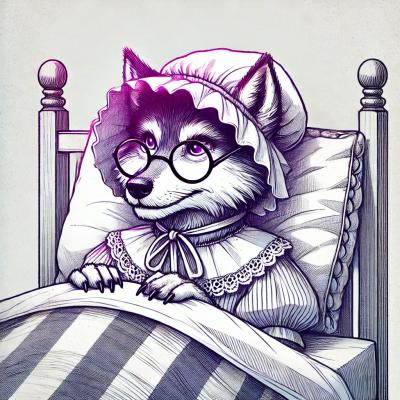
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Mathematical expression parser and evaluator It accepts string expression. Then it creates parsed object and and evaluate it.
It is parsing string, based on it, creates new expression and evaluating it.
Because parsing is complex, library is creating parsing object and when you need to use different variables with same expression it should be evaluated faster
User writes input like this
"3+k6+2*strength"
and it should be evaluated on backend server and should be secure and safe
import EvalExp from "evalexp";
// or
const { EvalExp } = require("evalexp");
const evalExp = new EvalExp("3k6 + 2strength");
evalExp.parse();
evalExp.evaluate({
k6: () => 3,
strength: 2
}); //return number 13
or if you plan to evaluate expression only once
EvalExp.evaluate("3k6+2strength", {
k6: () => 3,
strength: 2
}); //return number 13
or from function
import { evaluate } from "evalexp";
//or
const { evaluate } = require("evalexp");
evaluate("3k6+2strength", {
k6: () => 3,
strength: 2
});
const evalExp = new EvalExp("x+1");
const someInput = [1, 2, 3, 4, 5, 6, 7];
someInput.map(x => evalExp.evaluate({ x })) // [2, 3, 4, 5, 6, 7, 8])
EvalExp.evaluate("-5");
EvalExp.evaluate("-5*3");
EvalExp.evaluate("5");
EvalExp.evaluate("+5");
EvalExp.evaluate("4.345");
EvalExp.evaluate("5+4");
EvalExp.evaluate("+4+34");
EvalExp.evaluate("56.43+32");
EvalExp.evaluate("34-21");
EvalExp.evaluate("33-44");
EvalExp.evaluate("3^2"); //9
EvalExp.evaluate("(-3)^2"); //9
EvalExp.evaluate("9^0.5"); //3
EvalExp.evaluate("27^(1/3)"); //3
EvalExp.evaluate("6%2"); //0
EvalExp.evaluate("7%2"); //1
EvalExp.evaluate("5*5");
EvalExp.evaluate("-3*8");
EvalExp.evaluate("10/2");
EvalExp.evaluate("5*(3+(5-3)/4)");
multiplication operator can be ignored
EvalExp.evaluate("3(10+45)");
EvalExp.evaluate("3*someVariable");
multiplication operator can be ignored
EvalExp.evaluate("3someVariable");
EvalExp.evaluate("3*someFunctions");
multiplication operator can be ignored
EvalExp.evaluate("3someFunctions");
EvalExp.evaluate("3*someFunctions(10)");
complex arguments with inner functions are supported
EvalExp.evaluate("2+funcA(2*funcB(3+4, 4))");
This library does not give access to your global variables. In execute method all possible variables should be declared. If not declared variable is used in expression it will throw error. If you declare function and use it as variable (without bracked) it will be execeuted as zero argument function
variable varA is used in expression and is declated in evaluate function:
import EvalExp from "evalexp";
const evalExp = new EvalExp("10 + (varA + 5) * 2");
evalExp.parse();
evalExp.evaluate({
varA: 3
});
varA is declared as function and because of it, it will be executed as function in expression
import EvalExp from "evalexp";
const evalExp = new EvalExp("10 + (varA + 5) * 2");
evalExp.parse();
evalExp.evaluate({
varA() {
return 5;
}
});
This library by design does not give any predefined functions. It can be easily extended with any function you want
EvalExp.evaluate("IF(1, 2, 3)", {
IF: (condition, isTrue, isFalse) => (condition ? isTrue : isFalse)
});
EvalExp.evaluate("POW(2, 2)", {
POW: (arg1, arg) => Math.pow(arg1, arg)
});
FAQs
Mathematical expression parser and evaluator
We found that evalexp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.