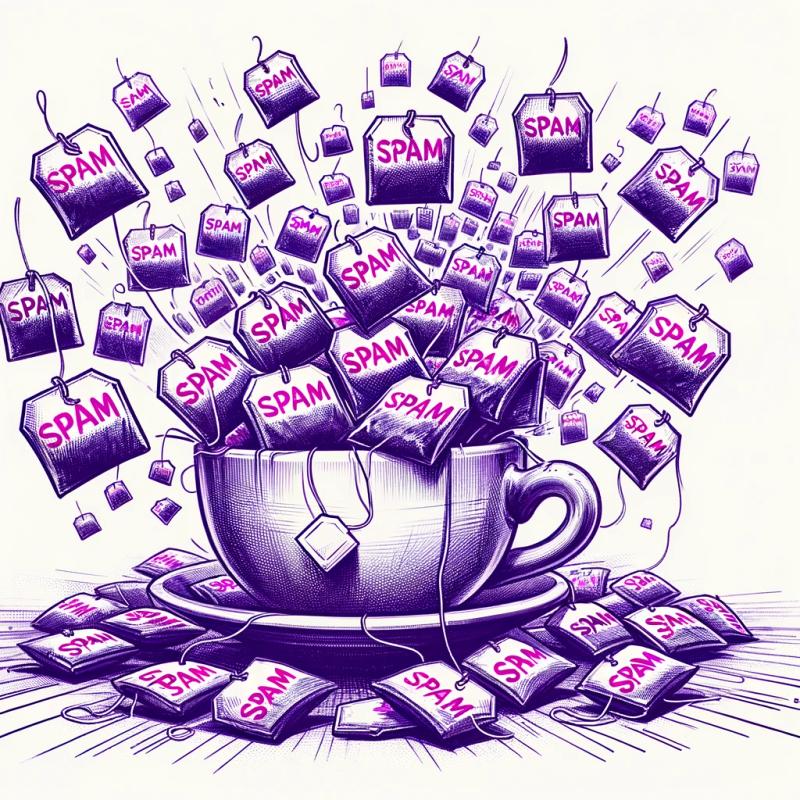
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
file-url
Advanced tools
Readme
Convert a file path to a file url:
unicorn.jpg
→file:///Users/sindresorhus/unicorn.jpg
$ npm install file-url
import fileUrl from 'file-url';
fileUrl('unicorn.jpg');
//=> 'file:///Users/sindresorhus/dev/file-url/unicorn.jpg'
fileUrl('/Users/pony/pics/unicorn.jpg');
//=> 'file:///Users/pony/pics/unicorn.jpg'
fileUrl('unicorn.jpg', {resolve: false});
//=> 'file:///unicorn.jpg'
Returns the filePath
converted to a file URL.
Type: string
File path to convert.
Type: object
Type: boolean
Default: true
Passing false
will make it not call path.resolve()
on the path.
FAQs
Convert a file path to a file url: `unicorn.jpg` → `file:///Users/sindresorhus/unicorn.jpg`
The npm package file-url receives a total of 368,871 weekly downloads. As such, file-url popularity was classified as popular.
We found that file-url demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.