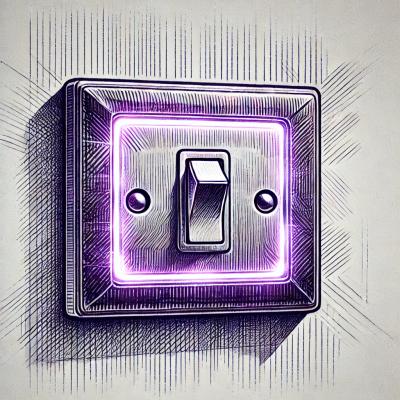
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
eslint-import-resolver-babel-module
Advanced tools
babel-plugin-module-resolver resolver for eslint-plugin-import
The eslint-import-resolver-babel-module package is an ESLint plugin that allows you to resolve import paths using Babel's module resolver. This is particularly useful for projects that use Babel to transform module paths, as it ensures that ESLint can correctly resolve and lint these paths.
Custom Module Resolution
This feature allows you to configure ESLint to use Babel's module resolver for resolving import paths. This ensures that ESLint can correctly resolve paths that are transformed by Babel.
{
"settings": {
"import/resolver": {
"babel-module": {}
}
}
}
Alias Configuration
This feature allows you to define custom aliases for module paths. This is useful for simplifying import statements and making your codebase more maintainable.
{
"settings": {
"import/resolver": {
"babel-module": {
"alias": {
"@components": "./src/components",
"@utils": "./src/utils"
}
}
}
}
}
Custom Extensions
This feature allows you to specify custom file extensions that should be resolved by Babel's module resolver. This is useful for projects that use multiple file types, such as JavaScript and TypeScript.
{
"settings": {
"import/resolver": {
"babel-module": {
"extensions": [".js", ".jsx", ".ts", ".tsx"]
}
}
}
}
The eslint-import-resolver-webpack package is an ESLint plugin that allows you to resolve import paths using Webpack's module resolver. This is useful for projects that use Webpack to bundle their code, as it ensures that ESLint can correctly resolve and lint these paths. Compared to eslint-import-resolver-babel-module, this package is more suitable for projects that rely heavily on Webpack for module resolution.
The eslint-import-resolver-alias package is an ESLint plugin that allows you to resolve import paths using custom alias configurations. This is useful for projects that use custom aliases for module paths, similar to eslint-import-resolver-babel-module. However, it does not rely on Babel and is more lightweight, making it a good choice for projects that do not use Babel for module resolution.
A babel-plugin-module-resolver resolver for eslint-plugin-import
npm install --save-dev eslint-plugin-import eslint-import-resolver-babel-module
Inside your .eslintrc
file, pass this resolver to eslint-plugin-import
:
"settings": {
"import/resolver": {
"babel-module": {}
}
}
And see babel-plugin-module-resolver to know how to configure your aliases.
{
"extends": "airbnb",
"rules": {
"comma-dangle": 0
},
"settings": {
"import/resolver": {
"babel-module": {}
}
}
}
Some babel plugins like babel-plugin-import-directory or babel-plugin-wildcard allow to import directories (i.e. each file inside a directory) as an object. In order to support this, you can activate the allowExistingDirectories
option as follows:
"settings": {
"import/resolver": {
"babel-module": { allowExistingDirectories: true }
}
}
Now when you import a directory like this, the ESLint plugin won't complain and recognize the existing directory:
import * as Items from './dir';
MIT, see LICENSE.md for details.
FAQs
babel-plugin-module-resolver resolver for eslint-plugin-import
The npm package eslint-import-resolver-babel-module receives a total of 160,603 weekly downloads. As such, eslint-import-resolver-babel-module popularity was classified as popular.
We found that eslint-import-resolver-babel-module demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.