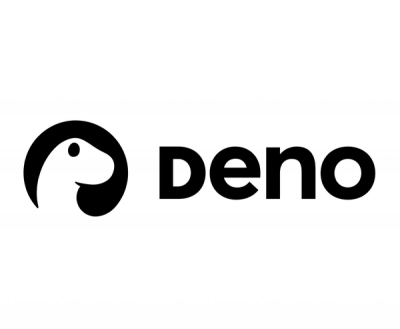
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The exceljs npm package is a comprehensive library for reading, writing, and manipulating Excel files in various formats such as XLSX, CSV, and more. It provides a wide range of functionalities to work with Excel documents programmatically, including creating new sheets, styling cells, adding formulas, and handling large datasets efficiently.
Reading Excel Files
This feature allows you to read existing Excel files. You can iterate through rows and cells, access values, and perform operations based on the data.
{"const ExcelJS = require('exceljs');
const workbook = new ExcelJS.Workbook();
workbook.xlsx.readFile('path/to/file.xlsx')
.then(() => {
const worksheet = workbook.getWorksheet('Sheet1');
worksheet.eachRow({ includeEmpty: true }, (row, rowNumber) => {
console.log('Row ' + rowNumber + ' = ' + JSON.stringify(row.values));
});
});"}
Writing Excel Files
This feature enables you to create new Excel files or modify existing ones. You can add worksheets, rows, and cells with data, and save the file to the disk.
{"const ExcelJS = require('exceljs');
const workbook = new ExcelJS.Workbook();
const sheet = workbook.addWorksheet('My Sheet');
sheet.addRow(['Name', 'Profession']);
sheet.addRow(['John Doe', 'Developer']);
workbook.xlsx.writeFile('path/to/newfile.xlsx');"}
Styling Cells
This feature allows you to apply various styles to cells, such as fonts, colors, borders, and fills. It helps in making the data more readable and visually appealing.
{"const ExcelJS = require('exceljs');
const workbook = new ExcelJS.Workbook();
const sheet = workbook.addWorksheet('My Sheet');
const row = sheet.addRow(['Name', 'Profession']);
row.getCell(1).font = { bold: true };
row.getCell(2).fill = {
type: 'pattern',
pattern: 'solid',
fgColor: { argb: 'FFFF0000' }
};
workbook.xlsx.writeFile('path/to/styledfile.xlsx');"}
Adding Formulas
This feature lets you insert formulas into cells. You can also pre-calculate the result of the formula and store it in the cell.
{"const ExcelJS = require('exceljs');
const workbook = new ExcelJS.Workbook();
const sheet = workbook.addWorksheet('My Sheet');
const row = sheet.addRow([100, 200, { formula: 'A1+B1', result: 300 }]);
workbook.xlsx.writeFile('path/to/formulafile.xlsx');"}
Handling Large Data Sets
This feature is particularly useful for handling large datasets without running out of memory. It streams the data to the file system as it's being processed.
{"const ExcelJS = require('exceljs');
const workbook = new ExcelJS.stream.xlsx.WorkbookWriter({ filename: 'path/to/largefile.xlsx' });
const sheet = workbook.addWorksheet('My Sheet');
for (let i = 0; i < 1000000; i++) {
sheet.addRow(['Row ' + i, 'Data']);
}
workbook.commit();"}
The 'xlsx' package is another popular library for parsing and writing various spreadsheet formats. It is known for its simplicity and small bundle size, but it may not offer as many features for styling and manipulating data as exceljs.
SheetJS, also known as 'xlsx', is a powerful and comprehensive library that supports a wide range of spreadsheet formats. It is similar to exceljs in functionality but differs in API design and implementation details.
The 'node-xlsx' package is a simpler alternative for parsing and building XLSX/CSV files. It focuses on basic functionality and is easier to use for simple tasks, but lacks the advanced features and fine control provided by exceljs.
Read, manipulate and write spreadsheet data and styles to XLSX and JSON.
Reverse engineered from Excel spreadsheet files as a project.
npm install exceljs
var Excel = require("exceljs");
var workbook = new Excel.Workbook();
var sheet = workbook.addWorksheet("My Sheet");
// Iterate over all sheets
// Note: workbook.worksheets.forEach will still work but this is better
workbook.eachSheet(function(worksheet, sheetId) {
// ...
});
// fetch sheet by name
var worksheet = workbook.getWorksheet("My Sheet");
// fetch sheet by id
var worksheet = workbook.getWorksheet(1);
// Add column headers and define column keys and widths
// Note: these column structures are a workbook-building convenience only,
// apart from the column width, they will not be fully persisted.
worksheet.columns = [
{ header: "Id", key: "id", width: 10 },
{ header: "Name", key: "name", width: 32 },
{ header: "D.O.B.", key: "DOB", width: 10 }
];
// Access an individual columns by key, letter and 1-based column number
var idCol = worksheet.getColumn("id");
var nameCol = worksheet.getColumn("B");
var dobCol = worksheet.getColumn(3);
// set column properties
dobCol.header = "Date of Birth"; // Note: will overwrite cell value C1
dobCol.header = ["Date of Birth", "A.K.A. D.O.B."]; // Note: this will overwrite cell values C1:C2
dobCol.key = "dob"; // from this point on, this column will be indexed by "dob" and not "DOB"
dobCol.width = 15;
// Add a couple of Rows by key-value, after the last current row, using the column keys
worksheet.addRow({id: 1, name: "John Doe", dob: new Date(1970,1,1)});
worksheet.addRow({id: 2, name: "Jane Doe", dob: new Date(1965,1,7)});
// Add a row by contiguous Array (assign to columns A, B & C)
worksheet.addRow([3, "Sam", new Date()]);
// Add a row by sparse Array (assign to columns A, E & I)
var rowValues = [];
rowValues[1] = 4;
rowValues[5] = "Kyle";
rowValues[9] = new Date();
worksheet.addRow(rowValues);
// Get a row object. If it doesn't already exist, a new empty one will be returned
var row = worksheet.getRow(5);
// Set a specific row height
row.height = 42.5;
row.getCell(1).value = 5; // A5's value set to 5
row.getCell("name").value = "Zeb"; // B5's value set to "Zeb" - assuming column 2 is still keyed by name
row.getCell("C").value = new Date(); // C5's value set to now
// Get a row as a sparse array
// Note: interface change: worksheet.getRow(4) ==> worksheet.getRow(4).values
row = worksheet.getRow(4).values;
expect(row[5]).toEqual("Kyle");
// assign row values by contiguous array (where array element 0 has a value)
row.values = [1,2,3];
expect(row.getCell(1).value).toEqual(1);
expect(row.getCell(2).value).toEqual(2);
expect(row.getCell(3).value).toEqual(3);
// assign row values by sparse array (where array element 0 is undefined)
var values = []
values[5] = 7;
values[10] = "Hello, World!";
row.values = values;
expect(row.getCell(1).value).toBeNull();
expect(row.getCell(5).value).toEqual(7);
expect(row.getCell(10).value).toEqual("Hello, World!");
// assign row values by object, using column keys
row.values = {
id: 13,
name: "Thing 1",
dob: new Date()
};
// Iterate over all rows that have values in a worksheet
// Note: interface change - argument order is now row, rowNumber
worksheet.eachRow(function(row, rowNumber) {
console.log("Row " + rowNumber + " = " + JSON.stringify(row.values));
});
// Iterate over all non-null cells in a row
row.eachCell(function(cell, colNumber) {
console.log("Cell " + colNumber + " = " + cell.value);
});
## Handling Individual Cells
```javascript
// Modify/Add individual cell
worksheet.getCell("C3").value = new Date(1968, 5, 1);
// query a cell's type
expect(worksheet.getCell("C3").type).toEqual(Excel.ValueType.Date);
// merge a range of cells
worksheet.mergeCells("A4:B5");
// merge by top-left, bottom-right
worksheet.mergeCells("G10", "H11");
worksheet.mergeCells(10,11,12,13); // top,left,bottom,right
// ... merged cells are linked
worksheet.getCell("B5").value = "Hello, World!";
expect(worksheet.getCell("A4").value).toBe(worksheet.getCell("B5").value);
expect(worksheet.getCell("A4")).toBe(worksheet.getCell("B5").master);
// display value as "1 3/5"
ws.getCell("A1").value = 1.6;
ws.getCell("A1").numFmt = "# ?/?";
// display value as "1.60%"
ws.getCell("B1").value = 0.016;
ws.getCell("B1").numFmt = "0.00%";
// for the wannabe graphic designers out there
ws.getCell("A1").font = {
name: "Comic Sans MS",
family: 4,
size: 16,
underline: true,
bold: true
};
// for the graduate graphic designers...
ws.getCell("A2").font = {
name: "Arial Black",
color: { argb: "FF00FF00" },
family: 2,
size: 14,
italic: true
};
// note: the cell will store a reference to the font object assigned.
// If the font object is changed afterwards, the cell font will change also...
var font = { name: "Arial", size: 12 };
ws.getCell("A3").font = font;
font.size = 20; // Cell A3 now has font size 20!
// Cells that share similar fonts may reference the same font object after
// the workbook is read from file or stream
Font Property | Description | Example Value(s) |
---|---|---|
name | Font name. | "Arial", "Calibri", etc. |
family | Font family. An integer value. | 1,2,3, etc. |
scheme | Font scheme. | "minor", "major", "none" |
charset | Font charset. An integer value. | 1, 2, etc. |
color | Colour description, an object containing an ARGB value. | { argb: "FFFF0000"} |
bold | Font weight | true, false |
italic | Font slope | true, false |
underline | Font underline style | true, false, "none", "single", "double", "singleAccounting", "doubleAccounting" |
strike | Font | true, false |
outline | Font outline | true, false |
// set cell alignment to top-left, middle-center, bottom-right
ws.getCell("A1").alignment = { vertical: "top", horizontal: "left" };
ws.getCell("B1").alignment = { vertical: "middle", horizontal: "center" };
ws.getCell("C1").alignment = { vertical: "bottom", horizontal: "right" };
// set cell to wrap-text
ws.getCell("D1").alignment = { wrapText: true };
// set cell indent to 1
ws.getCell("E1").alignment = { indent: 1 };
// set cell text rotation to 30deg upwards, 45deg downwards and vertical text
ws.getCell("F1").alignment = { textRotation: 30 };
ws.getCell("G1").alignment = { textRotation: -45 };
ws.getCell("H1").alignment = { textRotation: "vertical" };
Valid Alignment Property Values
horizontal | vertical | wrapText | indent | readingOrder | textRotation |
---|---|---|---|---|---|
left | top | true | integer | rtl | 0 to 90 |
center | middle | false | ltr | -1 to -90 | |
right | bottom | vertical | |||
fill | distributed | ||||
justify | justify | ||||
centerContinuous | |||||
distributed |
// set single thin border around A1 ws.getCell("A1").border = { top: {style:"thin"}, left: {style:"thin"}, bottom: {style:"thin"}, right: {style:"thin"} };
// set double thin green border around A3 ws.getCell("A3").border = { top: {style:"double", color: {argb:"FF00FF00"}}, left: {style:"double", color: {argb:"FF00FF00"}}, bottom: {style:"double", color: {argb:"FF00FF00"}}, right: {style:"double", color: {argb:"FF00FF00"}} };
// set thick red cross in A5 ws.getCell("A5").border = { diagonal: {up: true, down: true, style:"thick", color: {argb:"FFFF0000"}} };
Valid Border Styles
// read from a file
var workbook = new Excel.Workbook();
workbook.xlsx.readFile(filename)
.then(function() {
// use workbook
});
// pipe from stream
var workbook = new Excel.Workbook();
stream.pipe(workbook.xlsx.createInputStream());
// write to a file
var workbook = createAndFillWorkbook();
workbook.xlsx.writeFile(filename)
.then(function() {
// done
});
// write to a stream
workbook.xlsx.write(stream)
.then(function() {
// done
});
The following value types are supported.
Enum Name | Enum(*) | Description | Example Value |
---|---|---|---|
Excel.ValueType.Null | 0 | No value. | null |
Excel.ValueType.Merge | 1 | N/A | N/A |
Excel.ValueType.Number | 2 | A numerical value | 3.14 |
Excel.ValueType.String | 3 | A text value | "Hello, World!" |
Excel.ValueType.Date | 4 | A Date value | new Date() |
Excel.ValueType.Hyperlink | 5 | A hyperlink | { text: "www.mylink.com", hyperlink: "http://www.mylink.com" } |
Excel.ValueType.Formula | 6 | A formula | { formula: "A1+A2", result: 7 } |
Version | Changes |
---|---|
0.0.9 | |
0.1.0 |
|
0.1.1 |
|
0.1.2 |
|
0.1.3 |
|
0.1.4 |
|
Every effort is made to make a good consistent interface that doesn't break through the versions but regrettably, now and then some things have to change for the greater good.
The arguments in the callback function to Worksheet.eachRow have been swapped and changed; it was function(rowNumber,rowValues), now it is function(row, rowNumber) which gives it a look and feel more like the underscore (_.each) function and prioritises the row object over the row number.
This function has changed from returning a sparse array of cell values to returning a Row object. This enables accessing row properties and will facilitate managing row styles and so on.
The sparse array of cell values is still available via Worksheet.getRow(rowNumber).values;
cell.styles renamed to cell.style
FAQs
Excel Workbook Manager - Read and Write xlsx and csv Files.
The npm package exceljs receives a total of 1,933,308 weekly downloads. As such, exceljs popularity was classified as popular.
We found that exceljs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.