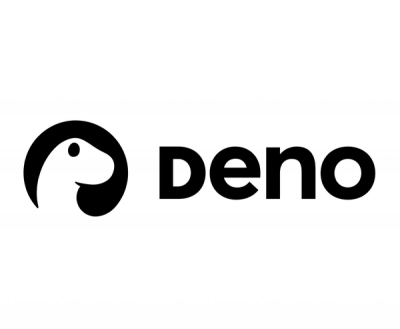
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
eslint-config-molindo
Advanced tools
Molindo ESLint config that implements our styleguide and helps to catch errors.
yarn add eslint eslint-config-molindo prettier --dev
eslint.config.mjs
:import {getPresets} from 'eslint-config-molindo';
export default [
...(await getPresets(
// Base config
'typescript', // or 'javascript'
// Optional extensions
'react',
'cssModules',
'tailwind',
'jest',
'cypress',
'vitest'
)),
{
// Your custom config
}
];
window
, you can add them to your config:// eslint.config.mjs
import globals from 'globals';
export default [
// ...
{
languageOptions: {
globals: {
...globals.browser,
...globals.node
}
}
}
];
package.json
:"prettier": "eslint-config-molindo/.prettierrc.json"
tsconfig.json
:"extends": "eslint-config-molindo/tsconfig.json"
Happy linting!
To validate your code in a CI pipeline, add the following to your package.json
:
"scripts": {
"lint": "eslint src && prettier src --check"
}
The following two extensions are recommended:
To auto-fix errors from ESLint as well as Prettier on save, you can use the following configuration:
// settings.json (VSCode)
{
"editor.codeActionsOnSave": {
"source.fixAll.eslint": "always"
},
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.formatOnSave": true
}
8.0.0
eslint-import-resolver-typescript
is now used for TypeScript files to configure eslint-plugin-import
correctly. This now correctly takes into consideration paths
from tsconfig.json
when determining ordering of imports.eslint-plugin-unicorn
:
unicorn/prefer-array-index-of
unicorn/prefer-optional-catch-binding
unicorn/no-useless-spread
@typescript-eslint
:
@typescript-eslint/prefer-optional-chain
@typescript-eslint/no-unnecessary-condition
eslint-plugin-import
:
import/no-useless-path-segments
.mts
, .mtsx
, .cts
and .ctsx
files.eslint-plugin-unused-imports
to remove unused imports during autofix.sort-imports
.eslint.config.mjs
)prettier --check
to your CI pipeline.valid-jsdoc
rule has been removed since it was removed from ESLint core and is only available via an additional plugin. If you'd like to keep this rule, add eslint-plugin-jsdoc
to your project.src
directory is no longer resolved by default. For TypeScript projects, paths
from tsconfig.json
are now suggested as the alternative and will be resolved by default. For JavaScript projects, you can add settings: {'import/resolver': {node: {paths: ['node_modules', 'src']}}}
to your ESLint config.FAQs
Molindo ESLint config that implements our styleguide and helps to catch errors.
The npm package eslint-config-molindo receives a total of 451 weekly downloads. As such, eslint-config-molindo popularity was classified as not popular.
We found that eslint-config-molindo demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.