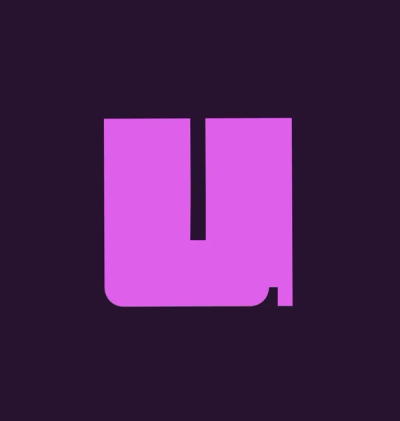
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
extended-spine
Advanced tools
Extended spine framework. It gives you ability to work with controllers almost like in Angular.
You can use it for example with SimQ.
$ npm install extended-spine
Before everything, you have to add this one line of code into your javascript.
Controller = require 'extended-spine'
Controller.init($)
Now only spine controller is extended.
Controller = require 'extended-spine/Controller'
class MyController extends Controller
constructor: (@el) ->
super
module.exports = MyController
As you can see, everything is same like in classic spine, only extended class is different.
This module will automatically look for elements in your page with data-application
attribute. If it will not find it, whole html page will
be used. Every element inside this data-application
element with data-controller
element will be used for controller.
Example is much better for explain.
...
<div data-application>
...
<div data-controller="/path/to/controller/for/this/element">...</div>
...
</div>
...
Text in data-controller
attribute is path used in require method.
This means that now you don't have to instantiate controllers on your own. :-)
If you like dependency injection pattern with autowired dependencies (again for example like in angular), you can use this feature also with this module.
Uses dependency-injection module.
DI = require 'dependency-injection'
di = new DI
Controller.init($, di)
Some controller:
Controller = require 'extended-spine/Controller'
class Chat extends Controller
http: null
jquery: null
model: null
constructor: (@el, @http, @jquery, @model) ->
module.exports = Chat
Chat module is dependent on three services. First argument will always be the container element and others will be services from DI container.
Check documentation of dependency-injection module to see how to add services into your DI container.
This works only with controllers which were created with data-controller attribute.
If your application uses for example ajax for repainting elements, you can use two methods, showed below for refreshing your controllers.
Controller = require 'extended-spine/Controller'
Controller.init($)
el = $('#element-which-will-be-repainted')
$.get(url, (data) ->
Controller.unbind(el)
el.html(data)
Controller.refresh(el)
)
Methods unbind
and refresh
manipulates also with element on which it was called. You can of course disable that.
Controller.unbind(el, false)
Controller.refresh(el, false)
From jQuery element:
menu = $('#menu').getController()
// or
menu = $('[data-controller="/app/controller/Menu"]').getController()
Otherwise:
menu = Controller.find('/app/controller/Menu')
If you don't want to instantiate some controller immediately, you can add html attribute data-lazy
to.
<div data-controller="/path/to/my/controller" data-lazy></div>
Now when you want to create instance of this controller, you have to get it's controller factory and use it.
factory = Controller.find('/path/to/my/controller') // for lazy controllers, factory function is returned
controller = factory() // just call it and it will return created controller
You can also set if some of your controllers is only for mobile or only for computers.
<div data-controller="/app/controllers/just/for/mobile" data-mobile>
<div data-controller="/app/controllers/just/for/computer" data-computer>
$ npm test
1.3.0
1.2.0
1.1.0
1.0.4
1.0.2 - 1.0.3
1.0.1
1.0.0
FAQs
[ABANDONED] Some extensions for spine framework
The npm package extended-spine receives a total of 1 weekly downloads. As such, extended-spine popularity was classified as not popular.
We found that extended-spine demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.