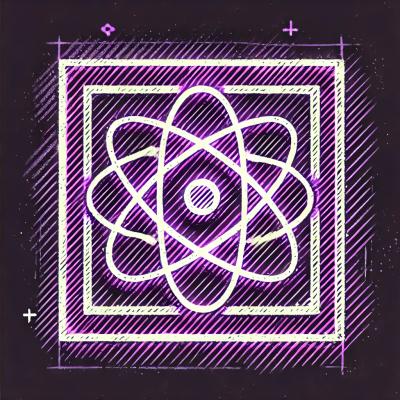
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
The fetch-h2 npm package is a modern HTTP/2 client for Node.js, providing a fetch API compatible interface. It allows you to make HTTP/2 requests with ease, leveraging the benefits of HTTP/2 such as multiplexing, header compression, and server push.
Basic HTTP/2 GET Request
This feature allows you to make a basic HTTP/2 GET request to a specified URL and log the response body.
const { fetch } = require('fetch-h2');
(async () => {
const response = await fetch('https://example.com');
const body = await response.text();
console.log(body);
})();
HTTP/2 POST Request with JSON Body
This feature allows you to make an HTTP/2 POST request with a JSON body to a specified URL and log the JSON response.
const { fetch } = require('fetch-h2');
(async () => {
const response = await fetch('https://example.com/api', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ key: 'value' })
});
const json = await response.json();
console.log(json);
})();
Handling HTTP/2 Server Push
This feature demonstrates how to handle server push in HTTP/2, where the server can send additional resources to the client without the client explicitly requesting them.
const { fetch } = require('fetch-h2');
(async () => {
const response = await fetch('https://example.com');
response.on('push', (pushStream) => {
pushStream.on('data', (chunk) => {
console.log('Pushed data:', chunk.toString());
});
});
})();
The http2 package is a core Node.js module that provides an implementation of the HTTP/2 protocol. It offers lower-level control over HTTP/2 connections compared to fetch-h2, which provides a higher-level, fetch-like API.
Axios is a popular HTTP client for Node.js and the browser. While it primarily supports HTTP/1.1, it can be used with HTTP/2 by configuring it with an HTTP/2-compatible adapter. Axios provides a promise-based API similar to fetch-h2 but is more widely used and has a larger community.
Node-fetch is a lightweight module that brings the window.fetch API to Node.js. It supports HTTP/1.1 and can be extended to support HTTP/2 with additional configuration. Node-fetch is similar to fetch-h2 in providing a fetch-like API but does not natively support HTTP/2.
HTTP/2 Fetch API implementation for Node.js (using Node.js' built-in http2
module). This module is intended to be solely for HTTP/2, handling HTTP/2 sessions transparently. For an HTTP/1(.1)-only alternative, you can use node-fetch.
The module tries to adhere to the Fetch API very closely, but extends it slightly to fit better into Node.js (e.g. using streams).
Regardless of whether you're actually interested in the Fetch API per se or not, as long as you want to handle HTTP/2 client requests in Node.js, this module is a lot easier and more natural to use than the native built-in http2
module which is low-level in comparison.
NOTE; HTTP/2 support was introduced in Node.js 8.4, and requires node
to be started with a flag --expose-http2
. This module won't work without it.
DISCLAIMER: This is an early project, and the Node.js implementation is early too. Don't expect it to be very stable yet. As an example, connecting to a TLS (HTTPS) server doesn't work in Node.js yet.
This README uses the ES6/TypeScript import
syntax, mainly because fetch-h2
is written in TypeScript (and also because ES6 modules will eventually arrive in Node.js). If you use pure JavaScript in Node.js today, you don't have modules support, just require
instead, e.g:
const { fetch } = require( 'fetch-h2' );
fetch-h2
exports more than just fetch()
, namely all necessary classes and functions for taking advantage of the Fetch API (and more).
import {
context,
fetch,
disconnect,
disconnectAll,
Body,
JsonBody,
Headers,
Request,
Response,
AbortError,
PushMessage,
} from 'fetch-h2'
Apart from the obvious fetch
, the functions context
, disconnect
and disconnectAll
are described below, and the classes Body
, Headers
, Request
and Response
are part of the Fetch API. JsonBody
is an extension over Body
, described below. AbortError
is the error thrown in case of an abort signal (this is also the error thrown in case of a timeout, which in fetch-h2
is internally implemented as an abort signal). The PushMessage
is an interface for onPush
callbacks, mentioned below.
Import fetch
from fetch-h2
and use it like you would use fetch
in the browser.
import { fetch } from 'fetch-h2'
const response = await fetch( url );
const responseText = await response.text( );
With HTTP/2, all requests to the same origin (domain name and port) share a single session (socket). In browsers, it is eventually disconnected, maybe. It's up to the implementation to handle disconnections. In fetch-h2
, you can disconnect it manually, which is great e.g. when using fetch-h2
in unit tests.
Disconnect the session for a certain url (the session for the origin will be disconnected) using disconnect
, and disconnect all sessions with disconnectAll
. Read more on contexts below to understand what "all" really means...
import { disconnect, disconnectAll } from 'fetch-h2'
await disconnect( "http://mysite.com/foo" ); // "/foo" is ignored, but allowed
// or
await disconnectAll( );
fetch-h2
has a few limitations, some purely technical, some more fundamental or perhaps philosophical, which you will find in the Fetch API but missing here.
fetch-h2
believes.Body
class/mixin doesn't support the formData()
function. This can be added if someone really wants it - PR's are welcome.Body
class/mixin doesn't support the blob()
function. This type of buffer doesn't exist in Node.js, use arrayBuffer()
instead.HEAD
and GET
requests. If e.g. a POST
request gets a 3xx-code response and redirect
is set to follow
, the result is an error. Redirections for non-idempotent requests are only allowed if redirect
is error
or manual
(which is the default). Note that the default for redirect
is different among browsers (and even versions of them). The specs are non-obvious but seems to suggest manual
initially, followed by redirect
. It's a good idea to explicitly set mode
and not depend on any default.credentials
option is currently not implemented since fetch-h2
has no cookie jar ('yet').cache
option is unused, as fetch-h2
has no built-in cache.referrer
and referrerPolicy
are unused, as fetch-h2
operates outside the concept of "web pages".integrity
option is actually implemented but no validation is performed if the result body is read through the Node.js ReadableStream
(using response.readable( )
). The body is validated if arrayBuffer( )
, blob( )
, json( )
or text( )
is used to read the body, in which case these functions will return a rejected promise.These are features in fetch-h2
, that don't exist in the Fetch API. Some things are just very useful in a Node.js environment (like streams), some are due to the lack of a browser with all its responsibilities.
redirect
is set to manual
, the response is supposed to be empty and useless, with no status code or anything (according to spec). In fetch-h2
, it's a normal useful Response
object.body
that can be sent in a Request, and that is available on the Response, can be a Node.js ReadableStream
. You can thereby stream data with a request, and stream the response body.body
that can be sent in a Request can be a Body
object. There's an extended JsonBody
class (extending Body
) which represents an object (that will be JSON stringified). This is useful to e.g. POST
an object. The fetch
implementation will detect this JsonBody
and apply the right content-type
if it isn't already set.fetch()
has an extra option, timeout
which can be used (although not together with signal
), which is a timeout in milliseconds before the request should be aborted and the returned promise thereby rejected (with an AbortError
).fetch()
has an extra option, onPush
which is an optional callback that will be called when pushes are performed for a certain fetch operation. This callback should take a PushMessage
argument, which will contain {url, method, statusCode, headers}
. fetch-h2
performs absolutely no push magic.Request.clone()
member function has an optional url
argument.HTTP/2 expects a client implementation to not create new sockets (sessions) for every request, but instead re-use them - create new requests in the same session. This is also totally transparent in the Fetch API. It might be useful to control this, and create new "browser contexts", each with their own set of HTTP/2-sessions-per-origin. This is done through the context
function.
This function returns an object which looks like the global fetch-h2
API, i.e. it will have the functions fetch
, disconnect
and disconnectAll
.
import { context } from 'fetch-h2'
const ctx = context( /* options */ );
ctx.fetch( url | Request, init?: InitOpts );
ctx.disconnect( url );
ctx.disconnectAll( );
The global fetch
, disconnect
and disconnectAll
functions are default-created from a context internally. They will therefore not interfere, and disconnect
/disconnectAll
only applies to its own context, be it a context created by you, or the default one from fetch-h2
.
If you want one specific context in a file, why not destructure the return in one go?
import { context } from 'fetch-h2'
const { fetch, disconnect, disconnectAll } = context( );
When an error is thrown (or a promise is rejected), fetch-h2
will always provide proper error objects, i.e. instances of Error
.
If servers are redirecting a fetch operation in a way that causes a circular redirection, e.g. servers redirect A -> B -> C -> D -> B
, fetch-h2
will detect this and fail the operation with an error. The error object will have a property urls
which is an array of the urls that caused the loop (in this example it would be [ B, C, D ]
, as D
would redirect to the head of this list again).
Using await
and the Body.json()
function we can easily get a JSON object from a response.
import { fetch } from 'fetch-h2'
const jsonData = await ( await fetch( url ) ).json( );
Use the JsonBody
subclass of Body
to simplify the creation of an application/json
body. This is an extension in fetch-h2
, not existing in the Fetch API.
import { fetch, JsonBody } from 'fetch-h2'
const myData = { foo: 'bar' };
const body = new JsonBody( myData );
const method = 'POST';
const response = await fetch( url, { method, body } );
FAQs
HTTP/1+2 Fetch API client for Node.js
The npm package fetch-h2 receives a total of 121,826 weekly downloads. As such, fetch-h2 popularity was classified as popular.
We found that fetch-h2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.