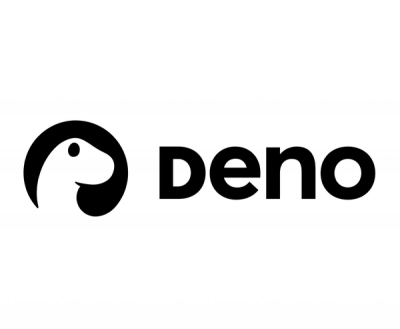
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
event-handle
Advanced tools
JavaScript event pattern with closures. (Built with Typescript!)
npm install event-handle
or yarn add event-handle
import EventHandle from 'event-handle'; // ES6
import { // ES6 Functional Import
createEventHandle,
isEventHandle,
onEvent,
} from 'event-handle';
const EventHandle = require('event-handle'); // CommonJS / Node.js
// Create an event.
let demoStarted = EventHandle.create();
// Create a handler.
EventHandle.on(demoStarted, (...args) => {
console.log('The demo started!', args)
}); // options: , { prepend: false, once: false }
// Create another handler.
let remove = demoStarted.handle((...args) => {
console.log('Got it...', args);
});
// Trigger the event, calling all handlers.
demoStarted('a','r','g','s');
// Remove the handler.
console.log('removed: ', remove());
// Other functions:
console.log('handlerCount: ', demoStarted.handlerCount());
console.log('id: ', demoStarted.id); // defined if create was passed an id.
console.log('isEventHandle: ', EventHandle.isEventHandle(demoStarted));
console.log('removeAllHandlers: ', demoStarted.removeAllHandlers());
See also:
spec/index.spec.ts
examples/
Events with closures.
function
Returns a function that triggers an event. The function has members such
as .handle()
to add a handler, .id
(a string), .handlerCount()
and
.removeAllHandlers()
.
boolean
Returns true if fn
is a function created by createEventHandle
.
function
Adds a handler to the given event.
Events with closures.
Kind: global variable
function
boolean
function
function
Returns a function that triggers an event. The function has members such
as .handle()
to add a handler, .id
(a string), .handlerCount()
and
.removeAllHandlers()
.
Kind: static property of EventHandle
Returns: function
- The event.
Param | Type | Description |
---|---|---|
[config] | EventHandleConfiguration | string | Event id or configuration. |
boolean
Returns true if fn
is a function created by createEventHandle
.
Kind: static property of EventHandle
Returns: boolean
- True if the given fn is an EventHandle.
Param | Type | Description |
---|---|---|
fn | function | The function to check. |
function
Adds a handler to the given event.
Kind: static property of EventHandle
Returns: function
- A function to remove the handler.
Param | Type | Description |
---|---|---|
evt | function | The event to add a handler to. |
handler | function | The handler function for the event. |
[options] | EventHandlerOptions | Options for the handler. |
function
Returns a function that triggers an event. The function has members such
as .handle()
to add a handler, .id
(a string), .handlerCount()
and
.removeAllHandlers()
.
Kind: global function
Returns: function
- The event.
Param | Type | Description |
---|---|---|
[config] | EventHandleConfiguration | string | Event id or configuration. |
boolean
Returns true if fn
is a function created by createEventHandle
.
Kind: global function
Returns: boolean
- True if the given fn is an EventHandle.
Param | Type | Description |
---|---|---|
fn | function | The function to check. |
function
Adds a handler to the given event.
Kind: global function
Returns: function
- A function to remove the handler.
Param | Type | Description |
---|---|---|
evt | function | The event to add a handler to. |
handler | function | The handler function for the event. |
[options] | EventHandlerOptions | Options for the handler. |
FAQs
Events with closures.
The npm package event-handle receives a total of 0 weekly downloads. As such, event-handle popularity was classified as not popular.
We found that event-handle demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.