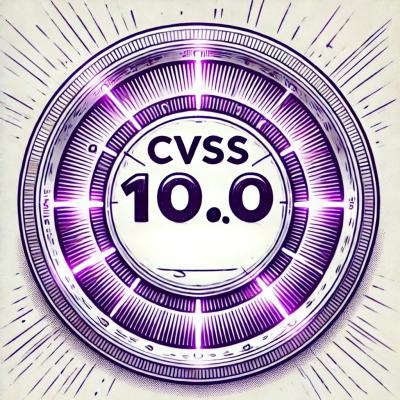
Security News
cURL Project and Go Security Teams Reject CVSS as Broken
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Easy way to add BlockStream.info API to your JS application.
Help me to stack sats! :blush:
bc1qvyw5kfx0uhu7g5qeahzna8tl9c5jsuemjumxzj
Or donate via Lightning Network!
Using npm:
$ npm install esplora-js
Using yarn:
$ yarn add esplora-js
Get an object where the key is the confirmation target (in number of blocks) and the value is the estimated feerate (in sat/vB).
import { fees } from 'esplora-js';
...
const feesRecommended = await fees.getFeesRecommended();
console.log(feesRecommended);
Get mempool backlog statistics.
import { mempool } from 'esplora-js';
...
const getMempool = await mempool.getMempool();
console.log(getMempool);
Get the full list of txids in the mempool as an array. The order of the txids is arbitrary and does not match bitcoind's.
import { mempool } from './../src/';
...
const getMempoolTxids = await mempool.getMempoolTxids();
console.log(getMempoolTxids);
Get a list of the last 10 transactions to enter the mempool. Each transaction object contains simplified overview data, with the following fields: txid, fee, vsize and value.
import { mempool } from 'esplora-js';
...
const getMempoolRecent = await mempool.getMempoolRecent();
console.log(getMempoolRecent);
Returns information about a block.
Parameters:
import { blocks } from 'esplora-js';
...
const block = await blocks.getBlock({
hash: '000000000000000015dc...'
});
console.log(block);
Returns the block status.
Parameters:
import { blocks } from 'esplora-js';
...
const blockStatus = await blocks.getBlockStatus({
hash: '000000000000000015dc...'
});
console.log(blockStatus);
Returns the hex-encoded block header.
Parameters:
import { blocks } from 'esplora-js';
...
const blockHeader = await blocks.getBlockHeader({
hash: '000000000000000015dc...'
});
console.log(blockStatus);
Returns a list of transactions in the block (up to 25 transactions beginning at start_index).
Parameters:
import { blocks } from 'esplora-js';
...
const blockTxs = await blocks.getBlockTxs({
hash: '000000000000000015dc...'
});
console.log(blockTxs);
Returns a list of all txids in the block.
Parameters:
import { blocks } from 'esplora-js';
...
const blockTxids = await blocks.getBlockTxids({
hash: '000000000000000015dc...'
});
console.log(blockTxids);
Returns the transaction at index :index within the specified block.
Parameters:
import { blocks } from 'esplora-js';
...
const blockTxid = await blocks.getBlockTxid({
hash: '000000000000000015dc...',
index: 218
});
console.log(blockTxids);
Returns the raw block representation in binary.
Parameters:
import { blocks } from 'esplora-js';
...
const blockRaw = await blocks.getBlockRaw({
hash: '000000000000000015dc...'
});
console.log(blockRaw);
Returns the hash of the block currently at height.
Parameters:
import { blocks } from 'esplora-js';
...
const blockHeight = await blocks.getBlockHeader({
height: 66666,
});
console.log(blockHeight);
Returns the 10 newest blocks starting at the tip or at start_height if specified.
Parameters:
import { blocks } from 'esplora-js';
...
const getBlocks = await blocks.getBlocks({
start_height: 66666
});
console.log(getBlocks);
Returns the height of the last block.
Parameters:
import { blocks } from 'esplora-js';
...
const blocksTipHeight = await blocks.getBlocksTipHeight();
console.log(blocksTipHeight);
Returns the hash of the last block.
Parameters:
import { blocks } from 'esplora-js';
...
const blocksTipHash = await blocks.getBlocksTipHash();
console.log(blocksTipHash);
Returns information about the transaction.
Parameters:
import { transactions } from 'esplora-js';
...
const tx = await transactions.getTx({
txid: '15e10745f15593...'
});
console.log(tx);
Returns the transaction confirmation status.
Parameters:
import { transactions } from 'esplora-js';
...
const txStatus = await transactions.getTxStatus({
txid: '15e10745f15593...'
});
console.log(txStatus);
Returns the transaction hex.
Parameters:
import { transactions } from 'esplora-js';
...
const txHex = await transactions.getTxHex({
txid: '15e10745f15593...'
});
console.log(txHex);
Returns the raw transaction in hex or as binary data.
Parameters:
import { transactions } from 'esplora-js';
...
const txRaw = await transactions.getTxRaw({
txid: '15e10745f15593...'
});
console.log(txRaw);
Returns a merkle inclusion proof for the transaction using bitcoind's merkleblock format.
Parameters:
import { transactions } from 'esplora-js';
...
const txMerkleBlockProof = await transactions.getTxMerkleBlockProof({
txid: '15e10745f15593...'
});
console.log(txMerkleBlockProof);
Returns a merkle inclusion proof for the transaction using Electrum's blockchain.transaction.get_merkle format.
Parameters:
import { transactions } from 'esplora-js';
...
const txMerkleProof = await transactions.getTxMerkleProof({
txid: '15e10745f15593...'
});
console.log(txMerkleProof);
Returns the spending status of a transaction output.
Parameters:
import { transactions } from 'esplora-js';
...
const txOutspend = await transactions.getTxOutspend({
txid: '15e10745f15593...',
vout: 3,
});
console.log(txOutspend);
Returns the spending status of all transaction outputs.
Parameters:
import { transactions } from 'esplora-js';
...
const txOutspends = await transactions.getTxOutspends({
txid: '15e10745f15593...'
});
console.log(txOutspends);
Broadcast a raw transaction to the network. The transaction should be provided as hex in the request body. The txid will be returned on success.
Parameters:
import { transactions } from 'esplora-js';
...
const postTx = await transactions.postTx({
txid: '15e10745f15593...'
});
console.log(postTx);
Get information about an address.
Parameters:
import { addresses } from 'esplora-js';
...
const addressTest = await addresses.getAddress({
address: '15e10745f15593a...'
});
console.log(addressTest);
Get transaction history for the specified address/scripthash, sorted with newest first.
Parameters:
import { addresses } from 'esplora-js';
...
const addressTxs = await addresses.getAddressTxs({
address: '15e10745f15593a...'
});
console.log(addressTxs);
Get confirmed transaction history for the specified address/scripthash, sorted with newest first.
Parameters:
import { addresses } from 'esplora-js';
...
const addressTxsChain = await addresses.getAddressTxsChain({
address: '15e10745f15593a...'
});
console.log(addressTxsChain);
Get unconfirmed transaction history for the specified address/scripthash.
Parameters:
import { addresses } from 'esplora-js';
...
const addressTxsMempool = await addresses.getAddressTxsMempool({
address: '15e10745f15593a...'
});
console.log(addressTxsMempool);
Get the list of unspent transaction outputs associated with the address/scripthash.
Parameters:
import { addresses } from 'esplora-js';
...
const addressTxsUtxo = await addresses.getAddressTxsUtxo({
address: '15e10745f15593a...'
});
console.log(addressTxsUtxo);
Get information about an asset.
Parameters:
import { assets } from 'esplora-js';
...
const asset = await assets.getAsset({
asset_id: '6f0279e9ed04...'
});
console.log(asset);
Get information about an asset txs.
Parameters:
import { assets } from 'esplora-js';
...
const asset = await assets.getAssetTxs({
asset_id: `6f0279e9ed041c3d710a9f57d0c02928416460c4b722ae3457a11eec381c526d`,
});
console.log(asset);
Get information about an asset mempool txs.
Parameters:
import { assets } from 'esplora-js';
...
const asset = await assets.getAssetTxsMempool({
asset_id: `6f0279e9ed041c3d710a9f57d0c02928416460c4b722ae3457a11eec381c526d`,
});
console.log(asset);
Get information about an asset txs.
Parameters:
import { assets } from 'esplora-js';
...
const asset = await assets.getAssetTxsChain({
asset_id: `6f0279e9ed041c3d710a9f57d0c02928416460c4b722ae3457a11eec381c526d`,
});
console.log(asset);
Get the current total supply of the specified asset.
Parameters:
import { assets } from 'esplora-js';
...
const asset = await assets.getAssetSupply({
asset_id: `6f0279e9ed041c3d710a9f57d0c02928416460c4b722ae3457a11eec381c526d`,
});
console.log(asset);
Get the current total supply of the specified asset.
Parameters:
import { assets } from 'esplora-js';
...
const asset = await assets.getAssetSupplyDecimal({
asset_id: `6f0279e9ed041c3d710a9f57d0c02928416460c4b722ae3457a11eec381c526d`,
});
console.log(asset);
Get information about an asset mempool txs.
Parameters:
import { assets } from 'esplora-js';
...
const asset = await assets.getAssetRegistry();
console.log(asset);
Pull requests are welcome! For major changes, please open an issue first to discuss what you would like to change.
FAQs
NPM Package for blockstream.info API.
We found that esplora-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.