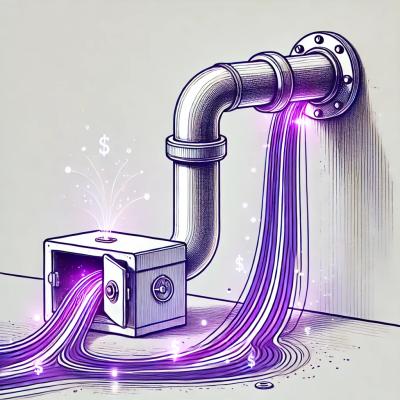
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
ezobjects
Advanced tools
EZ Objects is a Node.js module (that can also be usefully browserify'd) that aims to save you lots of time writing class objects. All you have to do is create simple configurations for each of your objects and then call the library function(s). Let's start by showing a basic example:
const ezobjects = require('ezobjects');
/**
* Create a customized object on the global (node) or window (browser)
* namespace.
*/
ezobjects.createObject({
className: 'DatabaseRecord',
properties: [
{ name: 'id', type: 'number', setTransform: x => parseInt(x) }
]
});
const record = new DatabaseRecord();
In this short snippet, we have effectively created a new class named DatabaseRecord
. The class
has a constructor, an initializer, and a getter/setter method for id
. The constructor calls the
initializer (named init
), which sets the value of id
to the default for type number, being
zero in this case. You could also explicitly pass a default by adding a default
key to the property,
should you so desire. We've also added a transform function that will take any value passed to the id
setter and apply parseInt() to it.
const ezobjects = require('ezobjects');
const fs = require('fs');
const moment = require('moment');
/**
* Load external MySQL configuration which uses the following JSON format:
* {
* "host" : "localhost",
* "user" : "ezobjects",
* "password" : "myPassword",
* "database" : "ezobjects"
* }
*/
const configMySQL = JSON.parse(fs.readFileSync('mysql-config.json'));
/**
* Create a connection object for the MySQL database using our MySQL
* module async/await wrapper. Currently, using the ezobjects MySQL
* database is the only option, but future versions may seek to expand
* the option to other databases, or at least allow the standard mysql
* module to work.
*/
const db = new ezobjects.MySQLConnection(configMySQL);
/**
* Configure a new EZ Object called DatabaseRecord with one 'id'
* property that contains extended MySQL configuration settings.
*/
const configDatabaseRecord = {
className: 'DatabaseRecord',
properties: [
{
name: 'id',
type: 'number',
mysqlType: 'int',
autoIncrement: true,
primary: true,
setTransform: x => parseInt(x)
}
]
};
/** Create the DatabaseRecord object */
ezobjects.createObject(configDatabaseRecord);
/**
* Configure a new EZ Object called Person that extends from the
* DatabaseRecord object and adds several additional properties and
* a MySQL index.
*/
const configPerson = {
tableName: 'people',
className: 'Person',
extends: DatabaseRecord,
extendsConfig: configDatabaseRecord,
properties: [
{
name: 'firstName',
type: 'string',
mysqlType: 'varchar',
length: 20
},
{
name: 'lastName',
type: 'string',
mysqlType: 'varchar',
length: 20
},
{
name: 'checkingBalance',
type: 'number',
mysqlType: 'double',
setTransform: x => parseFloat(x)
},
{
name: 'permissions',
type: 'Array',
mysqlType: 'text',
saveTransform: x => x.join(','),
loadTransform: x => x.split(',')
},
{
name: 'favoriteDay',
type: 'Date',
mysqlType: 'datetime',
saveTransform: x => moment(x).format('Y-MM-DD HH:mm:ss'),
loadTransform: x => new Date(x)
}
],
indexes: [
{ name: 'lastName', type: 'BTREE', columns: [ 'lastName' ] }
]
};
/** Create the Person object */
ezobjects.createObject(configPerson);
/** Create new person, initializing with object passed to constructor */
const person = new Person({
firstName: 'Rich',
lastName: 'Lowe',
checkingBalance: 4.32,
permissions: [1, 3, 5],
favoriteDay: new Date('01-01-2018')
});
/** Self-executing async wrapper so we can await results */
(async () => {
/** Create table if it doesn't already exist */
await ezobjects.createTable(db, configPerson);
/** Insert person into the database */
await person.insert(db);
/** Log person (should have automatically incremented ID now) */
console.log(person);
/** Close database connection */
db.close();
})();
Person {
_id: 1,
_firstName: 'Rich',
_lastName: 'Lowe',
_checkingBalance: 4.32,
_permissions: [ 1, 3, 5 ],
_favoriteDay: 2018-01-01T06:00:00.000Z }
In this snippet, we've created two classes, DatabaseRecord and Person. Person extends DatabaseRecord and is also associated with
a MySQL table called people
. Each property is given a JavaScript type
and a MySQL mysqlType
. Additional MySQL property
configuration options can be provided, which are outlined in more detail below. A BTREE index is also added on the lastName column
for faster searching. While not required, the moment library is used to help translate date formats between MySQL and JavaScript.
Since the Person class configuration provided a tableName
property, it will automatically have additional methods created that are
not present in a basic EZ Object. The additional methods are delete(db), insert(db), load(db, id), and update(db). These methods can
be used to delete the MySQL record corresponding to the object, insert the object properties as a new MySQL record, load a MySQL record
into the object properties, or update an existing MySQL record using the object properties. Transforms can be used to validate or
manipulate the property values when they are get or set in the object, or when they are saved or loaded from the database.
const a = new Person();
console.log(a);
Person {
_id: 0,
_firstName: '',
_lastName: '',
_checkingBalance: 0,
_permissions: [],
_favoriteDay: null }
const b = new Person({
id: 1,
firstName: 'Rich',
lastName: 'Lowe',
checkingBalance: 4.32,
permissions: [1, 3, 5],
favoriteDay: new Date('01-01-2018')
});
console.log(b);
Person {
_id: 1,
_firstName: 'Rich',
_lastName: 'Lowe',
_checkingBalance: 4.32,
_permissions: [ 1, 3, 5 ],
_favoriteDay: 2018-01-01T06:00:00.000Z }
const c = new Person();
c.id(2);
c.firstName('Bert');
c.lastName('Reynolds');
c.checkingBalance(91425518.32);
c.permissions([1, 4]);
c.favoriteDay(new Date('06-01-2017'));
console.log(c);
Person {
_id: 2,
_firstName: 'Bert',
_lastName: 'Reynolds',
_checkingBalance: 91425518.32,
_permissions: [ 1, 4 ],
_favoriteDay: 2017-06-01T05:00:00.000Z }
console.log(`ID: ${c.id()}`);
console.log(`First Name: ${c.firstName()}`);
console.log(`Last Name: ${c.lastName()}`);
console.log(`Checking Balance: $${c.checkingBalance()}`);
console.log(`Permissions: ${c.permissions().join(`, `)}`);
console.log(`Favorite Day: ${c.favoriteDay().toString()}`);
ID: 2
First Name: Bert
Last Name: Reynolds
Checking Balance: $91425518.32
Permissions: 1, 4
Favorite Day: Thu Jun 01 2017 00:00:00 GMT-0500 (CDT)
ezobjects.createTable(db, obj)
obj
, if it doesn't already existezobjects.createObject(obj)
obj
, with constructor, initializer, getters, setters, and also delete, insert, load, and update if tableName
is configuredezobjects.MySQLConnection(config)
FAQs
Easy Auto-Generated Strictly-Typed JavaScript Class Objects
The npm package ezobjects receives a total of 147 weekly downloads. As such, ezobjects popularity was classified as not popular.
We found that ezobjects demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.