configured:
Return an esbuild PartialMessage
object from the formatError
function to override any properties of the default error message.
Warning
The write
option in esbuild
must be false
to support this.
import { nodeModulesPolyfillPlugin } from 'esbuild-plugins-node-modules-polyfill';
import { build } from 'esbuild';
const buildResult = await build({
write: false,
plugins: [
nodeModulesPolyfillPlugin({
fallback: 'error',
modules: {
path: true,
},
formatError({ moduleName, importer, polyfillExists }) {
return {
text: polyfillExists
? `Polyfill has not been configured for "${moduleName}", imported by "${importer}"`
: `Polyfill does not exist for "${moduleName}", imported by "${importer}"`,
};
},
}),
],
});
Buy me some doughnuts
If you want to support me by donating, you can do so by using any of the following methods. Thank you very much in advance!
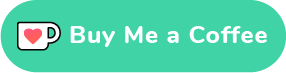
Contributors ✨
Thanks goes to these wonderful people: