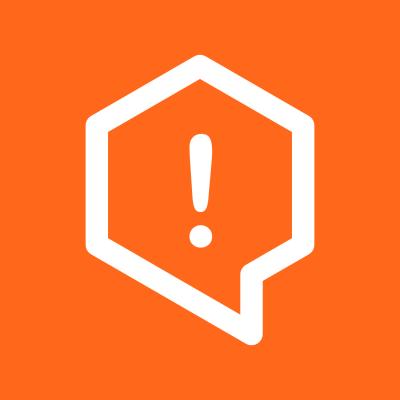
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
fast-graph
Advanced tools
A robust and fast package for handling graph opperations and algorithms
🚀 Cutting-edge TypeScript library designed to empower developers with a high-performance solution for efficient graph operations and algorithms. With a focus on speed and reliability, this library simplifies the implementation of graph-related tasks, offering a comprehensive set of features for seamless integration into any real world case.
yarn add fast-graph
npm install fast-graph
The Node
class represents a node in the graph, holding a unique identifier (id
) and an associated value of generic type (T
). The incomingNeighbors
property tracks incoming edges to the node.
import { Node } from "fast-graph";
// Example Usage:
const myNode = new Node<string>("uniqueId", "Node Value");
The Graph
class is the core component for graph operations. It allows you to create, manipulate, and perform various algorithms on graphs.
import { Node, Graph } from "fast-graph";
// Example Usage:
const myGraph = new Graph<string>();
// Adding Nodes
const nodeA = new Node<string>("A", "Node A");
const nodeB = new Node<string>("B", "Node B");
myGraph.addNode(nodeA);
myGraph.addNode(nodeB);
// Adding Edges
myGraph.addEdge(nodeA, nodeB);
// Removing Nodes
myGraph.removeNode(nodeA);
// Removing Edges
myGraph.removeEdge(nodeB, nodeA);
// Checking if the Graph is Empty
const isEmpty = myGraph.isEmpty();
// Getting Neighbors of a Node
const neighbors = myGraph.getNeighbors(nodeB);
// Kahn's Topological Sort
const topologicalOrder = myGraph.kahnTopologicalSort();
// Use BFS to visit all nodes in the graph
myGraph.bfs(node => {
console.log(`Visiting Node ${node.id} with value ${node.value}`);
return SearchAlgorithmNodeBehavior.continue;
});
// Use BFS to find a node
myGraph.bfs(node => {
console.log(`Visiting Node ${node.id} with value ${node.value}`);
return node.id === "id_your_looking_for"
? SearchAlgorithmNodeBehavior.break
: SearchAlgorithmNodeBehavior.continue;
});
// Use BFS to visit all nodes in the graph async
await myGraph.bfsAsync(async node => {
await yourExternalApiCall(node);
return SearchAlgorithmNodeBehavior.continue;
});
// Use DFS to visit all nodes in the graph
myGraph.dfs(node => {
console.log(`Visiting Node ${node.id} with value ${node.value}`);
return SearchAlgorithmNodeBehavior.continue;
});
// Use DFS to find a node
myGraph.dfs(node => {
console.log(`Visiting Node ${node.id} with value ${node.value}`);
return node.id === "id_your_looking_for"
? SearchAlgorithmNodeBehavior.break
: SearchAlgorithmNodeBehavior.continue;
});
// Use DFS to visit all nodes in the graph async
await myGraph.dfsAsync(async node => {
await yourExternalApiCall(node);
return SearchAlgorithmNodeBehavior.continue;
});
// Use Dijkstra's algorithm to discover shortest path from a node to all other nodes.
const dijkstraResult: NodeDistance = graph.dijkstra(nodeA);
Note: Ensure that you handle errors appropriately, as the library throws an error if attempting operations on non-existing nodes or in the presence of cycles.
Each algorithm has its strengths and is well-suited for specific types of problems. The choice depends on the nature of the problem you're solving and the characteristics of your data.
Contributions are welcome! Please submit a pull request with any improvements or bug fixes. Make sure to add tests for any new features and bug fixes, and ensure that the existing tests pass.
This project is licensed under the MIT License.
If you need help or have questions, feel free to open an issue in the GitHub repository.
FAQs
A fast implementation of graph data structure
The npm package fast-graph receives a total of 9 weekly downloads. As such, fast-graph popularity was classified as not popular.
We found that fast-graph demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.