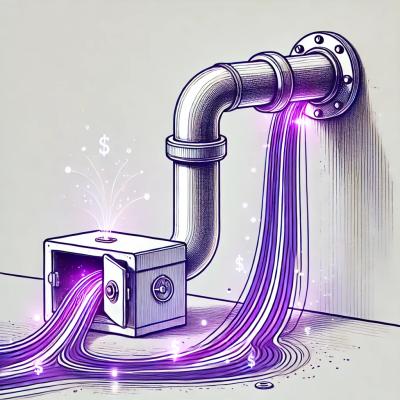
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
ezobjects
Advanced tools
EZ Objects is a Node.js module (that can also be usefully browserify'd) that aims to save you lots of time writing the initializers, property getters, and property setters for your data model objects. The library takes the form of a single function that can be passed a generic object containing a few configuration keys, defined below, that will allow it to automatically generate a new ES6 class object with the following features:
Auto-initializes all properties (including parent object properties, if extended)
_
, fortunately the module will strip the _
from the object if you wish to initialize
a new object using a JSON encoded/parsed version of the same objectAutomatically creates methods that perform getter/setter functionality with strict data typing
See the examples below to witness them used in a variety of situations.
Fully operational! Please open an issue for any bug reports or feature requests.
This module, when required, is a function that takes a single object argument. At present, that object can have the following keys:
Each property in the properties array is an object that can have the following keys:
Default defaults are:
Note that the created objects are added to the global space, being global
(node) or window
(browser), though you'll
have to browserify or equivalent to use in browser. Like normal classes, they can have other properties/methods added
externally using the prototype, though note that if you want external prototype-added properties to be initialized, you'll
have to rewrite the init() function manually. Alternatively, you can just extend the class and init the parent with
super
, see examples below.
const ezobjects = require('ezobjects');
/** Create a customized object on the global (node) or window (browser) namespace */
ezobjects({
name: 'DatabaseRecord',
properties: [
{ name: 'id', type: 'int' }
]
});
/** Example of the object newly instansiated */
const a = new DatabaseRecord();
console.log(a);
DatabaseRecord { _id: 0 }
/** Create another customized object that extends the first one */
ezobjects({
name: 'Person',
extends: DatabaseRecord,
properties: [
{ name: 'firstName', type: 'string' },
{ name: 'lastName', type: 'string' },
{ name: 'checkingBalance', type: 'float' },
{ name: 'permissions', type: 'Array' },
{ name: 'favoriteDay', type: 'Date' }
]
});
/** Example of the extended object newly instansiated */
const b = new Person();
console.log(b);
Person {
_id: 0,
_firstName: '',
_lastName: '',
_checkingBalance: 0,
_permissions: [],
_favoriteDay: null }
/** Example of the extended object instansiated and initialized using object passed to constructor */
const c = new Person({
id: 1,
firstName: 'Rich',
lastName: 'Lowe',
checkingBalance: 4.87,
permissions: [1, 2, 3],
favoriteDay: new Date('01-01-2018')
});
console.log(c);
Person {
_id: 1,
_firstName: 'Rich',
_lastName: 'Lowe',
_checkingBalance: 4.87,
_permissions: [ 1, 2, 3 ],
_favoriteDay: 2018-01-01T06:00:00.000Z }
/** Example of the extended object instansiated, then loaded with data using setter methods */
const d = new Person();
d.id(2);
d.firstName('Bert');
d.lastName('Reynolds');
d.checkingBalance(91425518.32);
d.permissions([1, 4]);
d.favoriteDay(new Date('06-01-2017'));
console.log(d);
Person {
_id: 2,
_firstName: 'Bert',
_lastName: 'Reynolds',
_checkingBalance: 91425518.32,
_permissions: [ 1, 4 ],
_favoriteDay: 2017-06-01T05:00:00.000Z }
/** Example of the extended object's properties being accessed using getter methods */
console.log(`ID: ${d.id()}`);
console.log(`First Name: ${d.firstName()}`);
console.log(`Last Name: ${d.lastName()}`);
console.log(`Checking Balance: $${d.checkingBalance()}`);
console.log(`Permissions: ${d.permissions().join(`, `)}`);
console.log(`Favorite Day: ${d.favoriteDay().toString()}`);
ID: 2
First Name: Bert
Last Name: Reynolds
Checking Balance: $91425518.32
Permissions: 1, 4
Favorite Day: Thu Jun 01 2017 00:00:00 GMT-0500 (CDT)
/** Adding property to the generated object's prototype */
DatabaseRecord.prototype.table = function (arg) {
/** Getter */
if ( arg === undefined )
return this._table;
/** Setter */
else if ( typeof arg == 'string' )
this._table = arg;
/** Handle type errors */
else
throw new TypeError(`${this.constructor.name}.table(${typeof arg}): Invalid signature.`);
/** Return this object for set call chaining */
return this;
};
/** Yuck, now I have to manually override the init() call if I want it initialized */
DatabaseRecord.prototype.init = function (data = {}) {
this.id(data.id || 0);
this.table(data.table || '');
};
const e = new DatabaseRecord();
console.log(e);
DatabaseRecord { _id: 0, _table: '' }
/** Adding arbitrary capability other than property to the generated object's prototype */
DatabaseRecord.prototype.hello = function () {
return "Hello, World!";
};
const f = new DatabaseRecord();
console.log(f.hello());
Hello, World!
/** These objects can be extended instead to accomplish the same things if preferred */
class DatabaseRecord2 extends DatabaseRecord {
constructor(data = {}) {
super(data);
}
init(data = {}) {
super.init(data);
this.test('Test');
}
test(arg) {
/** Getter */
if ( arg === undefined )
return this._test;
/** Setter */
else if ( typeof arg == 'string' )
this._test = arg.toString();
/** Handle type errors */
else
throw new TypeError(`${this.constructor.name}.test(${typeof arg}): Invalid signature.`);
/** Return this object for set call chaining */
return this;
}
}
const g = new DatabaseRecord2();
console.log(g);
console.log(g.hello());
DatabaseRecord2 { _id: 0, _table: '', _test: 'Test' }
Hello, World!
FAQs
Easy Auto-Generated Strictly-Typed JavaScript Class Objects
The npm package ezobjects receives a total of 147 weekly downloads. As such, ezobjects popularity was classified as not popular.
We found that ezobjects demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.