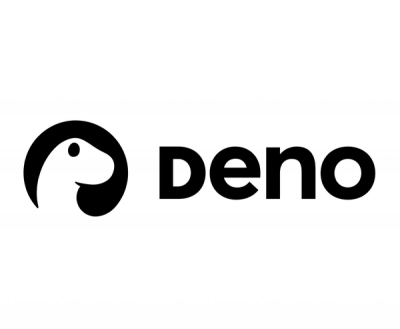
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
+ ██╗
+ ██████╗ ███████╗ █████═████╗ ██████╗ ██████╗██║ ██╗
+██╔═══██╗██╔═════╝██╔══██╔══██╗██╔═══██╗██╔════╝██║ ██╔╝
+████████║╚██████╗ ██║ ██║ ██║██║ ██║██║ ██████╔╝
+██╔═════╝ ╚════██╗██║ ██║ ██║██║ ██║██║ ██╔══██╗
+╚███████╗███████╔╝██║ ██║ ██║╚██████╔╝╚██████╗██║ ╚██╗
+ ╚══════╝╚══════╝ ╚═╝ ╚═╝ ╚═╝ ╚═════╝ ╚═════╝╚═╝ ╚═╝
esmock provides native ESM import mocking for unit tests. Use examples below as a quick-start guide or use the descriptive and friendly esmock guide here.
esmock
is used with node's --loader
{
"name": "give-esmock-a-star",
"type": "module",
"scripts": {
"test": "node --loader=esmock --test",
"test-mocha": "mocha --loader=esmock",
"test-tap": "NODE_OPTIONS=--loader=esmock tap",
"test-ava": "NODE_OPTIONS=--loader=esmock ava",
"test-uvu": "NODE_OPTIONS=--loader=esmock uvu spec",
"test-tsm": "node --loader=tsm --loader=esmock --test *ts",
"test-ts-node": "node --loader=ts-node/esm --loader=esmock --test *ts"
}
}
esmock
has the below signature
await esmock(
'./to/module.js', // path to target module being tested
{ ...childmocks }, // mock definitions imported by target module
{ ...globalmocks }) // mock definitions imported everywhere
esmock
examples
import test from 'node:test'
import assert from 'node:assert/strict'
import esmock from 'esmock'
test('should mock local files and packages', async () => {
const main = await esmock('../src/main.js', {
stringifierpackage: JSON.stringify,
'../src/hello.js': {
default: () => 'world',
exportedFunction: () => 'foo'
}
})
assert.strictEqual(main(), JSON.stringify({ test: 'world foo' }))
})
test('should do global instance mocks —third param', async () => {
const { getFile } = await esmock('../src/main.js', {}, {
fs: {
readFileSync: () => 'returns this globally'
}
})
assert.strictEqual(getFile(), 'returns this globally')
})
test('should mock "await import()" using esmock.p', async () => {
// using esmock.p, mock definitions are kept in cache
const doAwaitImport = await esmock.p('../awaitImportLint.js', {
eslint: { ESLint: cfg => cfg }
})
// mock definition is returned from cache, when import is called
assert.strictEqual(await doAwaitImport('cfg'), 'cfg')
// a bit more info are found in the descriptive guide
})
// a "partial mock" merges the new and original definitions
test('should suppport partial mocks', async () => {
const pathWrap = await esmock('../src/pathWrap.js', {
path: { dirname: () => '/path/to/file' }
})
// an error, because path.basename was not defined
await assert.rejects(async () => pathWrap.basename('/dog.png'), {
name: 'TypeError',
message: 'path.basename is not a function'
})
// use esmock.px to create a "partial mock"
const pathWrapPartial = await esmock.px('../src/pathWrap.js', {
path: { dirname: () => '/home/' }
})
// no error, because "core" path.basename was merged into the mock
assert.deepEqual(pathWrapPartial.basename('/dog.png'), 'dog.png')
assert.deepEqual(pathWrapPartial.dirname(), '/home/')
})
FAQs
provides native ESM import and globals mocking for unit tests
The npm package esmock receives a total of 23,116 weekly downloads. As such, esmock popularity was classified as popular.
We found that esmock demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.