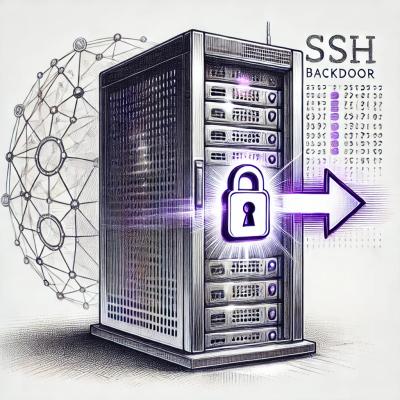
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
This is a simple npm package example based on How to Write an Open Source JavaScript Library course from egghead.io
npm set init-author-name 'Your Name'
npm set init-author-email 'your.email@yourdomain.com'
npm set init-author-url 'yourdomain.com'
npm set init-license 'MIT'
npm set save-exact true
signup for npm account (if you don't have already): npmjs.com
npm adduser
npm init
npm publish
git tag 1.0.0
git push --tags
Semantic versioning is a standard that a lot of projects use to communicate what kinds of changes are in this release. It's important to communicate what kinds of changes are in a release because sometimes those changes will break the code that depends on the package.
If a project is going to be shared with others, it should start at 1.0.0, though some projects on npm don't follow this rule.
After this, changes should be handled as follows:
Update version number in package.json file, commit your changes to github and add new tag and then run:
npm publish
Update version number to for example: 1.1.0-beta.0, commit your changes to github and add new tag and then run:
npm publish --tag beta
To install this beta verion you can run:
npm install your-package-name@beta
Install mocha and chai:
npm i -D mocha chai
To package.json add:
"scripts": {
"test": "mocha src/index.test.js"
},
To run test:
npm run test
npm install -g semantic-release-cli
semantic-release-cli setup
in .travis.yml add:
script:
- npm run test
npm install -D commitizen cz-conventional-changelog
Add following lines to package.json:
"scripts": {
"commit": "git-cz",
"czConfig": {
"path": "node_modules/cz-conventional-changelog"
}
Now you can commit yourchanges using:
npm run commit
In "List any breaking changes or issues closed by this change:" step you can use:
closes #1
if your update closes any existing issue
npm i -D ghooks
Update package.json to include:
"config": {
"ghooks": {
"pre-commit": "npm run test"
}
}
npm install -D istanbul
update package.json to include:
"scripts": {
"test": "istanbul cover -x *.test.js ./node_modules/mocha/bin/_mocha -- -R spec src/index.test.js",
update package.json to include:
"scripts": {
"check-coverage": "istanbul check-coverage --statements 100 --branches 100 --functions 100 --lines 100",
and
"config": {
"ghooks": {
"pre-commit": "npm run test && npm run check-coverage"
update .travis.yml to include:
script:
- npm run test
- npm run check-coverage
signup for codecov.io account (if you don't have already)
npm install -D codecov.io
update package.json to include:
"scripts": {
"report-coverage": "cat ./coverage/lcov.info | codecov",
update .travis.yml to include:
after_success:
- npm run report-coverage
You can go to shields.io and use their banges for example to create something like this:
use this code:
[](https://travis-ci.org/creativedeveloper-net/npm-package-example)
[](https://codecov.io/github/creativedeveloper-net/npm-package-example)
[](http://npm.im/npm-package-example)
[](http://npm-stat.com/charts.html?package=npm-package-example&from=2015-08-01)
[](http://opensource.org/licenses/MIT)
npm i -D babel-cli
npm i -D rimraf
npm i -D babel-preset-es2015 babel-preset-stage-2
update package.json to include:
"scripts": {
"prebuild": "rimraf dist",
"build": "babel --copy-files --out-dir dist --ignore *.test.js src",
and
"babel": {
"presets": ["es2015", "stage-2"]
}
and also update "main" section to use dist folder instead of src:
"main": "dist/index.js",
update .travis.yml to include:
script:
- npm run test
- npm run check-coverage
- npm run build
add "files" section to package.json to include only necessary files:
"files": [
"dist",
"README.md"
],
npm i -D nyc
update package.json "script" section:
"check-coverage": "nyc check-coverage --statements 100 --branches 100 --functions 100 --lines 100",
"report-coverage": "cat ./coverage/lcov.info | codecov",
"watch:test": "npm t -- --watch",
"test": "mocha src/index.test.js --compilers js:babel-core/register",
"cover": "nyc npm t",
and "ghooks" section:
"ghooks": {
"pre-commit": "npm run cover && npm run check-coverage"
},
update .travis.yml "script" section:
script:
- npm run cover
- npm run check-coverage
- npm run build
update .travis.yml to include:
branches:
only:
- master
npm i -D webpack
Add new file C:\Users\sciurkat\Desktop\My files\webpack.config.babel.js with this code:
import {join} from 'path'
const include = join(__dirname, 'src')
export default {
entry: './src/index',
output: {
path: join(__dirname, 'dist'),
libraryTarget: 'umd',
library: 'npmPackageExample',
},
devtool: 'source-map',
module: {
loaders: [
{test: /\.js$/, loader: 'babel', include},
{test: /\.json$/, 'loader': 'json', include},
]
}
}
install
npm i -D babel-loader json-loader
npm install npm-run-all --save-dev
update package.json "script" section:
"build": "npm-run-all --parallel build:*",
"build:main": "babel --copy-files --out-dir dist --ignore *.test.js src",
"build:umd": "webpack --output-filename index.umd.js",
"build:umd.min": "webpack --output-filename index.umd.min.js -p",
You can now access your package via url like this: https://unpkg.com/npm-package-example/dist/index.umd.js
https://docs.travis-ci.com/user/sonarqube/
generate token for sonarqube: https://sonarqube.com/account/security/
encrypt that token here: https://travis-encrypt.github.io/
update .travis.yml to inlcude:
script:
- npm run cover
- npm run check-coverage
- npm run build
- sonar-scanner -Dsonar.login=$SONAR_TOKEN -X
add sonar-project.properties file with code like this (update your projectKey and projectName):
sonar.projectKey=creativedeveloper.net:npm-package-example
sonar.projectName=creativedeveloper.net::npm-package-example
sonar.projectVersion=1.0
sonar.language=js
sonar.sources=src/index.js
sonar.sourceEncoding=UTF-8
sonar.tests=src/index.test.js
sonar.javascript.lcov.reportPath=coverage/lcov.info
install jshint
npm install jshint -g
update package.json:
"scripts": {
"check-jshint": "jshint src/index.js",
and:
"config": {
"ghooks": {
"pre-commit": "npm run cover && npm run check-coverage && npm run check-jshint"
}
},
FAQs
This is a simple npm package example
We found that example-ci demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.