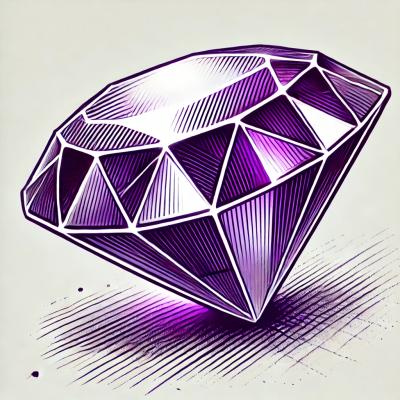
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
file-stream-rotator
Advanced tools
The file-stream-rotator npm package is used for rotating log files based on a specified time interval or file size. It is commonly used in logging systems to manage log file sizes and ensure that logs are archived and rotated properly.
Time-based rotation
This feature allows you to rotate log files based on a specified time interval, such as daily. The log files will be named with the current date.
const FileStreamRotator = require('file-stream-rotator');
const fs = require('fs');
const logStream = FileStreamRotator.getStream({
filename: 'logs/logfile-%DATE%.log',
frequency: 'daily',
verbose: false,
date_format: 'YYYYMMDD'
});
logStream.write('This is a log message.\n');
Size-based rotation
This feature allows you to rotate log files based on a specified file size. Once the log file reaches the specified size, it will be rotated, and a new log file will be created.
const FileStreamRotator = require('file-stream-rotator');
const fs = require('fs');
const logStream = FileStreamRotator.getStream({
filename: 'logs/logfile-%DATE%.log',
size: '10M',
max_logs: '10',
audit_file: 'logs/audit.json'
});
logStream.write('This is a log message.\n');
Custom rotation
This feature allows you to define custom rotation strategies, combining both time and size-based rotation. You can specify the frequency, size, and other parameters to control the rotation behavior.
const FileStreamRotator = require('file-stream-rotator');
const fs = require('fs');
const logStream = FileStreamRotator.getStream({
filename: 'logs/logfile-%DATE%.log',
frequency: 'custom',
verbose: false,
date_format: 'YYYYMMDD',
size: '5M',
max_logs: '5',
audit_file: 'logs/audit.json',
end_stream: true
});
logStream.write('This is a log message.\n');
The winston-daily-rotate-file package is a transport for the winston logging library that allows for daily log file rotation. It provides similar functionality to file-stream-rotator but is specifically designed to work with the winston logging library.
The rotating-file-stream package provides a similar functionality of rotating log files based on time or size. It offers more flexibility in terms of configuration and supports various rotation strategies.
The logrotate-stream package is a simple stream that rotates log files based on size. It is less feature-rich compared to file-stream-rotator but can be a lightweight alternative for basic log rotation needs.
NodeJS file stream rotator
To provide an automated rotation of Express/Connect logs or anything else that writes to a file on a regular basis that needs to be rotated based on date, a size limit or combination and remove old log files based on count or elapsed days.
npm install file-stream-rotator
{ flags: 'a' }
. // Default date added at the end of the file
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test.log", frequency:"daily", verbose: false});
// Default date added using file pattern
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test-%DATE%.log", frequency:"daily", verbose: false});
// Custom date added using file pattern using moment.js formats
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test-%DATE%.log", frequency:"daily", verbose: false, date_format: "YYYY-MM-DD"});
// Rotate when the date format as calculated by momentjs is different (e.g monthly)
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test-%DATE%.log", frequency:"custom", verbose: false, date_format: "YYYY-MM"});
// Rotate when the date format as calculated by momentjs is different (e.g weekly)
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test-%DATE%.log", frequency:"custom", verbose: false, date_format: "YYYY-ww"});
// Rotate when the date format as calculated by momentjs is different (e.g AM/PM)
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test-%DATE%.log", frequency:"custom", verbose: false, date_format: "YYYY-MM-DD-A"});
// Rotate on given minutes using the 'm' option i.e. 5m or 30m
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test.log", frequency:"5m", verbose: false});
// Rotate on the hour or any specified number of hours
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test.log", frequency:"1h", verbose: false});
// Rotate on the hour or any specified number of hours and keep 10 files
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test.log", frequency:"1h", verbose: false, max_logs: 10});
// Rotate on the hour or any specified number of hours and keep 10 days
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test.log", frequency:"1h", verbose: false, max_logs: "10d"});
// Rotate on the hour or any specified number of hours and keep 10 days and store the audit file in /tmp/log-audit.json
var rotatingLogStream = require('file-stream-rotator').getStream({filename:"/tmp/test.log", frequency:"1h", verbose: false, max_logs: "10d", audit_file: "/tmp/log-audit.json"});
//.....
// Use new stream in express
app.use(express.logger({stream: rotatingLogStream, format: "default"}));
//.....
You can listen to the open, close, error and finish events generated by the open stream. You can also listen for custom events:
You can also limit the size of each file by adding the size option using "k", "m" and "g" to specify the size of the file in kiloybytes, megabytes or gigabytes. When it rotates a file based on size, it will add a number to the end and increment it for every time the file rotates in the given period as shown below.
3078 7 Mar 13:09:58 2017 testlog-2017-03-07.13.09.log.20
2052 7 Mar 13:10:00 2017 testlog-2017-03-07.13.09.log.21
3078 7 Mar 13:10:05 2017 testlog-2017-03-07.13.10.log.1
3078 7 Mar 13:10:08 2017 testlog-2017-03-07.13.10.log.2
3078 7 Mar 13:10:11 2017 testlog-2017-03-07.13.10.log.3
3078 7 Mar 13:10:14 2017 testlog-2017-03-07.13.10.log.4
The example below will rotate files daily but each file will be limited to 5MB.
// Rotate every day or every 5 megabytes, whatever comes first.
var rotatingLogStream = require('file-stream-rotator').getStream(
{
filename:"/tmp/test-%DATE%.log",
frequency:"custom",
verbose: false,
date_format: "YYYY-MM-DD",
size: "5M" // its letter denominating the size is case insensitive
}
);
rotatingLogStream.on('rotate',function(oldFile,newFile){
// do something with old file like compression or delete older than X days.
})
The npm module for this library will be maintained by:
Thanks to the contributors below for raising PRs and everyone else that has raised issues to make the module better.
file-stream-rotator is licensed under the MIT license.
FAQs
Automated stream rotation useful for log files
The npm package file-stream-rotator receives a total of 294,563 weekly downloads. As such, file-stream-rotator popularity was classified as popular.
We found that file-stream-rotator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.