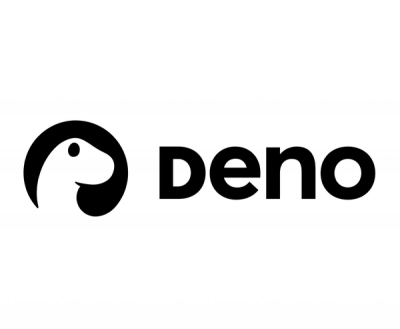
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
eslint-plugin-promise
Advanced tools
The eslint-plugin-promise package is an ESLint plugin that enforces best practices and common idioms for dealing with promises in JavaScript. It provides a set of rules that catch common mistakes and enforce conventions when working with promises.
Avoiding creation of new promises outside of utility libraries
This rule ensures that the executor function of a new Promise has the parameters named 'resolve' and 'reject'.
/* eslint promise/param-names: 'error' */
new Promise((resolve, reject) => { /* executor function */ });
Ensuring that each time a then() is applied to a promise, a catch() is applied as well
This rule enforces the use of catch() on unhandled promises.
/* eslint promise/catch-or-return: 'error' */
promise.then(function(data) {
// handle data
}).catch(function(error) {
// handle error
});
Enforcing the return of a promise in certain contexts
This rule ensures that promise chains always return a value, preventing silent failures and unhandled rejections.
/* eslint promise/always-return: 'error' */
function foo() {
return doSomething().then(function() {
// do something else
return 'result';
});
}
This plugin provides ESLint rules for async/await syntax, which is related to promises. It helps to enforce best practices and catch common mistakes when using async/await, but it does not focus on promises directly.
Part of the 'eslint-plugin-standard' plugin includes rules that are applicable to promises, as it adheres to the JavaScript Standard Style. However, it covers a broader range of JavaScript features and is not solely focused on promises.
Enforce best practices for JavaScript promises.
You'll first need to install ESLint:
$ npm i eslint --save-dev
Next, install eslint-plugin-promise
:
$ npm install eslint-plugin-promise --save-dev
Note: If you installed ESLint globally (using the -g
flag) then you must also install eslint-plugin-promise
globally.
Add promise
to the plugins section of your .eslintrc
configuration file. You can omit the eslint-plugin-
prefix:
{
"plugins": [
"promise"
]
}
Then configure the rules you want to use under the rules section.
{
"rules": {
"promise/always-return": "error",
"promise/no-return-wrap": "error",
"promise/param-names": "error",
"promise/catch-or-return": "error",
"promise/no-native": "off",
"promise/no-nesting": "warn",
"promise/no-promise-in-callback": "warn",
"promise/no-callback-in-promise": "warn",
"promise/avoid-new": "warn"
}
}
catch-or-return
Enforces the use of catch
on un-returned promises.no-return-wrap
Avoid wrapping values in Promise.resolve
or Promise.reject
when not needed.param-names
Enforce consistent param names when creating new promises.always-return
Return inside each then
to create readable and reusable Promise chains.no-native
In an ES5 environment, make sure to create a Promise
constructor before using.no-nesting
Avoid nested .then() or .catch() statementsno-promise-in-callback
Avoid using promises inside of callbacksno-callback-in-promise
Avoid calling cb()
inside of a then()
(use nodeify] instead)avoid-new
Avoid creating new
promises outside of utility libs (use pify instead)prefer-await-to-then
Prefer await
to then()
for reading Promise valuesprefer-await-to-callbacks
Prefer async/await to the callback patterncatch-or-return
Ensure that each time a then()
is applied to a promise, a
catch()
is applied as well. Exceptions are made if you are
returning that promise.
myPromise.then(doSomething).catch(errors);
myPromise.then(doSomething).then(doSomethingElse).catch(errors);
function doSomethingElse() { return myPromise.then(doSomething) }
myPromise.then(doSomething);
myPromise.then(doSomething, catchErrors); // catch() may be a little better
function doSomethingElse() { myPromise.then(doSomething) }
allowThen
You can pass an { allowThen: true }
as an option to this rule
to allow for .then(null, fn)
to be used instead of catch()
at
the end of the promise chain.
terminationMethod
You can pass a { terminationMethod: 'done' }
as an option to this rule
to require done()
instead of catch()
at the end of the promise chain.
This is useful for many non-standard Promise implementations.
You can also pass an array of methods such as
{ terminationMethod: ['catch', 'asCallback', 'finally'] }
.
This will allow any of
Promise.resolve(1).then(() => { throw new Error('oops') }).catch(logerror)
Promise.resolve(1).then(() => { throw new Error('oops') }).asCallback(cb)
Promise.resolve(1).then(() => { throw new Error('oops') }).finally(cleanUp)
always-return
Ensure that inside a then()
you make sure to return
a new promise or value.
See http://pouchdb.com/2015/05/18/we-have-a-problem-with-promises.html (rule #5)
for more info on why that's a good idea.
We also allow someone to throw
inside a then()
which is essentially the same as return Promise.reject()
.
myPromise.then((val) => val * 2));
myPromise.then(function(val) { return val * 2; });
myPromise.then(doSomething); // could be either
myPromise.then((b) => { if (b) { return "yes" } else { return "no" } });
myPromise.then(function(val) {});
myPromise.then(() => { doSomething(); });
myPromise.then((b) => { if (b) { return "yes" } else { forgotToReturn(); } });
param-names
Enforce standard parameter names for Promise constructors
new Promise(function (resolve) { ... })
new Promise(function (resolve, reject) { ... })
new Promise(function (reject, resolve) { ... }) // incorrect order
new Promise(function (ok, fail) { ... }) // non-standard parameter names
Ensures that new Promise()
is instantiated with the parameter names resolve, reject
to avoid confusion with order such as reject, resolve
. The Promise constructor uses the RevealingConstructor pattern. Using the same parameter names as the language specification makes code more uniform and easier to understand.
no-native
Ensure that Promise
is included fresh in each file instead of relying
on the existence of a native promise implementation. Helpful if you want
to use bluebird
or if you don't intend to use an ES6 Promise shim.
var Promise = require("bluebird");
var x = Promise.resolve("good");
var x = Promise.resolve("bad");
no-return-wrap
Ensure that inside a then()
or a catch()
we always return
or throw
a raw value instead of wrapping in Promise.resolve
or Promise.reject
myPromise.then(function(val) {
return val * 2;
});
myPromise.then(function(val) {
throw "bad thing";
});
myPromise.then(function(val) {
return Promise.resolve(val * 2);
});
myPromise.then(function(val) {
return Promise.reject("bad thing");
})
3.4.2
FAQs
Enforce best practices for JavaScript promises
The npm package eslint-plugin-promise receives a total of 4,108,108 weekly downloads. As such, eslint-plugin-promise popularity was classified as popular.
We found that eslint-plugin-promise demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.