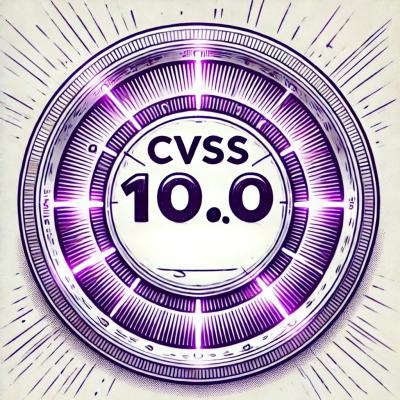
Security News
cURL Project and Go Security Teams Reject CVSS as Broken
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Easy and light weight interface wrapping the native node.js MongoDB driver, the mongodb module.
Simple wrapper to use basic insert/find/modify/delete functions. Ability to access native collection and database objects for more advanced operations.
By default uses shortId so that new objects have short strings as _ids instead of ObjectIDs. Also by default has protections in place to prevent accidentally clobbering an object on update. Optional ability to require the fields to retrieve for find() operations to be specified (good for performance!) and many other features as well!
Provides the following functions:
As well as access to the native collection and database objects:
Ability to enable/disable (good for maintenance mode)
npm install ezmongo
Constructs an EzMongo instance. The only required option is database, the rest have defaults.
EzMongo(options)
Connection options:
Feature options:
Logging options:
var ezMongo = new EzMongo({database: 'ezMongoTestDb'});
// below will query as soon as DB is connected
ezMongo.findOne('myCollection', function(err, doc) {
console.log('look what I found!',doc);
});
Looks up a single document from a collection. Callback with the document if found, or null if not. If multiple documents match the search and no sort is provided, the document returned is non-deterministic and up to the database.
findOne(collectionName, _idOrSearch, fields, sort, callback)
ezMongo.findOne('myCollection', {num: {$gte: 2}}, ['field1','field2.subfield'], [['rank','asc']], function(err, doc) {
console.log('Found document with _id ', doc._id, 'field1', doc.field1, 'and subfield', doc.field2.subfield);
});
Looks up multiple documents from a collection. Callback with the array of found documents. If no documents are found the array will be empty.
findMultiple(collectionName, _idsOrSearch, fields, sort, limit, skip, callback)
ezMongo.findMultiple('myCollection', {num: {$gte: 2}}, ['field1'], [['rank','asc']], 10, 20, function(err, docs) {
if (docs.length) {
console.log('Found documents ranked 11 through', 10+docs.length); //most will be 11 through 30
docs.forEach(function(doc) {
console.log('Found doc',doc);
});
} else {
console.log('no more documents found');
}
});
Updates a single document. Callback with number of documents modified: 1 if a document modified, 0 if not. If multiple documents match the search, the one that will be modified is non-deterministic and up to the database.
updateOne(collectionName, _idOrSearch, changes, callback)
ezMongo.updateOne('myCollection','_id1', {$set: {lastModified: new Date()}, $inc: {views: 1}}, function(err, success) {
if (success) {
console.log('document modified');
} else {
console.log('failed to find document with _id of _id1 to modify');
}
});
Updates multiple documents. Callback with number of documents modified.
updateMultiple(collectionName, _idsOrSearch, changes, callback)
ezMongo.updateMultiple('myCollection','{powerLevel: {$gt: 9000}}', {$set: {state: 'awesome'}}, function(err, numModified) {
console.log('Marked',numModified,'documents as awesome');
});
Upserts a single document. Updates it if found, otherwise inserts it. Callback with number of documents modified: 1 if a document inserted or updated, 0 if not. If multiple documents match the search, the one that will be modified is non-deterministic and up to the database.
updateOne(collectionName, _idOrSearch, changes, callback)
ezMongo.upsertOne('myCollection', {$num: 1}, {$set: {char: 'A'}}, function(err, numModified) {
if (numModified) {
console.log('document modified or inserted');
} else {
console.log('no modification needed');
}
});
Upserts multiple documents. Updates if any are found, otherwise inserts it. Callback with number of documents modified or 1 if a new document was inserted. If multiple documents match the search, all will be updated.
upsertMultiple(collectionName, _idsOrSearch, changes, callback)
ezMongo.upsertMultiple('myCollection',{type: 'dog'}, {$set: {name: 'Sophie'}}, function(err, numModified) {
console.log('We now have',numModified,'dogs named Sophie');
});
Removes a single document. Callback with number of documents removed: 1 if removed, 0 if not. If multiple documents match the search, the one that will be removed is non-deterministic and up to the database.
removeOne(collectionName, _idOrSearch, callback)
ezMongo.removeOne('myCollection', {powerLevel: {$lte: 9000}}, function(err, success) {
if (success) {
console.log('a document has been removed');
} else {
console.log('no documents with power levels 9000 or less to remove');
}
});
Removes multiple documents. Callback with number of documents removed.
removeMultiple(collectionName, _idsOrSearch, callback)
ezMongo.removeMultiple('myCollection', ['_id1','_id2','_id3'], function(err, numRemoved) {
if (numRemoved === 3) {
console.log('target documents removed');
} else {
console.log('Removed',numRemoved, 'documents;', 3 - numRemoved, 'documents not in collection');
}
});
Insert documents into the database. If documents don't have an _id, one will be automatically generated either by using shortId, or if useShortId constructor option was false by the database. Callback with the _id, or array of _ids of the newly inserted documents.
insert(collectionName, docs, callback)
ezMongo.insert('myCollection', {num: 3, powerLevel: 9001}, function(err, _id) {
console.log('Inserted new document has _id', _id);
});
Counts the number of documents in a collection that match the optionally provided search.
count('myCollection', {age: {'$gte': 18}}, callback)
Provides access to the native collection object for a collection. Callback with the native collection.
collection(collectionName, callback)
ezMongo.collection('myCollection', function(err, collection) {
collection.count(function(err, count) {
console.log(count,'documents in myCollection');
}):
});
Provides access to the native database object. Will attempt to connect. Callback with the native database.
db(callback)
ezMongo.db(function(err, db) {
db.collectionNames(function(err, names)) {
console.log('Collections on this database: ');
names.forEach(function(name) {
console.log(name);
});
};
});
Effectively disables the database as any subsequent EzMongo commands (other than enable/disable) will result in an error. Callback with whether or not the database was already disabled.
disable(callback)
ezMongo.disable();
ezMongo.findOne('myCollection','_id1', function(err, doc) {
// err will be non-null; doc will be null
if (err) {
console.log('Error doing find');
} else {
// won't get here
}
}):
Effectively enables the database, with EzMongo commands executing as intended. Callback with whether or not the database was already enabled.
enable(callback)
ezMongo.enable(function(err, alreadyEnabled) {
if (!allreadyEnabled) {
console.log('Database commands will now work again');
} else {
console.log('Database was already enabled');
}
});
The features are not yet inclusive. The collection and db commands allow getting around this, however going forward additional features (e.g. count()) may be added as the needs arise.
Testing uses nodeunit, which you can install globally
npm install -g nodeunit
To run the tests, execute:
nodeunit test/
This module is not yet fully covered by tests. Particularly the various constructor options. While uses in production also help tease out errors, expanded test coverage is a goal.
Version 0.0.6 has been tested and works with node version 0.12.4 and MongoDB 3.0.3. If you use older versions you may need an older version of ezmongo.
EzMongo was originally created for Node Knockout 2011 winner for Most Fun, Doodle Or Die and is still used today. Millions of hilarious doodles have been saved and accessed using EzMongo. EzMongo is also used for projects by the Video Blocks team. Unlimited downloads stock video, after effects templates, and more for one flat price.
FAQs
Easy and light weight interface wrapping the native node.js mongo driver
We found that ezmongo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.