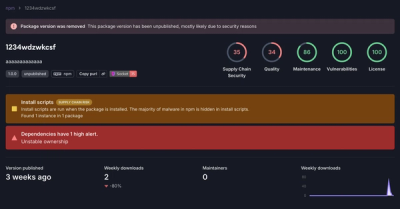
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
eslint-plugin-eslint-plugin
Advanced tools
eslint-plugin-eslint-plugin is an ESLint plugin designed to help developers create and maintain their own ESLint plugins. It provides rules and utilities that enforce best practices and catch common mistakes in ESLint plugin development.
Rule to enforce meta property in rule definitions
This feature ensures that each rule in your ESLint plugin has a `meta.docs.url` property, which is useful for documentation purposes.
module.exports = {
rules: {
'require-meta-docs-url': require('./lib/rules/require-meta-docs-url')
}
};
Rule to enforce correct rule tester usage
This feature checks that your rule tests are consistent and follow best practices, ensuring that your tests are reliable and maintainable.
module.exports = {
rules: {
'consistent-output': require('./lib/rules/consistent-output')
}
};
Rule to enforce correct use of context.report
This feature ensures that you are using the `context.report` API correctly, avoiding deprecated methods and promoting the use of the latest API.
module.exports = {
rules: {
'no-deprecated-report-api': require('./lib/rules/no-deprecated-report-api')
}
};
eslint-plugin-eslint-comments provides rules for best practices around ESLint directive comments (e.g., `// eslint-disable`). It helps ensure that these comments are used correctly and not overused, which is somewhat related to maintaining ESLint configurations but focuses on comments rather than plugin development.
eslint-plugin-node provides rules for Node.js-specific code, ensuring that your code adheres to best practices for Node.js development. While it is not focused on ESLint plugin development, it shares the goal of improving code quality through custom rules.
eslint-plugin-import offers rules to ensure proper import/export syntax and practices in JavaScript modules. It helps catch common issues with ES6 imports/exports, which is different from plugin development but similarly aims to improve code quality through linting.
An ESLint plugin for linting ESLint plugins. Rules written in CJS, ESM, and TypeScript are all supported.
You'll first need to install ESLint:
npm i eslint --save-dev
Next, install eslint-plugin-eslint-plugin
:
npm install eslint-plugin-eslint-plugin --save-dev
Here's an example ESLint configuration that:
sourceType
to script
for CJS plugins (most users) (use module
for ESM/TypeScript)recommended
configuration{
"extends": ["plugin:eslint-plugin/recommended"],
"rules": {
"eslint-plugin/require-meta-docs-description": "error"
}
}
eslint.config.js
(requires eslint>=v8.23.0)const eslintPlugin = require('eslint-plugin-eslint-plugin');
module.exports = [
eslintPlugin.configs['flat/recommended'],
{
rules: {
'eslint-plugin/require-meta-docs-description': 'error',
},
},
];
💼 Configurations enabled in.
✅ Set in the recommended
configuration.
🔧 Automatically fixable by the --fix
CLI option.
💡 Manually fixable by editor suggestions.
💭 Requires type information.
Name | Description | 💼 | 🔧 | 💡 | 💭 |
---|---|---|---|---|---|
fixer-return | require fixer functions to return a fix | ✅ | |||
meta-property-ordering | enforce the order of meta properties | 🔧 | |||
no-deprecated-context-methods | disallow usage of deprecated methods on rule context objects | ✅ | 🔧 | ||
no-deprecated-report-api | disallow the version of context.report() with multiple arguments | ✅ | 🔧 | ||
no-missing-message-ids | disallow messageId s that are missing from meta.messages | ✅ | |||
no-missing-placeholders | disallow missing placeholders in rule report messages | ✅ | |||
no-property-in-node | disallow using in to narrow node types instead of looking at properties | 💭 | |||
no-unused-message-ids | disallow unused messageId s in meta.messages | ✅ | |||
no-unused-placeholders | disallow unused placeholders in rule report messages | ✅ | |||
no-useless-token-range | disallow unnecessary calls to sourceCode.getFirstToken() and sourceCode.getLastToken() | ✅ | 🔧 | ||
prefer-message-ids | require using messageId instead of message or desc to report rule violations | ✅ | |||
prefer-object-rule | disallow function-style rules | ✅ | 🔧 | ||
prefer-placeholders | require using placeholders for dynamic report messages | ||||
prefer-replace-text | require using replaceText() instead of replaceTextRange() | ||||
report-message-format | enforce a consistent format for rule report messages | ||||
require-meta-docs-description | require rules to implement a meta.docs.description property with the correct format | ||||
require-meta-docs-recommended | require rules to implement a meta.docs.recommended property | ||||
require-meta-docs-url | require rules to implement a meta.docs.url property | 🔧 | |||
require-meta-fixable | require rules to implement a meta.fixable property | ✅ | |||
require-meta-has-suggestions | require suggestable rules to implement a meta.hasSuggestions property | ✅ | 🔧 | ||
require-meta-schema | require rules to implement a meta.schema property | ✅ | 💡 | ||
require-meta-type | require rules to implement a meta.type property | ✅ |
Name | Description | 💼 | 🔧 | 💡 | 💭 |
---|---|---|---|---|---|
consistent-output | enforce consistent use of output assertions in rule tests | ||||
no-identical-tests | disallow identical tests | ✅ | 🔧 | ||
no-only-tests | disallow the test case property only | ✅ | 💡 | ||
prefer-output-null | disallow invalid RuleTester test cases where the output matches the code | ✅ | 🔧 | ||
test-case-property-ordering | require the properties of a test case to be placed in a consistent order | 🔧 | |||
test-case-shorthand-strings | enforce consistent usage of shorthand strings for test cases with no options | 🔧 |
Name | Description | |
---|---|---|
✅ | recommended | enables all recommended rules in this plugin |
rules-recommended | enables all recommended rules that are aimed at linting ESLint rule files | |
tests-recommended | enables all recommended rules that are aimed at linting ESLint test files | |
all | enables all rules in this plugin, excluding those requiring type information | |
all-type-checked | enables all rules in this plugin, including those requiring type information | |
rules | enables all rules that are aimed at linting ESLint rule files | |
tests | enables all rules that are aimed at linting ESLint test files |
The list of recommended rules will only change in a major release of this plugin. However, new non-recommended rules might be added in a minor release of this plugin. Therefore, using the all
, rules
, and tests
presets is not recommended for production use, because the addition of new rules in a minor release could break your build.
Both flat and eslintrc configs are supported. For example, to enable the recommended
preset, use:
eslint.config.js
const eslintPlugin = require('eslint-plugin-eslint-plugin');
module.exports = [eslintPlugin.configs['flat/recommended']];
.eslintrc.json
{
"extends": ["plugin:eslint-plugin/recommended"]
}
Or to apply linting only to the appropriate rule or test files:
eslint.config.js
const eslintPlugin = require('eslint-plugin-eslint-plugin');
module.exports = [
{
files: ['lib/rules/*.{js,ts}'],
...eslintPlugin.configs['flat/rules-recommended'],
},
{
files: ['tests/lib/rules/*.{js,ts}'],
...eslintPlugin.configs['flat/tests-recommended'],
},
];
.eslintrc.js
{
"overrides": [
{
"files": ["lib/rules/*.{js,ts}"],
"extends": ["plugin:eslint-plugin/rules-recommended"]
},
{
"files": ["tests/lib/rules/*.{js,ts}"],
"extends": ["plugin:eslint-plugin/tests-recommended"]
}
]
}
FAQs
An ESLint plugin for linting ESLint plugins
The npm package eslint-plugin-eslint-plugin receives a total of 237,861 weekly downloads. As such, eslint-plugin-eslint-plugin popularity was classified as popular.
We found that eslint-plugin-eslint-plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.