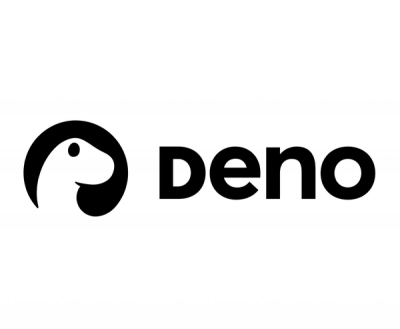
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
expo-splash-screen
Advanced tools
Provides a module to allow keeping the native Splash Screen visible until you choose to hide it.
The expo-splash-screen package is used to control and customize the splash screen for Expo and React Native apps. It allows developers to show a splash screen while the app is loading, hide it once the app is ready, and customize its appearance.
Preventing Auto-Hide
This feature allows you to prevent the splash screen from auto-hiding. This is useful if you need to perform some asynchronous operations before the splash screen is hidden.
import * as SplashScreen from 'expo-splash-screen';
SplashScreen.preventAutoHideAsync();
Hiding the Splash Screen
This feature allows you to manually hide the splash screen once your app is ready. This is typically done after your app has finished loading all necessary resources.
import * as SplashScreen from 'expo-splash-screen';
async function hideSplashScreen() {
await SplashScreen.hideAsync();
}
Showing the Splash Screen
This feature allows you to manually show the splash screen. This can be useful if you need to display the splash screen again after it has been hidden.
import * as SplashScreen from 'expo-splash-screen';
async function showSplashScreen() {
await SplashScreen.showAsync();
}
The react-native-splash-screen package provides similar functionality to expo-splash-screen, allowing you to control the splash screen in a React Native app. It offers methods to show and hide the splash screen, but it requires more manual setup compared to expo-splash-screen.
The react-native-bootsplash package is another alternative for managing splash screens in React Native apps. It provides a more customizable and flexible approach compared to expo-splash-screen, with support for different splash screen images for different devices and orientations.
expo-splash-screen
allows you to customize your app's splash screen, which is the initial screen users see when the app is launched, before it has loaded. Splash screens (sometimes called launch screens) provide a user's first experience with your application.
expo-splash-screen
contains a built-in feature for taking care of properly displaying your splash screen image. You can use the following resize modes to obtain behavior as if you were using the React Native <Image>
component's resizeMode
style.
CONTAIN
resize modeScale the image uniformly (maintaining the image's aspect ratio) so that both dimensions the width and height of the image will be equal to or less than the corresponding dimension of the device's screen.
Android | iOS |
---|---|
![]() | ![]() |
COVER
resize modeScale the image uniformly (maintaining the image's aspect ratio) so that both the width and height of the image will be equal to or larger than the corresponding dimension of the device's screen.
Android | iOS |
---|---|
![]() | ![]() |
NATIVE
resize modeAndroid only.
By using this resize mode your app will will leverage Android's ability to present a static bitmap while the application is starting up. Android (unlike iOS) does not support stretching of the provided image during launch, so the application will present the given image centered on the screen at its original dimensions.
Android |
---|
![]() |
Animation above presents one of our known issues
Selecting this resize mode requires some more work to be done in native configuration.
Please take a look at the res/drawable/splashscreen.xml
and res/drawable/splashscreen_background.png
sections.
import * as SplashScreen from 'expo-splash-screen';
The native splash screen that is controlled via this module autohides once the ReactNative-controlled view hierarchy is mounted. This means that when your app first render
s view component, the native splash screen will hide. This default behavior can be prevented by calling SplashScreen.preventAutoHideAsync()
and later on SplashScreen.hideAsync()
.
SplashScreen.preventAutoHideAsync()
This method makes the native splash screen stay visible until SplashScreen.hideAsync()
is called. This must be called before any ReactNative-controlled view hierarchy is rendered (either in the global scope of your main component, or when the component renders null
at the beginning - see Examples section).
Preventing default autohiding might come in handy if your application needs to prepare/download some resources and/or make some API calls before first rendering some actual view hierarchy.
A Promise
that resolves to true
when preventing autohiding succeeded and to false
if the native splash screen is already prevented from autohiding (for instance, if you've already called this method).
Promise
rejection most likely means that native splash screen cannot be prevented from autohiding (it's already hidden when this method was executed).
SplashScreen.hideAsync()
Hides the native splash screen. Only works if the native splash screen has been previously prevented from autohiding by calling SplashScreen.preventAutoHideAsync()
method.
A Promise
that resolves to true
once the splash screen becomes hidden and to false
if the splash screen is already hidden.
SplashScreen.preventAutoHideAsync()
in global scopeApp.tsx
import React from 'react';
import * as SplashScreen from 'expo-splash-screen';
// Prevent native splash screen from autohiding before App component declaration
SplashScreen.preventAutoHideAsync()
.then(result => console.log(`SplashScreen.preventAutoHideAsync() succeeded: ${result}`))
.catch(console.warn); // it's good to explicitly catch and inspect any error
export default class App extends React.Component {
componentDidMount() {
// Hides native splash screen after 2s
setTimeout(async () => {
await SplashScreen.hideAsync();
}, 2000);
}
render() {
return (
<View style={styles.container}>
<Text style={styles.text}>SplashScreen Demo! 👋</Text>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: '#aabbcc',
},
text: {
color: 'white',
fontWeight: 'bold',
},
};
SplashScreen.preventAutoHideAsync()
in component that initially renders null
App.tsx
import React from 'react';
import * as SplashScreen from 'expo-splash-screen';
export default class App extends React.Component {
state = {
appIsReady: false,
};
async componentDidMount() {
// Prevent native splash screen from autohiding
try {
await SplashScreen.preventAutoHideAsync();
} catch (e) {
console.warn(e);
}
this.prepareResources();
}
/**
* Method that serves to load resources and make API calls
*/
prepareResources = async () => {
await performAPICalls(...);
await downloadAssets(...);
this.setState({ appIsReady: true }, async () => {
await SplashScreen.hideAsync();
});
}
render() {
if (!this.state.appIsReady) {
return null;
}
return (
<View style={styles.container}>
<Text style={styles.text}>SplashScreen Demo! 👋</Text>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: '#aabbcc',
},
text: {
color: 'white',
fontWeight: 'bold',
},
};
Managed Expo projects are not supported by this unimodule at this moment. Instead, you should use the Expo.SplashScreen
module that is within the expo
package.
For bare React Native projects, you must ensure that you have installed and configured the react-native-unimodules
package before continuing.
expo install expo-splash-screen
Run pod install
in the ios
directory after installing the package.
To achieve native splash screen (in iOS ecosystem it's called LaunchScreen
) behavior, you have to provide either a SplashScreen.storyboard
file or a SplashScreen.xib
file, and configure your Xcode project accordingly.
The guide below shows how to configure your Xcode project to use single image file as a splash screen using a .storyboard
file (configuration for .xib
filetype is analogous).
Images.xcassets
First you need to add the image file that would serve as a splash screen to your native project's resources.
.xcassets
(often named Images.xcassets
or Assets.xcassets
) file.New image set
and name it SplashScreen
.SplashScreen.storyboard
This is the actual splash screen definition and will be used by the system to render your splash screen.
SplashScreen.storyboard
file.View Controller
to the newly created .storyboard
file:
Library
(+
button on the top-right),View Controller
element,.storyboard
file.Image View
to the View Controller
:
View
element from View Controller
,Library
(+
button on the top-right),Image View
element,View Controller
child in view hierarchy inspector.Storyboard ID
to SplashScreenViewController
:
View Controller
in view hierarchy inspector,Identity Inspector
in the right panel,Storyboard ID
to SplashScreenViewController
.Image View
source:
Image View
in view hierarchy inspector,Attributes Inspector
in the right panel,SplashScreen
in Image
parameter).Background
of the Image View
:
Image View
in view hierarchy inspector,Attributes Inspector
in the right panel,Background
parameter:
#RRGGBB
value you need to select Custom
option and in the Colors Popup
that appeared you need to navigate to the second tab and choose RGB Sliders
from dropdown select.ImageView
's ContentMode
This is how your image will be displayed on the screen.
SplashScreen.storyboard
and select Image View
from View Controller
.Attributes Inspector
in the right panel and locate Content Mode
.Aspect Fit
to obtain CONTAIN resize mode,Aspect Fill
to obtain COVER resize mode.The newly created SplashScreen.storyboard
needs to be marked as the Launch Screen File
in your Xcode project in order to be presented from the very beginning of your application launch.
Project Navigator
TARGETS
panel and navigate to General
tab.App Icons and Launch Images
section and Launch Screen File
option.SplashScreen
as the value for located option.To achieve fully-native splash screen behavior, expo-splash-screen
needs to be hooked into the native view hierarchy and consume some resources that have to be placed under /android/app/src/res
directory.
SplashScreen.show(Activity activity, SplashScreenImageResizeMode mode, Class rootViewClass)
This native method is used to hook SplashScreen
into the native view hierarchy that is attached to the provided activity.
You can use this method to customize how the splash screen view will be presented. Pass one of SplashScreenImageResizeMode.{CONTAIN, COVER, NATIVE}
as second argument to do so.
MainActivity.{java,kt}
Modify MainActivity.{java,kt}
or any other activity that is marked in the application main AndroidManifest.xml
as a main activity of your application (main activity is marked with the android.intent.action.MAIN
intent filter. You can take a look at this example from official Android docs).
Ensure SplashScreen.show(...)
method is called after super.onCreate(...)
(if onCreate
method is not overwritten yet, override it including SplashScreen.show(...)
).
+ import expo.modules.splashscreen.SplashScreen;
+ import expo.modules.splashscreen.SplashScreenImageResizeMode;
public class MainActivity extends ReactActivity {
// other methods
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
+ // SplashScreen.show(...) has to be called after super.onCreate(...)
+ SplashScreen.show(this, SplashScreenImageResizeMode.CONTAIN, ReactRootView.class);
...
}
// other methods
}
res/drawable/splashscreen_background.png
You have to provide your splash screen image and place it under the res/drawable
directory.
This image will be loaded as soon as Android mounts your application's native view hierarchy.
NATIVE
mode adjustmentsIf you've selected SplashScreenImageResizeMode.NATIVE
mode in SplashScreen.show
, you need to do a few additional steps.
In your application's res
directory you might want to have a number of drawable-X
sub-directories (where X
is the different DPI for different devices). They store different versions of images that are picked based on the device's DPI (for more information please see this official Android docs).
To achieve proper scaling of your splash screen image on every device you should have following directories:
res/drawable-mdpi
- scale 1x - resources for medium-density (mdpi) screens (~160dpi). (This is the baseline density.)res/drawable-hdpi
- scale 1.5x - resources for high-density (hdpi) screens (~240dpi).res/drawable-xhdpi
- scale 2x - resources for extra-high-density (xhdpi) screens (~320dpi).res/drawable-xxhdpi
- scale 3x - resources for extra-extra-high-density (xxhdpi) screens (~480dpi).res/drawable-xxxhdpi
- scale 4x - resources for extra-extra-extra-high-density (xxxhdpi) uses (~640dpi).Each of directories mentioned above should contain the same splashscreen_image.png
file, but with a different resolution (pay attention to scale factors).
res/values/colors.xml
This file contains colors that are reused across your application at the native level. Update the file with the following content or create one if missing:
<resources>
+ <color name="splashscreen_background">#AABBCC</color> <!-- #AARRGGBB or #RRGGBB format -->
<!-- Other colors defined for your application -->
</resources>
res/drawable/splashscreen.xml
This file contains the description of how the splash screen view should be drawn by the Android system. Create the file with the following content:
+ <layer-list xmlns:android="http://schemas.android.com/apk/res/android">
+ <item android:drawable="@color/splashscreen_background"/>
+ </layer-list>
NATIVE
mode adjustmentsIf you've selected SplashScreenImageResizeMode.NATIVE
mode in SplashScreen.show
, you should add:
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@color/splashscreen_background"/>
+ <item>
+ <bitmap android:gravity="center" android:src="@drawable/splashscreen_image"/>
+ </item>
</layer-list>
res/values/styles.xml
Locate your main activity theme in /android/app/src/res/values/styles.xml
or create one if missing.
<!-- Main activity theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar">
+ <item name="android:windowBackground">@drawable/splashscreen</item> <!-- this line instructs the system to use 'splashscreen.xml' as a background of the whole application -->
<!-- Other style properties -->
</style>
AndroidManifest.xml
Adjust your application's main AndroidManifest.xml
to contain an android:theme
property pointing to the style that contains your splash screen configuration:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapp">
...
<application ...>
+ <!-- Ensure that 'android:theme' property is pointing to the style containing native splash screen reference - see 'styles.xml' -->
<activity
android:name=".MainActivity"
+ android:theme="@style/AppTheme"
...
>
...
</activity>
</application>
</manifest>
Contributions are very welcome! Please refer to guidelines described in the contributing guide.
Splash Screens on iOS apps can sometimes encounter a caching issue where the previous image will flash before showing the new, intended image. When this occurs, we recommend you try power cycling your device and uninstalling and re-installing the application. However, the caching sometimes can persist for a day or two so be patient if the aforementioned steps were unable to resolve the issue.
NATIVE
mode pushes splash image up a little bitSee NATIVE
mode preview above.
We are aware of this issue and unfortunately haven't been able to provide a solution as of yet. This is on our immediate roadmap...
This module is based on a solid work from (many thanks for that 👏):
FAQs
Provides a module to allow keeping the native Splash Screen visible until you choose to hide it.
The npm package expo-splash-screen receives a total of 429,571 weekly downloads. As such, expo-splash-screen popularity was classified as popular.
We found that expo-splash-screen demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 28 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.