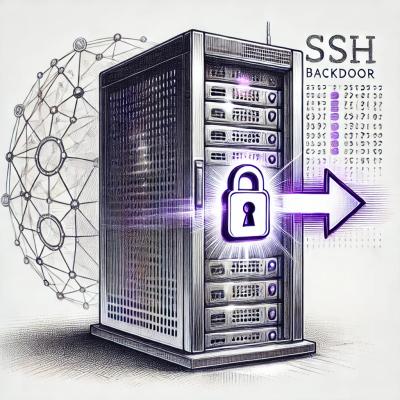
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
expo-camera
Advanced tools
The expo-camera package is a part of the Expo ecosystem and provides a comprehensive API for accessing and using the device's camera in React Native applications. It allows developers to capture photos, record videos, and access various camera settings and features.
Capture Photos
This feature allows you to capture photos using the device's camera. The code sample demonstrates how to set up the Camera component and take a picture when a button is pressed.
```javascript
import React, { useRef } from 'react';
import { View, Button } from 'react-native';
import { Camera } from 'expo-camera';
export default function App() {
const cameraRef = useRef(null);
const takePicture = async () => {
if (cameraRef.current) {
const photo = await cameraRef.current.takePictureAsync();
console.log(photo);
}
};
return (
<View style={{ flex: 1 }}>
<Camera style={{ flex: 1 }} ref={cameraRef} />
<Button title="Take Picture" onPress={takePicture} />
</View>
);
}
```
Record Videos
This feature allows you to record videos using the device's camera. The code sample demonstrates how to set up the Camera component and start/stop video recording when a button is pressed.
```javascript
import React, { useRef, useState } from 'react';
import { View, Button } from 'react-native';
import { Camera } from 'expo-camera';
export default function App() {
const cameraRef = useRef(null);
const [isRecording, setIsRecording] = useState(false);
const recordVideo = async () => {
if (cameraRef.current) {
if (isRecording) {
cameraRef.current.stopRecording();
} else {
setIsRecording(true);
const video = await cameraRef.current.recordAsync();
console.log(video);
setIsRecording(false);
}
}
};
return (
<View style={{ flex: 1 }}>
<Camera style={{ flex: 1 }} ref={cameraRef} />
<Button title={isRecording ? "Stop Recording" : "Record Video"} onPress={recordVideo} />
</View>
);
}
```
Access Camera Settings
This feature allows you to access and modify camera settings such as switching between the front and back cameras. The code sample demonstrates how to request camera permissions and toggle the camera type.
```javascript
import React, { useState, useEffect } from 'react';
import { View, Text } from 'react-native';
import { Camera } from 'expo-camera';
export default function App() {
const [hasPermission, setHasPermission] = useState(null);
const [cameraType, setCameraType] = useState(Camera.Constants.Type.back);
useEffect(() => {
(async () => {
const { status } = await Camera.requestPermissionsAsync();
setHasPermission(status === 'granted');
})();
}, []);
if (hasPermission === null) {
return <View />;
}
if (hasPermission === false) {
return <Text>No access to camera</Text>;
}
return (
<View style={{ flex: 1 }}>
<Camera style={{ flex: 1 }} type={cameraType} />
<Button
title="Flip Camera"
onPress={() => {
setCameraType(
cameraType === Camera.Constants.Type.back
? Camera.Constants.Type.front
: Camera.Constants.Type.back
);
}}
/>
</View>
);
}
```
The react-native-camera package is a popular alternative to expo-camera. It provides similar functionalities such as capturing photos, recording videos, and accessing camera settings. However, it requires linking and additional setup compared to the more streamlined experience of expo-camera within the Expo ecosystem.
The react-native-vision-camera package offers advanced camera functionalities, including frame processing and integration with machine learning models. It is more feature-rich compared to expo-camera but also requires more complex setup and configuration.
If you're using Cocoapods, add the dependency to your Podfile
:
pod 'EXCamera'
and run pod install
.
Libraries
➜ Add Files to [your project's name]
node_modules
➜ expo-camera
and add EXCamera.xcodeproj
libEXCamera.a
to your project's Build Phases
➜ Link Binary With Libraries
Cmd+R
).android/settings.gradle
:
include ':expo-camera'
project(':expo-camera').projectDir = new File(rootProject.projectDir, '../node_modules/expo-camera/android')
and if not already included
include ':expo-permissions-interface'
project(':expo-permissions-interface').projectDir = new File(rootProject.projectDir, '../node_modules/expo-permissions-interface/android')
android/app/build.gradle
:
compile project(':expo-camera')
and if not already included
compile project(':expo-permissions-interface')
A React component that renders a preview for the device's either front or back camera. Camera's parameters like zoom, auto focus, white balance and flash mode are adjustable. With use of Camera
one can also take photos and record videos that are saved to the app's cache. Morever, the component is also capable of detecting faces and bar codes appearing on the preview.
Note: Only one Camera preview is supported by this library right now. When using navigation, the best practice is to unmount previously rendered
Camera
component so next screens can use camera without issues.
Note: Android devices can use one of two available Camera apis underneath. This was previously chosen automatically, based on the device's Android system version and camera hardware capabilities. As we experienced some issues with Android's Camera2 API, we decided to choose the older API as a default. However, using the newer one is still possible through setting
useCamera2Api
prop to true. The change we made should be barely visible - the only thing that is not supported using the old Android's API is setting focus depth.
Requires Permissions.CAMERA
. Video recording requires Permissions.AUDIO_RECORDING
. (See expo-permissions
package).
import React from 'react';
import { Text, View, TouchableOpacity } from 'react-native';
import { Permissions } from 'expo-permissions';
import { Camera } from 'expo-camera';
export default class CameraExample extends React.Component {
state = {
hasCameraPermission: null,
type: Camera.Constants.Type.back,
};
async componentWillMount() {
const { status } = await Permissions.askAsync(Permissions.CAMERA);
this.setState({ hasCameraPermission: status === 'granted' });
}
render() {
const { hasCameraPermission } = this.state;
if (hasCameraPermission === null) {
return <View />;
} else if (hasCameraPermission === false) {
return <Text>No access to camera</Text>;
} else {
return (
<View style={{ flex: 1 }}>
<Camera style={{ flex: 1 }} type={this.state.type}>
<View
style={{
flex: 1,
backgroundColor: 'transparent',
flexDirection: 'row',
}}>
<TouchableOpacity
style={{
flex: 0.1,
alignSelf: 'flex-end',
alignItems: 'center',
}}
onPress={() => {
this.setState({
type:
this.state.type === Camera.Constants.Type.back
? Camera.Constants.Type.front
: Camera.Constants.Type.back,
});
}}>
<Text style={{ fontSize: 18, marginBottom: 10, color: 'white' }}> Flip </Text>
</TouchableOpacity>
</View>
</Camera>
</View>
);
}
}
}
Camera facing. Use one of Camera.Constants.Type
. When Type.front
, use the front-facing camera. When Type.back
, use the back-facing camera. Default: Type.back
.
Camera flash mode. Use one of Camera.Constants.FlashMode
. When on
, the flash on your device will turn on when taking a picture, when off
, it won't. Setting to auto
will fire flash if required, torch
turns on flash during the preview. Default: off
.
State of camera auto focus. Use one of Camera.Constants.AutoFocus
. When on
, auto focus will be enabled, when off
, it wont't and focus will lock as it was in the moment of change but it can be adjusted on some devices via focusDepth
prop.
A value between 0 and 1 being a percentage of device's max zoom. 0 - not zoomed, 1 - maximum zoom. Default: 0.
Camera white balance. Use one of Camera.Constants.WhiteBalance
: auto
, sunny
, cloudy
, shadow
, fluorescent
, incandescent
. If a device does not support any of these values previous one is used.
Distance to plane of sharpest focus. A value between 0 and 1: 0 - infinity focus, 1 - focus as close as possible. Default: 0. For Android this is available only for some devices and when useCamera2Api
is set to true.
Android only. A string representing aspect ratio of the preview, eg. 4:3
, 16:9
, 1:1
. To check if a ratio is supported by the device use getSupportedRatiosAsync
. Default: 4:3
.
Callback invoked when camera preview has been set.
Callback invoked with results of face detection on the preview. It will receive an object containing:
faceID
).{ x: number, y: number }
) -- position of the top left corner of a square containing the face in view coordinates,{ width: number, height: number }
) -- size of the square containing the face in view coordinates,{ x: number, y: number}
) -- position of the left ear in view coordinates,{ x: number, y: number}
) -- position of the right ear in view coordinates,{ x: number, y: number}
) -- position of the left eye in view coordinates,{ x: number, y: number}
) -- position of the right eye in view coordinates,{ x: number, y: number}
) -- position of the left cheek in view coordinates,{ x: number, y: number}
) -- position of the right cheek in view coordinates,{ x: number, y: number}
) -- position of the center of the mouth in view coordinates,{ x: number, y: number}
) -- position of the left edge of the mouth in view coordinates,{ x: number, y: number}
) -- position of the right edge of the mouth in view coordinates,{ x: number, y: number}
) -- position of the nose base in view coordinates.smilingProbability
, leftEyeOpenProbability
and rightEyeOpenProbability
are returned only if faceDetectionClassifications
property is set to .all
.
Positions of face landmarks are returned only if faceDetectionLandmarks
property is set to .all
.
See also FaceDetector
component.
Mode of the face detection. Use one of Camera.Constants.FaceDetection.Mode.{fast, accurate}
.
Whether to detect landmarks on the faces. Use one of Camera.Constants.FaceDetection.Landmarks.{all, none}
. See FaceDetector documentation for details.
Whether to run additional classifications on the faces. Use one of Camera.Constants.FaceDetection.Classifications.{all, none}
. See FaceDetector documentation for details.
Callback invoked when camera preview could not been started. It is provided with an error object that contains a message
.
Callback that is invoked when a bar code has been successfully read. The callback is provided with an Object of the shape { type: string, data: string }
, where the type refers to the bar code type that was scanned and the data is the information encoded in the bar code (in this case of QR codes, this is often a URL)
An array of bar code types. Usage: Camera.Constants.BarCodeType.<codeType>
where codeType
is one of the listed above. Default: all supported bar code types. For example: barCodeTypes={[Camera.Constants.BarCodeType.qr]}
Android only. Whether to use Android's Camera2 API. See Note
at the top of this page.
To use methods that Camera exposes one has to create a components ref
and invoke them using it.
// ...
<Camera
ref={ref => {
this.camera = ref;
}}
/>;
// ...
snap = async () => {
if (this.camera) {
let photo = await this.camera.takePictureAsync();
}
};
takePictureAsync
Takes a picture and saves it to app's cache directory. Photos are rotated to match device's orientation and scaled to match the preview. Anyway on Android it is essential to set ratio
prop to get a picture with correct dimensions.
options (object) --
A map of options:
Returns a Promise that resolves to an object: { uri, width, height, exif, base64 }
where uri
is a URI to the local image file (useable as the source for an Image
element) and width, height
specify the dimensions of the image. base64
is included if the base64
option was truthy, and is a string containing the JPEG data of the image in Base64--prepend that with 'data:image/jpg;base64,'
to get a data URI, which you can use as the source for an Image
element for example. exif
is included if the exif
option was truthy, and is an object containing EXIF data for the image--the names of its properties are EXIF tags and their values are the values for those tags.
The local image URI is temporary. Use Expo.FileSystem.copyAsync
to make a permanent copy of the image.
recordAsync
Starts recording a video that will be saved to cache directory. Videos are rotated to match device's orientation. Flipping camera during a recording results in stopping it.
options (object) --
A map of options:
Camera.Constants.VideoQuality['<value>']
, possible values: for 16:9 resolution 2160p
, 1080p
, 720p
, 480p
(Android only) and for 4:3 4:3
(the size is 640x480). If the chosen quality is not available for a device, the highest available is chosen.Returns a Promise that resolves to an object containing video file uri
property. The Promise is returned if stopRecording
was invoked, one of maxDuration
and maxFileSize
is reached or camera preview is stopped.
stopRecording
Stops recording if any is in progress.
getSupportedRatiosAsync
Android only. Get aspect ratios that are supported by the device and can be passed via ratio
prop.
Returns a Promise that resolves to an array of strings representing ratios, eg. ['4:3', '1:1']
.
Bar code format | iOS | Android |
---|---|---|
aztec | Yes | Yes |
codabar | No | Yes |
code39 | Yes | Yes |
code93 | Yes | Yes |
code128 | Yes | Yes |
code138 | Yes | No |
code39mod43 | Yes | No |
datamatrix | Yes | Yes |
ean13 | Yes | Yes |
ean8 | Yes | Yes |
interleaved2of5 | Yes | No |
itf14 | Yes* | Yes |
maxicode | No | Yes |
pdf417 | Yes | Yes |
rss14 | No | Yes |
rssexpanded | No | Yes |
upc_a | No | Yes |
upc_e | Yes | Yes |
upc_ean | No | Yes |
qr | Yes | Yes |
interleaved2of5
.FAQs
A React component that renders a preview for the device's either front or back camera. Camera's parameters like zoom, auto focus, white balance and flash mode are adjustable. With expo-camera, one can also take photos and record videos that are saved to t
The npm package expo-camera receives a total of 132,590 weekly downloads. As such, expo-camera popularity was classified as popular.
We found that expo-camera demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.