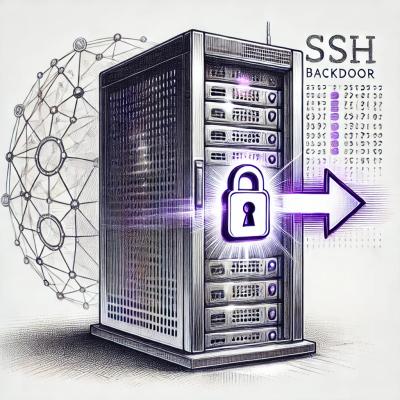
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
evt-promise
Advanced tools
An event-driven promise-like function wrapper, has a different api through the ES6 Promise
npm install evt-promise
BASIC:
const async = require('evt-async');
//Simple usage
var foo = async(function() {return true;});
foo.exec(function(value){console.log(value);},function(e) {throw e;}); //Will print true
//Catch errors
var bar = async(function() {throw new Error('TEST');});
bar.exec(function(v){}, function(e){console.error(e.message);}); //Will print 'TEST' at stderr
//Pass arguments
var baz = async(function(arg) {return arg;},[true]);
baz.exec(function(value){console.log(value);},function(e) {throw e;}); //Will print true
Advanced - Change the task that you want to run, Change the arguments that you want to pass to the task function:
const async = require('evt-async');
//Change the task
var foo = async(function() {return true});
foo.task = function() {
return false;
}
foo.exec(function(value){console.log(value);},function(e) {throw e;}); //Will print false, not true
//Change the arguments
var bar = async(function(arg) {return arg;},[true]);
bar.args = [false];
bar.exec(function(value){console.log(value);},function(e) {throw e;}); //Will print false not true
Advanced - Emit custom events in the task and handle it
const async = require('evt-async');
var fn = function() {
this.emit('custom','custom event'); //The this object is an instance of eventemitter2, injected by the apply() function
return 'hello custom';
};
var resHandler = function(value) {
console.log(value);
};
var rejHandler = function(e) {
throw e;
};
var foo = async(fn)
foo.event.on('custom', function(value) {console.log(value);});
foo.exec(resHandler,rejHandler); //Will print 'custom event' and then 'hello coustom'
Warning: If you use arrow function as the task, this example won't work because you cannot inject a this object to an arrow function
FAQs
An event-driven promise-like function wrapper
We found that evt-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.