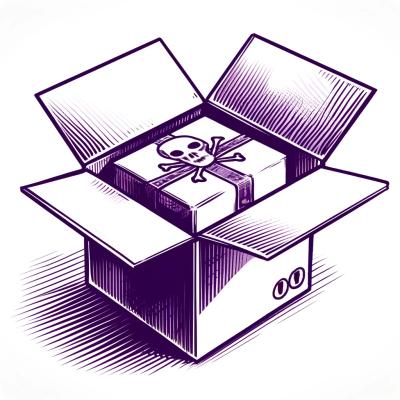
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
express-urlrewrite
Advanced tools
The express-urlrewrite package is a middleware for Express.js that allows you to rewrite URLs based on specified patterns. This can be useful for redirecting traffic, simplifying URLs, or handling legacy URL structures.
Simple URL Rewriting
This feature allows you to rewrite a simple URL from '/old-path' to '/new-path'. When a user visits '/old-path', they will be served the content from '/new-path'.
const express = require('express');
const rewrite = require('express-urlrewrite');
const app = express();
app.use(rewrite('/old-path', '/new-path'));
app.get('/new-path', (req, res) => {
res.send('This is the new path');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Pattern-based URL Rewriting
This feature allows you to rewrite URLs based on patterns. In this example, any URL matching '/user/:id' will be rewritten to '/profile/:id', where ':id' is a dynamic parameter.
const express = require('express');
const rewrite = require('express-urlrewrite');
const app = express();
app.use(rewrite('/user/:id', '/profile/:id'));
app.get('/profile/:id', (req, res) => {
res.send(`Profile ID: ${req.params.id}`);
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Regular Expression URL Rewriting
This feature allows you to use regular expressions for URL rewriting. In this example, any URL matching the pattern '/old-<number>' will be rewritten to '/new/<number>'.
const express = require('express');
const rewrite = require('express-urlrewrite');
const app = express();
app.use(rewrite(/^\/old-(\d+)$/, '/new/$1'));
app.get('/new/:id', (req, res) => {
res.send(`New ID: ${req.params.id}`);
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
The connect-modrewrite package provides similar functionality to express-urlrewrite, allowing you to rewrite URLs using mod_rewrite syntax. It is more flexible in terms of complex rewrite rules but may have a steeper learning curve.
The express-rewrite package is another alternative for URL rewriting in Express.js. It offers similar features but with a different API. It is less popular than express-urlrewrite but can be a good alternative for specific use cases.
The express-redirect package focuses on URL redirection rather than rewriting. It allows you to easily set up HTTP redirects in your Express.js application. While it doesn't offer the same level of URL manipulation as express-urlrewrite, it is useful for simple redirection needs.
URL rewrite middleware for express.
Rewrite using a regular expression, rewriting /i123
to /items/123
.
app.use(rewrite(/^\/i(\w+)/, '/items/$1'));
Rewrite using route parameters, references may be named
or numeric. For example rewrite /foo..bar
to /commits/foo/to/bar
:
app.use(rewrite('/:src..:dst', '/commits/$1/to/$2'));
app.use(rewrite('/:src..:dst', '/commits/:src/to/:dst'));
You may also use the wildcard *
to soak up several segments,
for example /js/vendor/jquery.js
would become
/public/assets/js/vendor/jquery.js
:
app.use(rewrite('/js/*', '/public/assets/js/$1'));
In the above examples, the original query string (if any) is left untouched. The regular expression is applied to the full url, so the query string can be modified as well:
app.use(rewrite('/file\\?param=:param', '/file/:param'))
The query string delimiter (?) must be escaped for the regular expression to work.
app.use(rewrite('/path', '/anotherpath?param=some'))
now updates req.query, so req.query.param == 'some'
.
rewrite can be used as a route middleware as in
app.get('/route/:var', rewrite('/rewritten/:var'));
app.get('/rewritten/:var', someMw);
Instead of passing control to next middleware, it passes control to next route.
Set environment variable DEBUG=express-urlrewrite
FAQs
URL rewrite middleware for express
The npm package express-urlrewrite receives a total of 147,526 weekly downloads. As such, express-urlrewrite popularity was classified as popular.
We found that express-urlrewrite demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.