What is fastq?
The fastq npm package is a fast, in-memory queue with worker functions. It is useful for managing tasks and processing them concurrently with a controlled level of parallelism. It allows you to define tasks, add them to the queue, and process them with a defined concurrency level.
What are fastq's main functionalities?
Task Queueing
This feature allows you to create a queue with a worker function that processes tasks. The second argument to `fastq` is the concurrency level, which in this case is set to 2, meaning that up to two tasks can be processed in parallel.
const fastq = require('fastq');
const worker = (task, cb) => {
console.log(`Processing task: ${task}`);
cb();
};
const queue = fastq(worker, 2);
queue.push('task1', (err) => console.log('Finished processing task1'));
queue.push('task2', (err) => console.log('Finished processing task2'));
Pause and Resume
This feature allows you to pause the processing of tasks in the queue and resume it later. This can be useful for throttling or when the system needs to perform other high-priority operations.
const fastq = require('fastq');
const worker = (task, cb) => {
setTimeout(() => {
console.log(`Processed: ${task}`);
cb();
}, 1000);
};
const queue = fastq(worker, 1);
queue.push('task1');
queue.push('task2');
queue.pause();
// ... some time later
queue.resume();
Drain Event
The 'drain' event is emitted when the last item from the queue has been assigned to a worker. It can be used to know when all tasks have been processed and the queue is empty.
const fastq = require('fastq');
const worker = (task, cb) => {
console.log(`Processing task: ${task}`);
cb();
};
const queue = fastq(worker, 2);
queue.drain = () => {
console.log('All tasks have been processed');
};
queue.push('task1');
queue.push('task2');
Other packages similar to fastq
async
The 'async' package provides a collection of utilities for working with asynchronous JavaScript. It includes a queue function that can be used to control concurrency similar to fastq. However, async offers a broader set of features for handling asynchronous operations beyond just queueing.
bee-queue
Bee-queue is a simple, fast, robust job/task queue for Node.js, backed by Redis. It is designed to be robust by handling failures and retries. It is more feature-rich for distributed systems compared to fastq, which is more focused on in-memory queueing.
bull
Bull is a Redis-based queue package for handling distributed jobs and messages in Node.js. It offers a rich set of features for job processing such as prioritization, scheduling, and repeatable jobs. Bull is more suitable for complex job processing scenarios compared to fastq's simpler in-memory queueing.
fastq 
Fast, in memory work queue. fastq
is API compatible with
async.queue
Benchmarks (1 million tasks):
- setImmediate: 1359ms
- fastq: 1492ms
- async.queue: 4039ms
- neoAsync.queue: 4476ms
Obtained on node 4.2.2, on a MacBook Pro 2014 (i7, 16GB of RAM).
If you need zero-overhead series function call, check out
fastseries. For zero-overhead parallel
function call, check out fastparallel.
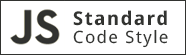
Install
npm i fastq --save
Usage
'use strict'
var queue = require('fastq')(worker, 1)
queue.push(42, function (err, result) {
if (err) { throw err }
console.log('the result is', result)
})
function worker (arg, cb) {
cb(null, 42 * 2)
}
Setting this
'use strict'
var that = { hello: 'world' }
var queue = require('fastq')(that, worker, 1)
queue.push(42, function (err, result) {
if (err) { throw err }
console.log(this)
console.log('the result is', result)
})
function worker (arg, cb) {
console.log(this)
cb(null, 42 * 2)
}
API
fastqueue([that], worker, concurrency)
Creates a new queue.
Arguments:
that
, optional context of the worker
function.worker
, worker function, it would be called with that
as this
,
if that is specified.concurrency
, number of concurrent tasks that could be executed in
parallel.
queue.push(task, done)
Add a task at the end of the queue. done(err, result)
will be called
when the task was processed.
queue.unshift(task, done)
Add a task at the beginning of the queue. done(err, result)
will be called
when the task was processed.
queue.pause()
Pause the processing of tasks. Currently worked tasks are not
stopped.
queue.resume()
Resume the processing of tasks.
queue.idle()
Returns false
if there are tasks being processed or waiting to be processed.
true
otherwise.
queue.length()
Returns the number of tasks waiting to be processed (in the queue).
queue.kill()
Removes all tasks waiting to be processed, and reset drain
to an empty
function.
queue.killAndDrain()
Same than kill
but the drain
function will be called before reset to empty.
queue.concurrency
Property that returns the number of concurrent tasks that could be executed in
parallel. It can be altered at runtime.
queue.drain
Function that will be called when the last
item from the queue has been processed by a worker.
It can be altered at runtime.
queue.empty
Function that will be called when the last
item from the queue has been assigned to a worker.
It can be altered at runtime.
queue.saturated
Function that will be called when the queue hits the concurrency
limit.
It can be altered at runtime.
License
ISC