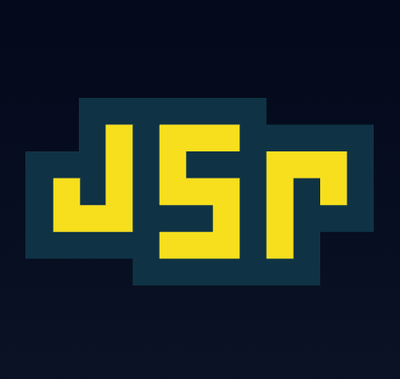
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
eventsource-parser
Advanced tools
The eventsource-parser npm package is a utility for parsing Server-Sent Events (SSE) streams. It allows you to handle real-time data streams from servers efficiently by parsing the incoming data into manageable events.
Parsing SSE Streams
This feature allows you to parse incoming SSE data streams. The `createParser` function initializes a parser that processes incoming data and triggers a callback for each event.
const { createParser } = require('eventsource-parser');
const parser = createParser((event) => {
if (event.type === 'event') {
console.log('Received event:', event);
}
});
const sseData = `data: Hello\n\n`;
parser.feed(sseData);
Handling Different Event Types
This feature allows you to handle different types of events, such as comments and data events. The parser can differentiate between these types and process them accordingly.
const { createParser } = require('eventsource-parser');
const parser = createParser((event) => {
if (event.type === 'event') {
console.log('Received event:', event);
} else if (event.type === 'comment') {
console.log('Received comment:', event);
}
});
const sseData = `: This is a comment\ndata: Hello\n\n`;
parser.feed(sseData);
Error Handling
This feature demonstrates how to handle errors that may occur during the parsing of SSE data. The parser can throw errors if the data is not in the correct format, and you can catch these errors to handle them gracefully.
const { createParser } = require('eventsource-parser');
const parser = createParser((event) => {
if (event.type === 'event') {
console.log('Received event:', event);
}
});
try {
const invalidSseData = `data: Hello\ninvalid\n`;
parser.feed(invalidSseData);
} catch (error) {
console.error('Error parsing SSE data:', error);
}
The 'eventsource' package is a polyfill for the EventSource API, which is used to receive server-sent events. Unlike eventsource-parser, which focuses on parsing SSE streams, 'eventsource' provides a full implementation of the EventSource interface, including connection management and event handling.
The 'sse-channel' package is designed for creating SSE channels on the server side. It allows you to manage multiple clients and broadcast events to them. While eventsource-parser is focused on parsing incoming SSE data, 'sse-channel' is more about managing and sending SSE data from the server.
The 'express-sse' package is a simple SSE implementation for Express.js. It allows you to easily set up SSE endpoints in an Express application. Unlike eventsource-parser, which is a low-level parser, 'express-sse' provides higher-level abstractions for integrating SSE into web applications.
A streaming parser for server-sent events/eventsource, without any assumptions about how the actual stream of data is retrieved. It is intended to be a building block for clients and polyfills in javascript environments such as browsers, node.js and deno.
You create an instance of the parser, and feed it chunks of data - partial or complete, and the parse emits parsed messages once it receives a complete message.
npm install --save eventsource-parser
import {createParser, ParsedEvent, ReconnectInterval} from 'eventsource-parser'
function onParse(event: ParsedEvent | ReconnectInterval) {
if (event.type === 'event') {
console.log('Received event!')
console.log('id: %s', event.id || '<none>')
console.log('name: %s', event.name || '<none>')
console.log('data: %s', event.data)
} else if (event.type === 'reconnect-interval') {
console.log('We should set reconnect interval to %d milliseconds', event.value)
}
}
const parser = createParser(onParse)
const sseStream = getSomeReadableStream()
for await (const chunk of sseStream) {
parser.feed(chunk)
}
// If you want to re-use the parser for a new stream of events, make sure to reset it!
parser.reset()
console.log('Done!')
MIT © Espen Hovlandsdal
FAQs
Streaming, source-agnostic EventSource/Server-Sent Events parser
We found that eventsource-parser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.