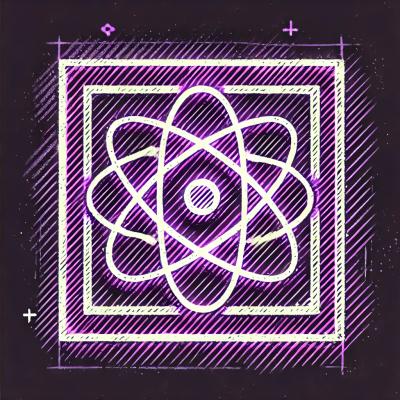
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Fibre.js is a small library based on FindAndReplaceDOMText
by James Padolsey.
The method collections of Fibre.js help search for the regular expression matches in a given context (a DOM node) and replace/wrap each one of them with a new text run or a new DOM node.
Chaining & string-like syntax supported.
npm i fibre.js --save
Use the script
element,
<script src="vendor/fibre.js/fibre.js"></script>
AMD,
require( './node_modules/fibre.js/src/fibre', function( Fibre ) {
var fibre = Fibre( document.body )
…
})
CommonJS (NPM),
var Fibre = require( 'fibre.js' )
var fibre = Fibre( document.body )
…
sudo npm i
gulp test
Fibre.js works on all modern browsers with no legacy support for older IE.
Fibre.js is released under MIT License.
The syntax is as simple as jQuery!
Fibre
needs no new
operator to create an instance. Assign one DOM node to the first parametre context
to initialise.
var fibre = Fibre( context, noPreset )
fibre
.replace( … )
.wrap( … )
noPreset
switchBy default, Fibre ignores elements like style
, script
, img
, etc and considers elements like div
, p
, h1
, etc the boundaries of text. Set the second parametre noPreset
to true
to turn off such configuration.
// Regular DOM node
Fibre( document.body )
// Document node
Fibre( document )
// jQuery object
Fibre( $( '.container article' )[0] )
// CSS selector (will only affect the first matched node)
Fibre( '.container article' )
The method wraps an assigned node round the matched text in the given context.
fibre.wrap( regexp|substr, strElemName|node )
regexp
substr
strElemName
node
<body>
<p>Apple-eaters eat thousands of apples a day.</p>
</body>
Fibre( document.body ).wrap( /\bapple(s)?\b/gi, 'em' )
Which results in:
<body>
<p><em>Apple</em>-eaters eat thousands of <em>apples</em> a day.</p>
</body>
Works with matches spread in different nodes,
<body>
<p><span>App</span>le-eaters eat thousands of apple<span class="pl">s</span> a day.</p>
</body>
Results in:
<body>
<p><span><em>App</em></span><em>le</em>-eaters eat thousands of <em>apple</em><span class="pl"><em>s</em></span> a day.</p>
</body>
The method replaces the matched text in the given context with a new string.
fibre.replace( regexp|substr, newSubStr|function )
regexp
substr
newSubStr
function
<article>
<p>Th<span>is</span> paragraph is to be later replaced.</p>
</article>
var fibre = Fibre( 'article' )
fibre
.replace( /(\w+)/g, '*$1' )
.replace( /\*this/ig, function( portion, match ) {
var idx = portion.index
return idx + match[ idx ].toUpperCase()
})
Will result in:
<article>
<p>*TH<span>IS</span> *paragraph *is *to *be *later *replaced.</p>
</article>
The method sets the portion mode of the instance.
fibre.setMode( portionMode )
portionMode
The method adds text boundary(ies) to avoids their cross-node matching and replacing.
fibre.addBoundary( selector )
selector
<div id="test">
<p>Something</p>
<p>Some</p><blockquote>Thing</blockquote>
<a>Something</a>
<a>Some</a><a class="block">Thing</a>
</div>
Fibre( document.getElementById( 'test' ))
.wrap( /something/gi, 'span' )
.addBoundary( 'a.block' )
.wrap( /something/gi, 'b' )
Will result in:
<div id="test">
<p><span><b>Something</b></span></p>
<p>Some</p><blockquote>Thing</blockquote>
<a><span><b>Something</b></span></a>
<a><span>Some</span></a><a class="block"><span>Thing</span></a>
</div>
The methods removes all custom text boundaries of the instance.
fibre.removeBoundary()
Note: The preset configurations will not be removed by the use of the method.
The method avoids the nodes matching the given selector which will later not apply the wrap/replace method.
Note: An older name of the method filterOut()
is deprecated since v0.2.0. Will be removed in the next major update.
fibre.avoid( selector )
selector
<div id="test">
<p>This <span class="be">is</span> a simple test.</p>
</div>
<script>
var test = 'This is a simple test.'
</script>
Fibre( document.getElementById( 'test' ))
.wrap( /is/gi, 'span' )
.avoid( '.be' )
.replace( /is/gi, 'IZZ' )
Will result in:
<div id="test">
<p>Th<b>IZZ</b> <span class="be"><b>is</b></span> a simple test.</p>
</div>
<script>
var test = 'ThIZZ IZZ a simple test.'
</script>
The method ends the avoiding and pops back to previous state of the context.
fibre.endAvoid( all )
all
Note: The preset configurations will not be reset by the use of the method.
The method filters the nodes matching the given selector which will later apply the wrap/replace method.
fibre.filter( selector )
selector
<div id="test">
<p>This <span class="be">is</span> a simple test.</p>
</div>
<script>
var test = 'This is a simple test.'
</script>
Fibre( document.getElementById( 'test' ))
.wrap( /is/gi, 'b' )
.filter( '.be b' )
.wrap( /is/gi, 'em' )
Will result in:
<div id="test">
<p>Th<b>is</b> <span class="be"><b><em>is</em></b></span> a simple test.</p>
</div>
<script>
var test = 'This is a simple test.'
</script>
Note: The matched text inside script
element isn't altered for Fibre avoids script
, style
, etc by default.
The method ends the filtering and pops back to previous state of the context.
fibre.endFilter( all )
all
Note: The preset configurations will not be reset by the use of the method.
The method reverts the finder by a given level.
fibre.revert( [level] )
level
'all'
indicating the finder level to revert. The default value is 1
.
var fibre = Fibre( document.getElementById( 'test' ))
.replace( /\bis\b/gi, 'isn\'t' )
.wrap( /\bwill\b/gi, 'span' )
// Later,
fibre.revert( 'all' )
The last line of the script above will revert the context (document.getElementById( 'test' )
) back to the state before any replace or wrap method has executed.
The method compares and returns whether a given node matches the given CSS selectors.
Fibre.matches( node, selector, bypassNodeType39 )
node
selector
bypassNodeType39
<!doctype html>
<html lang="en">
<head>
</head>
<body class="post">
</body>
</html>
Fibre.matches( document.body, 'body.index, body.post' ) // returns true
Fibre.matches( document.documentElement, '[lang="es"]' ) // returns false
Fibre.matches( document, 'style' ) // returns false
Fibre.matches( document, 'style', true ) // returns true
FAQs
String-like DOM text manipulator
The npm package fibre.js receives a total of 10 weekly downloads. As such, fibre.js popularity was classified as not popular.
We found that fibre.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.