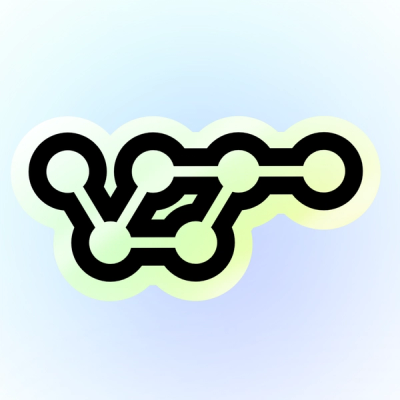
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
eth-block-tracker
Advanced tools
A block tracker for the Ethereum blockchain. Keeps track of the latest block.
The eth-block-tracker npm package is designed to help developers track the latest blocks on the Ethereum blockchain. It provides a simple interface to subscribe to new block events, poll for new blocks, and manage block tracking efficiently.
Polling for New Blocks
This feature allows you to poll for new blocks on the Ethereum blockchain. The code sample demonstrates how to set up a block tracker that listens for the latest block and logs it to the console.
const EthBlockTracker = require('eth-block-tracker');
const provider = require('eth-provider');
const blockTracker = new EthBlockTracker({ provider: provider() });
blockTracker.on('latest', (block) => {
console.log('Latest block:', block);
});
blockTracker.start();
Handling Errors
This feature allows you to handle errors that may occur during block tracking. The code sample demonstrates how to set up an error handler that logs errors to the console.
const EthBlockTracker = require('eth-block-tracker');
const provider = require('eth-provider');
const blockTracker = new EthBlockTracker({ provider: provider() });
blockTracker.on('error', (error) => {
console.error('Error:', error);
});
blockTracker.start();
Custom Polling Interval
This feature allows you to set a custom polling interval for checking new blocks. The code sample demonstrates how to set up a block tracker with a polling interval of 20 seconds.
const EthBlockTracker = require('eth-block-tracker');
const provider = require('eth-provider');
const blockTracker = new EthBlockTracker({ provider: provider(), pollingInterval: 20000 });
blockTracker.on('latest', (block) => {
console.log('Latest block:', block);
});
blockTracker.start();
The web3 package is a comprehensive library for interacting with the Ethereum blockchain. It includes functionality for tracking blocks, but also provides a wide range of other features such as contract interaction, account management, and more. Compared to eth-block-tracker, web3 is more feature-rich but may be overkill if you only need block tracking.
The ethers package is another popular library for interacting with the Ethereum blockchain. It offers similar functionalities to web3, including block tracking, but is known for its smaller size and better performance. Like web3, it provides a broader set of features beyond block tracking.
The ethereumjs-blockstream package is specifically designed for streaming Ethereum blocks. It provides a more focused approach to block tracking compared to web3 and ethers, making it a closer alternative to eth-block-tracker. However, it may not be as widely used or supported as the other two libraries.
This module walks the Ethereum blockchain, keeping track of the latest block. It uses a web3 provider as a data source and will continuously poll for the next block.
const createInfuraProvider = require('eth-json-rpc-infura')
const { PollingBlockTracker } = require('eth-block-tracker')
const provider = createInfuraProvider({ network: 'mainnet', projectId: process.env.INFURA_PROJECT_ID })
const blockTracker = new PollingBlockTracker({ provider })
blockTracker.on('sync', ({ newBlock, oldBlock }) => {
if (oldBlock) {
console.log(`sync #${Number(oldBlock)} -> #${Number(newBlock)}`)
} else {
console.log(`first sync #${Number(newBlock)}`)
}
})
creates a new block tracker with provider
as a data source and
pollingInterval
(ms) timeout between polling for the latest block.
If an Error is encountered when fetching blocks, it will wait retryTimeout
(ms) before attempting again.
If keepEventLoopActive
is false, in Node.js it will unref the polling timeout, allowing the process to exit during the polling interval. defaults to true
, meaning the process will be kept alive.
synchronous returns the current block. may be null
.
console.log(blockTracker.getCurrentBlock())
Asynchronously returns the latest block. if not immediately available, it will fetch one.
Tells the block tracker to ask for a new block immediately, in addition to its normal polling interval.
Useful if you received a hint of a new block (e.g. via tx.blockNumber
from getTransactionByHash
).
Will resolve to the new latest block when its done polling.
The latest
event is emitted for whenever a new latest block is detected.
This may mean skipping blocks if there were two created since the last polling period.
blockTracker.on('latest', (newBlock) => console.log(newBlock))
The sync
event is emitted the same as "latest" but includes the previous block.
blockTracker.on('sync', ({ newBlock, oldBlock }) => console.log(newBlock, oldBlock))
The error
event means an error occurred while polling for the latest block.
blockTracker.on('error', (err) => console.error(err))
FAQs
A block tracker for the Ethereum blockchain. Keeps track of the latest block.
The npm package eth-block-tracker receives a total of 265,058 weekly downloads. As such, eth-block-tracker popularity was classified as popular.
We found that eth-block-tracker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 13 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.