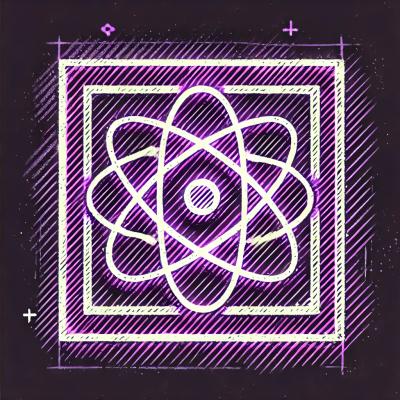
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
express-zod-api
Advanced tools
Start your API server with I/O schema validation and custom middlewares in minutes.
yarn install express-zod-api
Add the following options to your tsconfig.json
file in order to make it work as expected:
{
"compilerOptions": {
"noImplicitAny": true,
"strictNullChecks": true
}
}
Full example in ./example
. You can clone the repo and run yarn start
to check it out in action.
const config: ConfigType = {
server: {
listen: 8090,
cors: true
},
logger: {
level: 'debug',
color: true
}
};
See ./src/config-type.ts
for all available options.
const endpointsFactory = new EndpointsFactory();
You can also instantly add middlewares to it using .addMiddleware()
method.
const getUserEndpoint = endpointsFactory
.build({
methods: ['get'],
input: z.object({
id: z.string().transform((id) => parseInt(id, 10))
}),
output: z.object({
name: z.string(),
}),
handler: async ({input: {id}, options, logger}) => {
logger.debug(`Requested id: ${id}`);
logger.debug('Options:', options);
const name = 'John Doe';
return { name: 'John Doe' };
}
});
Note: options
come from the output of middlewares.
You can add middlewares by using .addMiddleware()
method before .build()
.
All inputs and outputs are validated.
const routing: Routing = {
v1: {
getUser: getUserEndpoint
}
};
This sets up getUserEndpoint to handle requests to the /v1/getUser path.
createServer(config, routing);
You can create middlewares separately using createMiddleware()
function and connect them later.
All outputs of connected middlewares are put in options
argument of the endpoint handler.
All middleware inputs are also available as the endpoint inputs.
// This one provides the method of the request
const methodProviderMiddleware = createMiddleware({
input: z.object({}).nonstrict(),
middleware: async ({request}) => ({
method: request.method.toLowerCase() as Method,
})
});
// This one performs the authentication
// using key from the input and token from headers
const authMiddleware = createMiddleware({
input: z.object({
key: z.string().nonempty()
}),
middleware: async ({input: {key}, request, logger}) => {
logger.debug('Checking the key and token...');
if (key !== '123') {
throw createHttpError(401, 'Invalid key');
}
if (request.headers['token'] !== '456') {
throw createHttpError(401, 'Invalid token');
}
return {token: request.headers['token']};
}
});
You can also implement the validation inside the input schema:
const authMiddleware = createMiddleware({
input: z.object({
key: z.string().nonempty()
.refine((key) => key === '123', 'Invalid key')
}),
...
})
You can instantiate your own express app and connect your endpoints the following way:
const config: ConfigType = {...};
const logger = createLogger(config);
const routing = {...};
initRouting({app, logger, config, routing});
You can export only the types of your endpoints for your front-end:
export type GetUserEndpoint = typeof getUserEndpoint;
Then use provided helpers to obtain their input and output types:
type GetUserEndpointInput = EndpointInput<GetUserEndpoint>;
type GetUserEndpointOutput = EndpointOutput<GetUserEndpoint>;
You can generate the specification of your API the following way and write it to a .yaml
file:
const yamlString = generateOpenApi({
routing,
version: '1.2.3',
title: 'Example API',
serverUrl: 'http://example.com'
}).getSpecAsYaml();
Unfortunately Typescript does not perform excess proprety check for objects resolved in Promise
, so there is no error during development of endpoint's output.
endpointsFactory.build({
methods, input,
output: z.object({
anything: z.number()
}),
handler: async () => ({
anything: 123,
excessive: 'something' // no type error
})
});
You can achieve this check by assigning the output schema to a constant and reusing it in additional definition of handler's return type:
const output = z.object({
anything: z.number()
});
endpointsFactory.build({
methods, input, output,
handler: async (): Promise<z.infer<typeof output>> => ({
anything: 123,
excessive: 'something' // error TS2322, ok!
})
});
FAQs
A Typescript framework to help you get an API server up and running with I/O schema validation and custom middlewares in minutes.
We found that express-zod-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.