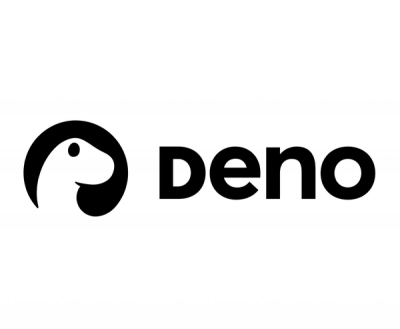
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Lightweight javascript library to mock network request for testing purposes
This project is still an idea and probably needs further improvements. Suggests and PR are welcome.
npm install --save-dev faussaire
Faussaire is library aiming to mock an API in tests. As said Robert C. Martin in the Ruby Midwest 2011 Conf, tests should be completely independant from your webservices, your database or whatever kind of IO device / Network implementation. Following this idea, one should be able to use a fake API with a similar behavior of any server.
Faussaire implements a simple interface allowing you to create route with URLs and method, and to put a controller to
return a response object. Using the fetch()
method you can easily make calls and simulate a response.
You can register a route using faussaire.Route.
import faussaire from 'faussaire';
faussaire
.Route({
template: "http://foo.com",
methods: ["GET"],
controller: {
run: (params, options) => {
return faussaire.Response({
data: {
foo: params.foo,
bar: params.bar
},
status: 200,
statusText: "OK"
})
}
}
});
const response = faussaire.fetch("http://foo.com", "GET", {foo: "bar", bar: "qux"});
You can as well pre-authenticate the user sending a request by defining an authenticate(params, options)
in the
controller. It should return a token in case of success and it will be stored in the options object as options.token
.
If the authentication fail, there wont be any token object in options.
import faussaire from 'faussaire';
faussaire
.Route({
template: "http://foo.com/ressouce",
methods: ["GET"],
controller: {
authenticate: function(params, options){
if(params.apikey){
return {
apikey: params.apikey
at: Date.now()
expire: //...
}
}
},
run: (params, options) => {
if(options.token){
return faussaire.Response({
status: 200,
statusText: "OK"
})
}
return faussaire.Response({
status: 403,
statusText: "Wrong credentials"
})
}
}
});
const response = faussaire.fetch("http://foo.com", "GET", {foo: "bar", bar: "qux"});
The equivalent of a standard fetch.
Adds a route to Faussaire.
Return a basic HTTP response with :
You might want to make your own Fetch interface using Faussaire to automate switching between network fetching and local fetching. This is how it can be implemented :
import fetch from 'fetch';
import faussaire from 'faussaire';
const fetch = (url, method, params) => {
if(process.env.NODE_ENV === "test"){
return faussaire(url, method, params);
}
return fetch(url, method, params);
};
export default fetch;
FAQs
Lightweight library to mock API for testing purpose
The npm package faussaire receives a total of 3 weekly downloads. As such, faussaire popularity was classified as not popular.
We found that faussaire demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.