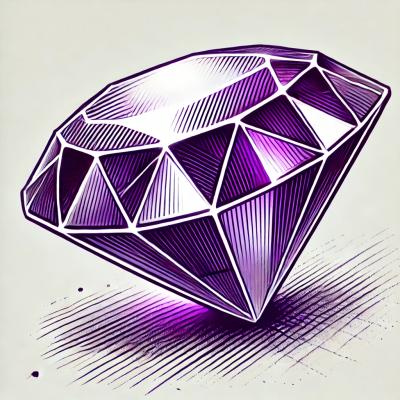
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
estree-walker
Advanced tools
The estree-walker package is a simple utility for walking an ESTree-compliant AST (Abstract Syntax Tree), such as those produced by parsers like Acorn or Esprima. It allows users to traverse the tree and manipulate nodes during the traversal.
Walking an AST
This feature allows you to traverse an AST, performing actions when entering and leaving each node. The 'enter' function is called when a node is entered, and the 'leave' function is called when a node is left during the traversal.
const { walk } = require('estree-walker');
const ast = { /* some AST object */ };
walk(ast, {
enter(node, parent, prop, index) {
// Perform actions upon entering a node
},
leave(node, parent, prop, index) {
// Perform actions upon leaving a node
}
});
Manipulating Nodes
This feature demonstrates how you can manipulate nodes during traversal. In this example, numeric literal values are doubled.
const { walk } = require('estree-walker');
const ast = { /* some AST object */ };
walk(ast, {
enter(node) {
if (node.type === 'Literal' && typeof node.value === 'number') {
node.value *= 2; // Double the number
}
}
});
Acorn-walk is a small, fast, JavaScript-based AST walker for the Acorn parser. It provides similar functionality to estree-walker but is specifically designed to work with the ASTs generated by Acorn.
Babel-traverse is part of the Babel toolchain and allows for traversing and manipulating Babel-generated ASTs. It offers a more complex API and additional features compared to estree-walker, such as scope tracking and path manipulation.
Recast provides AST traversal and manipulation capabilities, as well as source map support and pretty-printing of the modified code. It is more feature-rich than estree-walker and integrates with the Esprima parser.
Simple utility for walking an ESTree-compliant AST, such as one generated by acorn.
npm i estree-walker
var walk = require( 'estree-walker' ).walk;
var acorn = require( 'acorn' );
ast = acorn.parse( sourceCode, options ); // https://github.com/marijnh/acorn
walk( ast, {
enter: function ( node, parent ) {
// some code happens
},
leave: function ( node, parent ) {
// some code happens
}
});
Inside the enter
function, calling this.skip()
will prevent the node's children being walked, or the leave
function (which is optional) being called.
The ESTree spec is evolving to accommodate ES6/7. I've had a couple of experiences where estraverse was unable to handle an AST generated by recent versions of acorn, because it hard-codes visitor keys.
estree-walker, by contrast, simply enumerates a node's properties to find child nodes (and child lists of nodes), and is therefore resistant to spec changes. It's also much smaller. (The performance, if you're wondering, is basically identical.)
None of which should be taken as criticism of estraverse, which has more features and has been battle-tested in many more situations, and for which I'm very grateful.
MIT
0.5.2
FAQs
Traverse an ESTree-compliant AST
The npm package estree-walker receives a total of 18,440,469 weekly downloads. As such, estree-walker popularity was classified as popular.
We found that estree-walker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.